File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
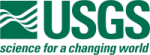 |
Isis 3 Programmer Reference
|
8 #include "CubeDataThread.h"
10 #include <QApplication>
15 #include <QReadWriteLock>
24 #include "IException.h"
26 #include "UniversalGroundMap.h"
72 while (!isFinished()) {
73 QThread::yieldCurrentThread();
79 delete (*p_managedData)[i].first;
80 delete (*p_managedData)[i].second;
126 bool mustOpenReadWrite) {
133 if (!mustOpenReadWrite) {
147 newEntry.first =
true;
148 newEntry.second = newCube;
175 newEntry.first =
false;
176 newEntry.second = cube;
200 QString msg =
"CubeDataThread::RemoveCube failed because cube ID [";
201 msg += QString::number(cubeId);
202 msg +=
"] not found";
208 QString msg =
"CubeDataThread::RemoveCube failed cube ID [";
209 msg += QString::number(cubeId);
210 msg +=
"] has requested Bricks";
216 delete i.value().second;
217 i.value().second = NULL;
259 int band,
void *caller,
bool sharedLock) {
261 Brick *requestedBrick = NULL;
265 - ss + 1, el - sl + 1, 1);
272 bool exactMatch =
false;
273 int index =
OverlapIndex(requestedBrick, cubeId, instance, exactMatch);
276 while (index != -1) {
283 (*p_managedData)[index].first->unlock();
289 (*p_managedData)[index].first->unlock();
304 index =
OverlapIndex(requestedBrick, cubeId, instance, exactMatch);
309 if (exactIndex == -1) {
316 managedDataEntry.first =
new QReadWriteLock();
320 managedDataEntry.second = requestedBrick;
330 if (el - sl + 1 != (*
p_managedData)[exactIndex].second->LineDimension())
353 while (i.hasNext()) {
355 if (i.value().second == cubeToFind)
359 "Cube does not exist in this CubeDataThread", _FILEINFO_);
378 while (!lockObject->tryLockForRead()) {
383 QThread::yieldCurrentThread();
386 qApp->sendPostedEvents(
this, 0);
393 while (!lockObject->tryLockForWrite()) {
398 QThread::yieldCurrentThread();
401 qApp->sendPostedEvents(
this, 0);
428 int endSample,
int endLine,
int band,
434 msg +=
"] is not a valid cube ID";
438 GetCubeData(cubeId, startSample, startLine, endSample, endLine, band,
463 int startLine,
int endSample,
int endLine,
464 int band,
void *caller) {
468 msg +=
"] is not a valid cube ID";
472 GetCubeData(cubeId, startSample, startLine, endSample, endLine, band,
490 int instanceNum,
bool &exact) {
494 int startSample = overlapping->
Sample(0);
495 int endSample = overlapping->
Sample(overlapping->
size() - 1);
496 int startLine = overlapping->
Line(0);
497 int endLine = overlapping->
Line(overlapping->
size() - 1);
498 int startBand = overlapping->
Band(0);
499 int endBand = overlapping->
Band(overlapping->
size() - 1);
503 for (
int knownBrick = 0; knownBrick <
p_managedData->size(); knownBrick++) {
504 int sourceCube = (*p_managedDataSources)[knownBrick];
507 if (sourceCube != cubeId)
511 (*p_managedData)[knownBrick];
513 Brick &brick = *managedBrick.second;
517 int compareSampStart = brick.
Sample(0);
518 int compareSampEnd = brick.
Sample(brick.
size() - 1);
519 int compareLineStart = brick.
Line(0);
520 int compareLineEnd = brick.
Line(brick.
size() - 1);
521 int compareBandStart = brick.
Band(0);
522 int compareBandEnd = brick.
Band(brick.
size() - 1);
524 bool overlap =
false;
527 if (compareSampStart >= startSample && compareSampStart <= endSample) {
532 if (compareSampEnd >= startSample && compareSampEnd <= endSample) {
537 if (compareLineStart >= startLine && compareLineStart <= endLine) {
542 if (compareLineEnd >= startLine && compareLineEnd <= endLine) {
547 if (compareBandStart >= startBand && compareBandStart <= endBand) {
552 if (compareBandEnd >= startBand && compareBandEnd <= endBand) {
557 if (compareSampStart == startSample && compareSampEnd == endSample
558 && compareLineStart == startLine && compareLineEnd == endLine
559 && compareBandStart == startBand && compareBandEnd == endBand) {
568 if (instanceNum < 0) {
588 ASSERT(brickDone != NULL);
590 bool exactMatch =
false;
591 bool writeLock =
false;
593 int index =
OverlapIndex(brickDone, cubeId, instance, exactMatch);
595 while (index != -1) {
599 index =
OverlapIndex(brickDone, cubeId, instance, exactMatch);
607 IString msg =
"Overlapping data had write locks";
616 IString msg =
"Overlapping data had write locks";
621 (*p_managedData)[index].first->unlock();
628 Brick cpy(*brickDone);
633 (*p_managedData)[index].first->unlock();
660 (*p_managedData)[index].first->unlock();
663 (*p_managedData)[index].first->unlock();
671 index =
OverlapIndex(brickDone, cubeId, instance, exactMatch);
688 if (!(*
p_managedData)[brickIndex].first->tryLockForWrite()) {
690 "CubeDataThread::FreeBrick called on a locked brick",
694 (*p_managedData)[brickIndex].first->unlock();
701 delete (*p_managedData)[brickIndex].first;
702 delete (*p_managedData)[brickIndex].second;
710 delete (*p_managedData)[i].first;
711 delete (*p_managedData)[i].second;
739 return numBricksInMemory;
753 "Invalid Cube ID [" +
IString(cubeId) +
"]",
770 "Invalid Cube ID [" +
IString(cubeId) +
"]",
unsigned int p_currentLocksWaiting
Number of locks being attempted that re-entered the event loop.
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
virtual ~CubeDataThread()
This class is a self-contained thread, so normally it would be bad to simply delete it.
bool FreeBrick(int brickIndex)
This is used internally to delete bricks when possible.
bool p_stopping
This is set to help the shutdown process when deleted.
void DoneWithData(int, const Isis::Brick *)
When done processing with a brick (reading or writing) this slot needs to be signalled to free locks ...
CubeDataThread()
This constructs a CubeDataThread().
This is free and unencumbered software released into the public domain.
void ReadWriteCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
This slot should be connected to and upon receiving a signal it will begin the necessary cube I/O to ...
File name manipulation and expansion.
QMap< int, QPair< bool, Cube * > > * p_managedCubes
This is a list of the opened cubes.
unsigned int p_currentId
This is the unique id counter for cubes.
int OverlapIndex(const Brick *initial, int cubeId, int instanceNum, bool &exact)
This is a searching method used to identify overlapping data already in memory.
void AcquireLock(QReadWriteLock *lockObject, bool readLock)
This method is exclusively used to acquire locks.
Buffer for containing a three dimensional section of an image.
int FindCubeId(const Cube *) const
Given a Cube pointer, return the cube ID associated with it.
void AddChangeListener()
You must call this method after connecting to the BrickChanged signal, otherwise you are not guarante...
void BrickChanged(int cubeId, const Isis::Brick *data)
DO NOT CONNECT TO THIS SIGNAL WITHOUT CALLING AddChangeListener().
const Cube * GetCube(int cubeId) const
This returns a constant pointer to a Cube at the given Cube ID.
int AddCube(const FileName &fileName, bool mustOpenReadWrite=false)
This method is designed to be callable from any thread before data is requested, though no known side...
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
QList< int > * p_managedDataSources
This is the associated cube ID with each brick.
void ReadReady(void *requester, int cubeId, const Isis::Brick *data)
This signal will be emitted when ReadCube has finished processing.
int BricksInMemory()
This is a helper method for both testing/debugging and general information that provides the current ...
UniversalGroundMap * GetUniversalGroundMap(int cubeId) const
This returns a new Universal Ground Map given a Cube ID.
QMutex * p_threadSafeMutex
This locks the member variable p_managedCubes and p_managedData itself.
IO Handler for Isis Cubes.
void ReadWriteReady(void *requester, int cubeId, Isis::Brick *data)
This signal will be emitted when ReadWriteCube has finished processing.
int p_numChangeListeners
This is the number of shaded locks to put on a brick when changes made.
int Band(const int index=0) const
Returns the band position associated with a shape buffer index.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void RemoveChangeListener()
You must call this method after disconnecting from the BrickChanged signal, otherwise bricks cannot b...
void RemoveCube(int cubeId)
Removes a cube from this lock manager.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.
int size() const
Returns the total number of pixels in the shape buffer.
void ReadCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
This slot should be connected to and upon receiving a signal it will begin the necessary cube I/O to ...
void GetCubeData(int cubeId, int ss, int sl, int es, int el, int band, void *caller, bool sharedLock)
This helper method reads in cube data and handles the locking of similar bricks appropriately.
Adds specific functionality to C++ strings.
int Sample(const int index=0) const
Returns the sample position associated with a shape buffer index.
void open(const QString &cfile, QString access="r")
This method will open an isis cube for reading or reading/writing.
int Line(const int index=0) const
Returns the line position associated with a shape buffer index.
This is free and unencumbered software released into the public domain.
QList< QPair< QReadWriteLock *, Brick * > > * p_managedData
This is a list of bricks in memory and their locks.