File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
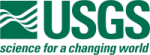 |
Isis 3 Programmer Reference
|
7 #include "CameraFocalPlaneMap.h"
26 Init(parent, naifIkCode);
99 if (naifIkCode != 0) {
100 QString xkey =
"INS" +
toString(naifIkCode) +
"_TRANSX";
101 QString ykey =
"INS" +
toString(naifIkCode) +
"_TRANSY";
102 QString ixkey =
"INS" +
toString(naifIkCode) +
"_ITRANSS";
103 QString iykey =
"INS" +
toString(naifIkCode) +
"_ITRANSL";
104 for (
int i = 0; i < 3; ++i) {
112 QString xkey =
"IDEAL_TRANSX";
113 QString ykey =
"IDEAL_TRANSY";
114 QString ixkey =
"IDEAL_TRANSS";
115 QString iykey =
"IDEAL_TRANSL";
116 for (
int i = 0; i < 3; ++i) {
202 double magCoef1 = fabs(
p_transx[1]);
203 double magCoef2 = fabs(
p_transx[2]);
205 if (magCoef1 > magCoef2) {
224 double magCoef1 = fabs(
p_transy[1]);
225 double magCoef2 = fabs(
p_transy[2]);
227 if (magCoef1 > magCoef2) {
325 const double lineOffset) {
353 for (
int i=0; i<3; ++i) {
365 for (
int i=0; i<3; ++i) {
377 for (
int i=0; i<3; ++i) {
389 for (
int i=0; i<3; ++i) {
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
void SetTransS(const QVector< double > transS)
Set the affine coefficients for converting destorted (x,y) to a detector Sample.
double DetectorSampleOffset() const
double FocalPlaneY() const
double p_focalPlaneY
y value of focal plane coordinate
double p_detectorLineOffset
Offset of the detector origin line from the average.
double p_detectorLineOrigin
The origin line of the detector.
void SetTransX(const QVector< double > transX)
Set the affine coefficients for converting detector (sample,line) to a distorted X.
void Init(Camera *parent, const int naifIkCode)
Initialize the focal plane map to its default state.
CameraFocalPlaneMap(Camera *parent, const int naifIkCode)
Construct mapping between detectors and focal plane x/y.
double SignMostSigX()
Return the sign of the p_transx coefficient with the greatest magnitude.
Camera * p_camera
Camera of the image.
double DetectorLine() const
void SetDetectorOffset(const double sampleOffset, const double lineOffset)
Set the detector offset.
void ComputeCentered()
Convenience method to center detector origin (use when inheriting)
double p_itransl[3]
The y transition from distorted to detector.
void ComputeUncentered()
Convenience method to center detector origin (use when inheriting)
double p_detectorLine
line value of the detector
double DetectorSampleOrigin() const
double p_centeredDetectorSample
Detector sample position.
double DetectorSample() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
const double * TransX() const
double CenteredDetectorLine() const
int FocalPlaneXDependency()
Return the focal plane x dependency variable.
double p_detectorSample
sample value of the detector
double DetectorLineOffset() const
double p_detectorSampleOffset
offset of the detector origin sample from the average
double DetectorLineOrigin() const
const double * TransL() const
virtual bool SetFocalPlane(const double dx, const double dy)
Compute detector position (sample,line) from focal plane coordinates.
@ Line
The x value of the focal plane maps to a line.
double p_centeredDetectorLine
Detector line position.
double p_transx[3]
The x transition from detector to distorted.
const double * TransY() const
void SetTransY(const QVector< double > transY)
Set the affine coefficients for converting detector (sample,line) to a distorted Y.
virtual ~CameraFocalPlaneMap()
Destructor.
std::vector< double > Coefficients(int var)
Return the affine coeffients for the entered variable (1 or 2).
void SetFocalPlaneMap(CameraFocalPlaneMap *map)
Sets the Focal Plane Map.
double CenteredDetectorSample() const
double p_detectorSampleOrigin
The origin sample of the detector.
const double * TransS() const
@ Sample
The x value of the focal plane maps to a sample.
double p_transy[3]
The y transition from detector to distorted.
double FocalPlaneX() const
virtual bool SetDetector(const double sample, const double line)
Compute distorted focal plane coordinate from detector position (sampel,line)
void SetTransL(const QVector< double > transL)
Set the affine coefficients for converting destorted (x,y) to a detector Line.
double SignMostSigY()
Return the sign of the p_transy coefficient with the greatest magnitude.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
double p_focalPlaneX
x value of focal plane coordinate
This is free and unencumbered software released into the public domain.
std::vector< double > InverseCoefficients(int var)
Return the inverse affine coeffients for the entered variable (1 or 2).
double p_itranss[3]
The x transition from distorted to detector.