Loading [MathJax]/jax/output/NativeMML/config.js
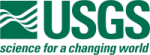 |
Isis 3 Programmer Reference
|
1 #ifndef BulletTargetShape_h
2 #define BulletTargetShape_h
10 #include <QSharedPointer>
11 #include <QScopedPointer>
14 #include "IsisBullet.h"
15 #include "BulletClosestRayCallback.h"
48 btCollisionObject *
body()
const;
50 btScalar maximumDistance()
const;
virtual ~BulletTargetShape()
Desctructor.
btScalar m_maximumDistance
! The Bullet collision object for the body
void writeBullet(const QString &btName) const
Write a serialized version of the target shape to a Bullet file.
Container for cube-like labels.
void setMaximumDistance()
Calculate and save the maximum distance across the body.
static BulletTargetShape * load(const QString &dem, const Pvl *conf=0)
Load a DEM file into the target shape.
static BulletTargetShape * loadDSK(const QString &dem, const Pvl *conf=0)
Load a DSK in Bullet.
static BulletTargetShape * loadCube(const QString &dem, const Pvl *conf=0)
Load an ISIS cube type DEM in Bullet.
void setTargetBody(btCollisionObject *body)
Set the Bullet shape object to this object instance
QSharedPointer< btCollisionObject > m_btbody
! The name of the body
QString name() const
Return name of the target shape.
BulletTargetShape()
Default empty constructor.
Bullet Target Shape for planetary bodies.
static BulletTargetShape * loadPC(const QString &dem, const Pvl *conf=0)
Load a point cloud type DEM in Bullet.
This is free and unencumbered software released into the public domain.
btCollisionObject * body() const
Return a pointer to the Bullet target object/shape.