Loading [MathJax]/jax/output/NativeMML/config.js
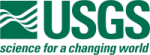 |
Isis 3 Programmer Reference
|
7 #include "Projection.h"
19 #include "Constants.h"
20 #include "Displacement.h"
22 #include "IException.h"
24 #include "Longitude.h"
25 #include "NaifStatus.h"
28 #include "PvlKeyword.h"
29 #include "RingPlaneProjection.h"
30 #include "SpecialPixel.h"
31 #include "TProjection.h"
32 #include "WorldMapper.h"
91 Projection::Projection(
Pvl &label) : m_mappingGrp(
"Mapping") {
134 if (str.toUpper() ==
"SKY")
m_sky =
true;
142 QString msg =
"Projection failed. Invalid label group [Mapping]";
164 if (
Name() != proj.
Name())
return false;
179 return !(*
this == proj);
189 m_projectionType = ptype;
199 return m_projectionType;
418 if (coord1 ==
Null || coord2 ==
Null) {
447 if (coord1 ==
Null || coord2 ==
Null) {
506 projectionX = worldX;
507 projectionY = worldY;
510 return SetCoordinate(projectionX, projectionY);
567 if (projectionX ==
Null) {
569 "Unable to convert to world x. The given x-value ["
570 +
IString(projectionX) +
"] is invalid.",
595 if (projectionY ==
Null) {
597 "Unable to convert to world y. The given y-value ["
598 +
IString(projectionY) +
"] is invalid.",
623 if (worldX ==
Null) {
625 "Unable to convert to projection x. The given x-value ["
626 +
IString(worldX) +
"] is invalid.",
651 if (worldY ==
Null) {
653 "Unable to convert to projection y. The given y-value ["
654 +
IString(worldY) +
"] is invalid.",
707 int iangle = (int)angle;
708 double mins = abs(angle - iangle) * 60.0;
709 int imins = (int)mins;
710 double secs = (mins - imins) * 60.0;
711 int isecs = (int)secs;
712 double frac = (secs - isecs) * 1000.0;
713 if (frac >= 1000.0) {
726 s << iangle <<
" " << setw(2) << setfill(
'0')
727 << imins <<
"m " << setw(2) << setfill(
'0') << isecs <<
"." <<
728 setprecision(3) << frac <<
"s";
729 return s.str().c_str();
742 double tangle = angle;
743 while (tangle < 0.0) tangle += 360.0;
744 while (tangle > 360.0) tangle -= 360.0;
746 int ihrs = (int)(hrs);
747 double mins = (hrs - ihrs) * 60.0;
748 int imins = (int)(mins);
749 double secs = (mins - imins) * 60.0;
750 int isecs = (int)(secs);
751 double msecs = (secs - isecs) * 1000.0;
752 int imsecs = (int)(msecs + 0.5);
753 if (imsecs >= 1000) {
766 s << setw(2) << setfill(
'0') << ihrs <<
"h " << setw(2) << setfill(
'0') <<
767 imins <<
"m " << setw(2) << setfill(
'0') << isecs <<
"." << imsecs <<
"s";
768 return s.str().c_str();
791 m_x = x * cos(rot) + y * sin(rot);
792 m_y = y * cos(rot) - x * sin(rot);
821 return m_x * cos(rot) -
m_y * sin(rot);
832 return m_y * cos(rot) +
m_x * sin(rot);
virtual double WorldX(const double projectionX) const =0
This pure virtual method will return a world X coordinate given a projection Y coordinate in meters.
bool m_sky
Indicates whether projection is sky or land.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
WorldMapper * m_mapper
This points to a mapper passed into the SetWorldMapper method.
const double PI
The mathematical constant PI.
A single keyword-value pair.
double Resolution() const
This method returns the resolution for mapping world coordinates into projection coordinates.
void SetUpperLeftCorner(const Displacement &x, const Displacement &y)
This method searches for extreme (min/max/discontinuity) coordinate values along the constBorder line...
double m_pixelResolution
Pixel resolution value from the PVL mapping group, in meters/pixel.
virtual double ProjectionX(const double worldX) const =0
This pure virtual method will return a projection X coordinate in meters given a world X coordinate.
double Rotation() const
Returns the value of the Rotation keyword from the mapping group.
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
double PixelResolution() const
Returns the pixel resolution value from the PVL mapping group in meters/pixel.
@ Unknown
A type of error that cannot be classified as any of the other error types.
double m_minimumY
See minimumX description.
double GetX() const
Calculates the unrotated form of current x value.
double ToProjectionY(const double worldY) const
This method converts a world y value to a projection y value.
virtual QString Name() const =0
This method returns the name of the map projection.
ProjectionType projectionType() const
Returns an enum value for the projection type.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void SetXY(double x, double y)
This protected method is a helper for derived classes.
double m_y
This contains the rotated Y coordinate for a specific projection at the position indicated by m_latit...
void SetWorldMapper(WorldMapper *mapper)
If desired the programmer can use this method to set a world mapper to be used in the SetWorld,...
virtual double Resolution() const
This virtual method will the resolution of the world system relative to one unit in the projection sy...
virtual bool SetUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.
virtual double WorldY(const double projectionY) const =0
This pure virtual method will return a world Y coordinate given a projection Y coordinate in meters.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
PvlGroup m_mappingGrp
Mapping group that created this projection.
bool operator!=(const Projection &proj)
This method determines whether two map projection objects are not equal.
double GetY() const
Calculates the unrotated form of the current y value.
void setProjectionType(const ProjectionType ptype)
Sets the projection subclass type.
virtual double ProjectionY(const double worldY) const =0
This pure virtual method will return a projection Y coordinate in meters given a world Y coordinate.
@ Traverse
Search child objects.
Displacement is a signed length, usually in meters.
virtual bool SetUniversalGround(const double lat, const double lon)
This method is used to set the latitude/longitude which must be Planetocentric (latitude) and Positiv...
virtual double WorldY() const
This returns the world Y coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
bool IsGood() const
This indicates if the last invocation of SetGround, SetCoordinate, SetUniversalGround,...
Base class for Map Projections of plane shapes.
double meters() const
Get the displacement in meters.
double m_minimumX
The data elements m_minimumX, m_minimumY, m_maximumX, and m_maximumY are convience data elements when...
virtual bool IsEquatorialCylindrical()
This method returns true if the projection is equatorial cylindrical.
bool m_good
Indicates if the contents of m_x, m_y, m_latitude, and m_longitude are valid.
Base class for Map TProjections.
bool SetUnboundUniversalGround(const double coord1, const double coord2)
This method is used to set the latitude/longitude.
Create a mapping between a projection and other coordinate system.
double ToWorldY(const double projectionY) const
This method converts a projection y value to a world y value.
bool SetUniversalGround(const double ringRadius, const double ringLongitude)
This method is used to set the ring radius/longitude which must be PositiveEast/Domain360 (ring longi...
virtual double WorldX() const
This returns the world X coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
double m_rotation
Rotation of map (usually zero)
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
bool m_groundRangeGood
Indicates if the ground range (min/max lat/lons) were read from the labels.
const double Null
Value for an Isis Null pixel.
virtual bool operator==(const Projection &proj)
This method determines whether two map projection objects are equal by comparing the resolution,...
void SetComputedXY(double x, double y)
This protected method is a helper for derived classes.
Namespace for the standard library.
double ToWorldX(const double projectionX) const
This method converts a projection x value to a world x value.
double ToProjectionX(const double worldX) const
This method converts a world x value to a projection x value.
virtual ~Projection()
Destroys the Projection object.
double m_x
This contains the rotated X coordinate for a specific projection at theposition indicated by m_latitu...
static QString ToDMS(double angle)
Converts the given angle (in degrees) to degrees, minutes, seconds.
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
bool IsSky() const
Returns true if projection is sky and false if it is land.
Adds specific functionality to C++ strings.
static double ToHours(double angle)
Converts the given angle (in degrees) to hours by using the ratio 15 degrees per hour.
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
double m_maximumY
See minimumX description.
Base class for Map Projections.
double m_maximumX
See minimumX description.
static QString ToHMS(double angle)
Converts the given angle (in degrees) to hours, minutes, seconds.
ProjectionType
This enum defines the subclasses of Projection supported in Isis.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
virtual bool HasGroundRange() const
This indicates if the longitude direction type is positive west (as opposed to postive east).
This is free and unencumbered software released into the public domain.
virtual bool SetUnboundUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.