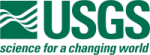 |
Isis 3 Programmer Reference
|
1 #ifndef ControlNetVersioner_h
2 #define ControlNetVersioner_h
15 #include <QSharedPointer>
18 #include "ControlPoint.h"
19 #include "ControlPointV0001.h"
20 #include "ControlPointV0002.h"
21 #include "ControlPointV0003.h"
31 class ControlPointV0001;
32 class ControlPointV0002;
33 class ControlPointV0003;
419 QString
netId()
const;
QString creationDate() const
Returns the date and time that the network was created.
ControlNetHeaderV0005 m_header
Header containing information about the whole network.
void readProtobufV0002(const Pvl &header, const FileName netFile, Progress *progress=NULL)
Read a protobuf version 2 control network and prepare the data to be converted into a control network...
Contains Pvl Groups and Pvl Objects.
void createHeader(const ControlNetHeaderV0001 header)
Create the internal header from a V0001 header.
void readPvlV0001(const PvlObject &network, Progress *progress=NULL)
read a version 1 Pvl control network and convert the data into control points.
void readPvlV0005(const PvlObject &network, Progress *progress=NULL)
read a version 5 Pvl control network and convert the data into control points.
This is free and unencumbered software released into the public domain.
A container for the information stored in a version 2 ControlPoint.
void readPvl(const Pvl &network, Progress *progress=NULL)
Read a Pvl control network and prepare the data to be converted into a control network.
File name manipulation and expansion.
void readProtobufV0001(const Pvl &header, const FileName netFile, Progress *progress=NULL)
Read a protobuf version 1 control network and prepare the data to be converted into a control network...
ControlPoint * takeFirstPoint()
Returns the first point stored in the versioner's internal list.
Container for cube-like labels.
void readProtobuf(const Pvl &header, const FileName netFile, Progress *progress=NULL)
Read a protobuf control network and prepare the data to be converted into a control network.
ControlNetHeaderV0001 ControlNetHeaderV0004
Typedef for consistent naming of containers for version 4.
ControlNetVersioner()
Default constructor. Intentially un-implemented.
~ControlNetVersioner()
Destroy a ControlNetVersioner.
int writeFirstPoint(std::fstream *output)
This will write the first control point to a file stream.
QString description() const
Returns the network's description.
void read(const FileName netFile, Progress *progress=NULL)
Read a control network file and prepare the data to be converted into a control network.
void readPvlV0004(const PvlObject &network, Progress *progress=NULL)
read a version 4 Pvl control network and convert the data into control points.
QString userName() const
Returns the name of the last person or program to modify the network.
QString netId() const
Returns the ID for the network.
void readPvlV0002(const PvlObject &network, Progress *progress=NULL)
read a version 2 Pvl control network and convert the data into control points.
ControlPointV0003 ControlPointV0005
Typedef for consistent naming of containers for version 5.
A container for the information stored in a version 3 and 4 ControlPoint.
QString targetName() const
Returns the target for the network.
void readPvlV0003(const PvlObject &network, Progress *progress=NULL)
read a version 3 Pvl control network and convert the data into control points.
Program progress reporter.
ControlMeasure * createMeasure(const ControlPointFileEntryV0002_Measure &)
Create a pointer to a ControlMeasure from a V0006 file.
int numPoints() const
Returns the number of points that have been read in or are ready to write out.
ControlNetVersioner(const ControlNetVersioner &other)
Copy constructor.
void readProtobufV0005(const Pvl &header, const FileName netFile, Progress *progress=NULL)
Read a protobuf version 5 control network and prepare the data to be converted into a control network...
ControlPointV0003 ControlPointV0004
Typedef for consistent naming of containers for version 4.
void write(FileName netFile)
This will write a control net file object to disk.
void writeHeader(std::fstream *output)
This will read the binary protobuffer control network header to an fstream.
Pvl toPvl()
Generates a Pvl file from the currently stored control points and header.
ControlPoint * createPoint(ControlPointV0001 &point)
Create a pointer to a latest version ControlPoint from an object in a V0001 control net file.
ControlNetHeaderV0001 ControlNetHeaderV0003
Typedef for consistent naming of containers for version 3.
bool m_ownsPoints
Flag if the versioner owns the control points stored in it.
QString lastModificationDate() const
Returns the date and time of the last modification to the network.
ControlNetHeaderV0001 ControlNetHeaderV0005
Typedef for consistent naming of containers for version 5.
A container for the information stored in a version 1 ControlPoint.
ControlNetVersioner & operator=(const ControlNetVersioner &other)
Asssignment operator.
This is free and unencumbered software released into the public domain.
Handle various control network file format versions.
QList< ControlPoint * > m_points
ControlPoints that are read in from a file or ready to be written out to a file.
ControlNetHeaderV0001 ControlNetHeaderV0002
Typedef for consistent naming of containers for version 2.