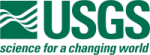 |
Isis 3 Programmer Reference
|
9 #include "CorrelationMatrix.h"
11 #include <QDataStream>
16 #include <QStringList>
17 #include <QtCore/qmath.h>
19 #include "IException.h"
20 #include "LinearAlgebra.h"
22 #include "PvlObject.h"
23 #include "SparseBlockMatrix.h"
75 if (storedMatrixData.
name() !=
"CorrelationMatrixData") {
76 QString msg =
"This Pvl Object does not have the correct correlation information. The Object "
77 "you are looking for is called CorrelationMatrixData.";
86 QString msg =
"Could not find the Covariance Matrix .dat file name.";
91 QString corrFileName = storedMatrixData.
findKeyword(
"CorrelationMatrixFileName")[0];
92 if (corrFileName ==
"NULL") {
100 QString msg =
"Could not find the Correlation Matrix .dat file name.";
106 imgsIt = storedMatrixData.
findGroup(
"ImagesAndParameters").begin();
107 while ( imgsIt != storedMatrixData.
findGroup(
"ImagesAndParameters").end() ) {
114 QString msg =
"Could not get Images and Parameters from ImagesAndParameters group.";
165 if (&other !=
this) {
206 QString msg =
"Cannot compute correlation matrix without a specified file name. Use "
207 "setCorrelationFileName(FileName) before calling computeCorrelationMatrix().";
219 matrixInput.open(QIODevice::ReadOnly);
220 matrixOutput.open(QIODevice::WriteOnly);
223 QDataStream inStream(&matrixInput);
224 QDataStream outStream(&matrixOutput);
226 double firstParam1 = 0,
232 while ( !inStream.atEnd() ) {
236 int numOfBlocks = sbcm.size();
237 int lastBlock = numOfBlocks - 1;
238 int numDiagonals = sbcm[lastBlock]->size1();
241 for (
int i = 0; i < numDiagonals; i++) {
242 double val = ( *(sbcm[lastBlock]) )(i, i);
247 QMapIterator<int, LinearAlgebra::Matrix *> block(sbcm);
249 while ( block.hasNext() ) {
251 for (
int row = 0; row < (int)block.value()->size1(); row++) {
252 for (
int column = 0; column < (int)block.value()->size2(); column++) {
254 ( *block.value() )(row, column) = ( *block.value() )(row, column) /
260 param2 = firstParam2;
262 firstParam1 += block.value()->size1();
263 param1 = firstParam1;
266 param1 = firstParam1;
267 firstParam2 += block.value()->size2();
268 param2 = firstParam2;
276 matrixOutput.close();
369 QString(
"correlation") );
477 PvlObject corrMatInfo(
"CorrelationMatrixData");
482 PvlGroup imgsAndParams(
"ImagesAndParameters");
484 while ( imgParamIt.hasNext() ) {
486 imgsAndParams +=
PvlKeyword( imgParamIt.key(), imgParamIt.value().join(
",") );
488 corrMatInfo += imgsAndParams;
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
FileName correlationFileName()
Public access for the correlation matrix file name.
Contains Pvl Groups and Pvl Objects.
~CorrelationMatrix()
Destructor.
A single keyword-value pair.
This is free and unencumbered software released into the public domain.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
QMap< QString, QStringList > * m_imagesAndParameters
This map holds the images used to create this matrix and their associated parameters.
void setImagesAndParameters(QMap< QString, QStringList > imagesAndParameters)
Set the qmap of images and parameters.
CorrelationMatrix()
Default Constructor.
File name manipulation and expansion.
void retrieveVisibleElements(int x, int y)
Extract requested area from correlation matrix This method will open the correlation matrix file and ...
FileName covarianceFileName()
Public access for the covariance matrix file name.
void computeCorrelationMatrix()
Read covariance matrix and compute correlation values This method reads the covariance matrix in from...
PvlObject pvlObject()
This method creates a Pvl group with the information necessary to recreate this correlation matrix.
This is a container for the correlation matrix that comes from a bundle adjust.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Contains multiple PvlContainers.
FileName * m_correlationFileName
FileName of the correlation matrix.
void retrieveThreeVisibleBlocks()
Display only part of a matrix This method will be used when the matrix is too big to display the whol...
QString name() const
Returns the container name.
CorrelationMatrix & operator=(const CorrelationMatrix &other)
Equal Operator.
QList< double > * m_diagonals
List of the parameter values.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void setCovarianceFileName(FileName covarianceFileName)
Set the qmap of images and parameters.
bool hasCovMat()
Check if the correlation matrix has a covariance matrix This is used to make sure the covariance matr...
FileName * m_covarianceFileName
FileName of the covariance matrix calculated when the bundle was run.
QList< PvlKeyword >::iterator PvlKeywordIterator
The keyword iterator.
PvlKeyword & findKeyword(const QString &kname, FindOptions opts)
Finds a keyword in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within this...
bool isValid()
This is the public accessor for the list of elements that should be displayed in the current view.
QList< SparseBlockColumnMatrix > * m_visibleBlocks
This will be the three blocks (or whole matrix depending on size) that apply to the given area.
QList< SparseBlockColumnMatrix > * visibleBlocks()
Get the visible part of the matrix.
QMap< QString, QStringList > * imagesAndParameters()
Public access for the qmap of images and parameters.
This is free and unencumbered software released into the public domain.
void setCorrelationFileName(FileName correlationFileName)
Set the qmap of images and parameters.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
void retrieveWholeMatrix()
This method will read the matrix in from the file and hold on to the whole thing.