File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
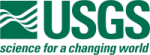 |
Isis 3 Programmer Reference
|
7 #include "RingPlaneProjection.h"
19 #include "Constants.h"
20 #include "Displacement.h"
22 #include "IException.h"
24 #include "Longitude.h"
25 #include "NaifStatus.h"
28 #include "PvlKeyword.h"
29 #include "SpecialPixel.h"
30 #include "WorldMapper.h"
73 if ((QString)
m_mappingGrp[
"RingLongitudeDirection"] ==
"Clockwise") {
76 else if ((QString)
m_mappingGrp[
"RingLongitudeDirection"] ==
"CounterClockwise") {
80 QString msg =
"Projection failed. Invalid value for keyword "
81 "[RingLongitudeDirection] must be "
82 "[Clockwise or CounterClockwise]";
87 if ((QString)
m_mappingGrp[
"RingLongitudeDomain"] ==
"360") {
90 else if ((QString)
m_mappingGrp[
"RingLongitudeDomain"] ==
"180") {
94 QString msg =
"Projection failed. Invalid value for keyword "
95 "[RingLongitudeDomain] must be [180 or 360]";
112 IString msg =
"Projection failed. "
119 IString msg =
"Projection failed. "
126 IString msg =
"Projection failed. "
127 "[MinimumRingRadius,MaximumRingRadius] of ["
130 +
"properly ordered";
135 IString msg =
"Projection failed. "
136 "[MinimumRingLongitude,MaximumRingLongitude] of ["
139 +
"properly ordered";
163 IString msg =
"Projection failed. Invalid label group [Mapping]";
182 if (!Projection::operator==(proj))
return false;
241 if (ringLongitude ==
Null) {
243 "Unable to convert to Clockwise. The given ring longitude value ["
247 double myRingLongitude = ringLongitude;
249 myRingLongitude *= -1;
254 else if (domain == 180) {
259 +
"] is not 180 or 360.";
263 return myRingLongitude;
281 if (ringLongitude ==
Null) {
283 "Unable to convert to CounterClockwise. The given ring longitude value ["
284 +
IString(ringLongitude) +
"] is invalid.",
287 double myRingLongitude = ringLongitude;
289 myRingLongitude *= -1;
294 else if (domain == 180) {
298 IString msg =
"Unable to convert ring longitude. Domain [" +
IString(domain)
299 +
"] is not 180 or 360.";
303 return myRingLongitude;
316 return "CounterClockwise";
353 if (ringLongitude ==
Null) {
355 "Unable to convert to 180 degree domain. The given ring longitude value ["
356 +
IString(ringLongitude) +
"] is invalid.",
371 if (ringLongitude ==
Null) {
373 "Unable to convert to 360 degree domain. The given ring longitude value ["
374 +
IString(ringLongitude) +
"] is invalid.",
377 double result = ringLongitude;
379 if ( (ringLongitude < 0.0 || ringLongitude > 360.0) &&
380 !qFuzzyCompare(ringLongitude, 0.0) && !qFuzzyCompare(ringLongitude, 360.0)) {
457 if (ringRadius ==
Null || ringLongitude ==
Null) {
547 if (ringRadius ==
Null || ringLongitude ==
Null) {
593 return ringLongitude;
657 double &minY,
double &maxY) {
710 if (ringRadius ==
Null || ringLongitude ==
Null) {
@ CounterClockwise
Ring longitude values increase in the counterclockwise direction.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
virtual bool SetGround(const double ringRadius, const double ringLongitude)
This method is used to set the ring radius/longitude (assumed to be of the correct LatitudeType,...
bool Has360Domain() const
This indicates if the ring longitude domain is 0 to 360 (as opposed to -180 to 180).
double m_maximumRingLongitude
Contains the maximum longitude for the entire ground range.
double UniversalRingLongitude()
This returns a universal ring longitude (clockwise in 0 to 360 domain).
WorldMapper * m_mapper
This points to a mapper passed into the SetWorldMapper method.
double MinimumRingRadius() const
This returns the minimum radius of the area of interest.
virtual PvlGroup Mapping()
This method is used to find the XY range for oblique aspect projections (non-polar projections) by "w...
static double To360Domain(const double lon)
This method converts an ring longitude into the 0 to 360 domain.
const double DEG2RAD
Multiplier for converting from degrees to radians.
@ Unknown
A type of error that cannot be classified as any of the other error types.
double m_minimumY
See minimumX description.
double RingLongitude() const
This returns a ring longitude with correct ring longitude direction and domain as specified in the la...
double LocalRadius() const
This returns a local radius.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void SetXY(double x, double y)
This protected method is a helper for derived classes.
virtual bool XYRange(double &minX, double &maxX, double &minY, double &maxY)
This method is used to determine the x/y range which completely covers the area of interest specified...
bool Has180Domain() const
This indicates if the longitude domain is -180 to 180 (as opposed to 0 to 360).
virtual double Resolution() const
This virtual method will the resolution of the world system relative to one unit in the projection sy...
double m_maximumRingRadius
Contains the maximum ring radius for the entire ground range.
virtual bool SetCoordinate(const double x, const double y)
This method is used to set the projection x/y.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Longitude force180Domain() const
This returns a longitude that is constricted to -180 to 180 degrees.
double m_ringRadius
This contain a ring radius value in m.
PvlGroup m_mappingGrp
Mapping group that created this projection.
void setProjectionType(const ProjectionType ptype)
Sets the projection subclass type.
void XYRangeCheck(const double ringRadius, const double ringLongitude)
This convience function is established to assist in the development of the XYRange virtual method.
double MaximumRingRadius() const
This returns the maximum radius of the area of interest.
This class is designed to encapsulate the concept of a Longitude.
std::string RingLongitudeDirectionString() const
This method returns the ring longitude direction as a string.
virtual double TrueScaleRingRadius() const
This method returns the radius of true scale.
std::string RingLongitudeDomainString() const
This method returns the ring longitude domain as a string.
bool IsGood() const
This indicates if the last invocation of SetGround, SetCoordinate, SetUniversalGround,...
Base class for Map Projections of plane shapes.
double m_minimumX
The data elements m_minimumX, m_minimumY, m_maximumX, and m_maximumY are convience data elements when...
Contains multiple PvlContainers.
static double ToCounterClockwise(const double ringLongitude, const int domain)
This method converts an ring longitude into the counterclockwise direction.
bool m_good
Indicates if the contents of m_x, m_y, m_latitude, and m_longitude are valid.
bool IsClockwise() const
This indicates if the longitude direction type is positive west (as opposed to postive east).
double RingRadius() const
This returns a radius.
double MaximumRingLongitude() const
This returns the maximum ring longitude of the area of interest.
double MinimumRingLongitude() const
This returns the minimum ring longitude of the area of interest.
virtual PvlGroup MappingRingRadii()
This function returns the ring radius keywords that this projection uses.
double m_ringLongitude
This contain a ring longitude value.
@ RingPlane
These projections are used to map ring planes.
bool SetUniversalGround(const double ringRadius, const double ringLongitude)
This method is used to set the ring radius/longitude which must be PositiveEast/Domain360 (ring longi...
RingLongitudeDirection m_ringLongitudeDirection
An enumerated type indicating the LongitudeDirection read from the labels.
bool IsCounterClockwise() const
This indicates if the longitude direction type is positive east (as opposed to postive west).
static double To180Domain(const double lon)
This method converts a ring longitude into the -180 to 180 domain.
bool m_groundRangeGood
Indicates if the ground range (min/max lat/lons) were read from the labels.
const double Null
Value for an Isis Null pixel.
void SetComputedXY(double x, double y)
This protected method is a helper for derived classes.
virtual ~RingPlaneProjection()
Destroys the Projection object.
Namespace for the standard library.
double Scale() const
This method returns the scale for mapping world coordinates into projection coordinates.
virtual bool operator==(const Projection &proj)
This method determines whether two map projection objects are equal by comparing the ring longitude d...
double degrees() const
Get the angle in units of Degrees.
double m_minimumRingRadius
Contains the minimum ring radius for the entire ground range.
static double ToClockwise(const double ringLongitude, const int domain)
This method converts an ring longitude into the clockwise direction.
virtual PvlGroup MappingRingLongitudes()
This function returns the ring longitude keywords that this projection uses.
Adds specific functionality to C++ strings.
@ Clockwise
Ring longitude values increase in the clockwise direction.
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
double m_maximumY
See minimumX description.
double m_minimumRingLongitude
Contains the minimum longitude for the entire ground range.
int m_ringLongitudeDomain
This integer is either 180 or 360 and is read from the labels.
Base class for Map Projections.
double m_maximumX
See minimumX description.
Longitude force360Domain() const
This returns a longitude that is constricted to 0-360 degrees.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
virtual bool HasGroundRange() const
This indicates if the longitude direction type is positive west (as opposed to postive east).
This is free and unencumbered software released into the public domain.
double UniversalRingRadius()
This returns a universal radius, which is just the radius in meters.