File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
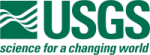 |
Isis 3 Programmer Reference
|
1 #ifndef CubeDataThread_h
2 #define CubeDataThread_h
12 template<
typename T>
class QList;
14 template<
typename A,
typename B>
struct QPair;
15 template<
typename A,
typename B>
class QMap;
24 class UniversalGroundMap;
67 bool mustOpenReadWrite =
false);
83 void ReadCube(
int cubeId,
int startSample,
int startLine,
84 int endSample,
int endLine,
int band,
void *caller);
85 void ReadWriteCube(
int cubeId,
int startSample,
int startLine,
86 int endSample,
int endLine,
int band,
void *caller);
155 int instanceNum,
bool &exact);
157 void GetCubeData(
int cubeId,
int ss,
int sl,
int es,
int el,
int band,
158 void *caller,
bool sharedLock);
160 void AcquireLock(QReadWriteLock *lockObject,
bool readLock);
unsigned int p_currentLocksWaiting
Number of locks being attempted that re-entered the event loop.
virtual ~CubeDataThread()
This class is a self-contained thread, so normally it would be bad to simply delete it.
bool FreeBrick(int brickIndex)
This is used internally to delete bricks when possible.
bool p_stopping
This is set to help the shutdown process when deleted.
void DoneWithData(int, const Isis::Brick *)
When done processing with a brick (reading or writing) this slot needs to be signalled to free locks ...
CubeDataThread()
This constructs a CubeDataThread().
This is free and unencumbered software released into the public domain.
void ReadWriteCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
This slot should be connected to and upon receiving a signal it will begin the necessary cube I/O to ...
CubeDataThread(const CubeDataThread &cdt)
Assigning CubeDataThreads to eachother is bad, so this has been intentionally not implemented!
File name manipulation and expansion.
QMap< int, QPair< bool, Cube * > > * p_managedCubes
This is a list of the opened cubes.
unsigned int p_currentId
This is the unique id counter for cubes.
int OverlapIndex(const Brick *initial, int cubeId, int instanceNum, bool &exact)
This is a searching method used to identify overlapping data already in memory.
void AcquireLock(QReadWriteLock *lockObject, bool readLock)
This method is exclusively used to acquire locks.
Buffer for containing a three dimensional section of an image.
Encapsulation of Cube I/O with Change Notifications.
int FindCubeId(const Cube *) const
Given a Cube pointer, return the cube ID associated with it.
void AddChangeListener()
You must call this method after connecting to the BrickChanged signal, otherwise you are not guarante...
void BrickChanged(int cubeId, const Isis::Brick *data)
DO NOT CONNECT TO THIS SIGNAL WITHOUT CALLING AddChangeListener().
const Cube * GetCube(int cubeId) const
This returns a constant pointer to a Cube at the given Cube ID.
int AddCube(const FileName &fileName, bool mustOpenReadWrite=false)
This method is designed to be callable from any thread before data is requested, though no known side...
QList< int > * p_managedDataSources
This is the associated cube ID with each brick.
const CubeDataThread & operator=(CubeDataThread rhs)
Assigning CubeDataThreads to eachother is bad, so this has been intentionally not implemented!
void ReadReady(void *requester, int cubeId, const Isis::Brick *data)
This signal will be emitted when ReadCube has finished processing.
int BricksInMemory()
This is a helper method for both testing/debugging and general information that provides the current ...
UniversalGroundMap * GetUniversalGroundMap(int cubeId) const
This returns a new Universal Ground Map given a Cube ID.
QMutex * p_threadSafeMutex
This locks the member variable p_managedCubes and p_managedData itself.
IO Handler for Isis Cubes.
void ReadWriteReady(void *requester, int cubeId, Isis::Brick *data)
This signal will be emitted when ReadWriteCube has finished processing.
int p_numChangeListeners
This is the number of shaded locks to put on a brick when changes made.
void RemoveChangeListener()
You must call this method after disconnecting from the BrickChanged signal, otherwise bricks cannot b...
void RemoveCube(int cubeId)
Removes a cube from this lock manager.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.
void ReadCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
This slot should be connected to and upon receiving a signal it will begin the necessary cube I/O to ...
void GetCubeData(int cubeId, int ss, int sl, int es, int el, int band, void *caller, bool sharedLock)
This helper method reads in cube data and handles the locking of similar bricks appropriately.
This is free and unencumbered software released into the public domain.
QList< QPair< QReadWriteLock *, Brick * > > * p_managedData
This is a list of bricks in memory and their locks.