File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
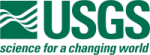 |
Isis 3 Programmer Reference
|
72 unsigned int Width()
const;
unsigned int Precision() const
Get the Column's precision.
@ Decimal
decimal alignment
Column::Type p_type
Type of the data in the Column.
Align
Alignment of data in the Column.
unsigned int Width() const
Get the Column's width.
unsigned int p_precision
Precision of the data in the Column.
Column::Type DataType() const
Returns the type of data this column will contain.
void SetAlignment(Column::Align alignment)
Sets the alignment of the Column.
void SetPrecision(unsigned int precision)
Sets the precision of the Column, for real number values.
QString p_name
Name of the Column.
QString Name() const
Get the Column's name.
Column::Align Alignment() const
Get the Column's alignment.
void SetType(Column::Type type)
Sets the data type of the Column.
Type
Type of data in the Column.
Store and/or manipulate pixel values.
unsigned int p_width
Width of the Column.
void SetName(QString name)
Sets the Column name, or header.
Column::Align p_align
Alignment of the data in the Column.
void SetWidth(unsigned int width)
Sets the width of the Column, in text columns.
This is free and unencumbered software released into the public domain.