File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
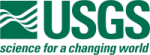 |
Isis 3 Programmer Reference
|
1 #ifndef ConcurrentControlNetReader_h
2 #define ConcurrentControlNetReader_h
16 #include <QStringList>
18 #include "ProgressBar.h"
49 void read(QString filename);
61 void updateProgressValue();
62 void mappedFinished();
73 QPointer<ProgressBar> m_progressBar;
74 QPointer<QTimer> m_progressUpdateTimer;
84 const QPair<FileName, Progress *> &, Control *> {
void nullify()
Initializes members to NULL.
This reads a control net in the background.
~ConcurrentControlNetReader()
This destructor will cancel all running threads and block.
ConcurrentControlNetReader()
Allocates memory at construction instead of as needed.
This represents an ISIS control net in a project-based GUI interface.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.
void read(QString filename)
This is free and unencumbered software released into the public domain.
QFutureWatcher< Control * > * m_watcher
provides SIGNALS / SLOTS for FileNameToControlFunctor
This is free and unencumbered software released into the public domain.