File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
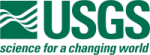 |
Isis 3 Programmer Reference
|
1 #ifndef SparseBlockMatrix_h
2 #define SparseBlockMatrix_h
19 #include <boost/numeric/ublas/fwd.hpp>
22 #include "LinearAlgebra.h"
58 public QMap< int, LinearAlgebra::Matrix * > {
80 void print(std::ostream& outstream);
125 public QMap< int, LinearAlgebra::Matrix * > {
142 void copyToBoost(boost::numeric::ublas::compressed_matrix<double>& B);
145 void print(std::ostream& outstream);
208 void print(std::ostream& outstream);
210 bool write(std::ofstream &fp_out,
bool binary=
true);
int getLeadingColumnsForBlock(int nblockColumn)
Sums and returns the number of columns in each matrix block prior to nblockColumn.
void printClean(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
void copyToBoost(boost::numeric::ublas::compressed_matrix< double > &B)
Copies a SparseBlockRowMatrix to a Boost compressed_matrix.
SparseBlockRowMatrix & operator=(const SparseBlockRowMatrix &src)
"Equals" operator.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
This is free and unencumbered software released into the public domain.
void copy(const SparseBlockRowMatrix &src)
Copy method.
void zeroBlocks()
Sets all elements of all matrix blocks to zero.
~SparseBlockRowMatrix()
Destructor.
void wipe()
Deletes all pointer elements and removes them from the map.
void setStartColumn(int nStartColumn)
Sets starting column for block in full matrix.
SparseBlockColumnMatrix & operator=(const SparseBlockColumnMatrix &src)
"Equals" operator.
void print(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
int numberOfRows()
Returns total number of rows in map (this needs to be clarified and maybe rewritten).
boost::numeric::ublas::matrix< double > Matrix
Definition for an Isis::LinearAlgebra::Matrix of doubles.
void printClean(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
SparseBlockColumnMatrix()
Default constructor.
~SparseBlockColumnMatrix()
Destructor.
int startColumn() const
Sets starting column for block in full matrix.
int numberOfElements()
Returns total number of matrix elements in map (NOTE: NOT the number of matrix blocks).
bool insertMatrixBlock(int nColumnBlock, int nRows, int nCols)
Inserts a "newed" LinearAlgebra::Matrix pointer of size (nRows, nCols) into the map with the block co...
int getLeadingRowsForBlock(int nblockRow)
Sums and returns the number of rows in each matrix block prior to nblockRow.
int numberOfElements()
Returns number of matrix elements in matrix.
~SparseBlockMatrix()
Destructor.
int m_startColumn
starting column for this Block Column in full matrix e.g.
void copy(const SparseBlockColumnMatrix &src)
Copy method.
void wipe()
Deletes all pointer elements and removes them from the list.
int numberOfOffDiagonalBlocks()
Returns number of off-diagonal matrix blocks.
void print(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
LinearAlgebra::Matrix * getBlock(int column, int row)
Returns pointer to boost matrix at position (column, row).
bool insertMatrixBlock(int nRowBlock, int nRows, int nCols)
Inserts a "newed" LinearAlgebra::Matrix pointer of size (nRows, nCols) into the map with the block ro...
void print(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
void printClean(std::ostream &outstream)
Prints matrix blocks to std output stream out for debugging.
int getLeadingColumnsForBlock(int nblockColumn)
Sums and returns the number of columns in each matrix block prior to nblockColumn.
void zeroBlocks()
Sets all elements of all matrix blocks to zero.
void zeroBlocks()
Sets all elements of all matrix blocks to zero.
bool setNumberOfColumns(int n)
Initializes number of columns (SparseBlockColumnMatrix).
This is free and unencumbered software released into the public domain.
int numberOfColumns()
Returns total number of columns in map (NOTE: NOT the number of matrix blocks).
int numberOfDiagonalBlocks()
Returns number of diagonal matrix blocks (equivalent to size - there is one per column).
int numberOfElements()
Returns total number of matrix elements in map (NOTE: NOT the number of matrix blocks).
void copy(const SparseBlockMatrix &src)
Copy method.
std::istream & operator>>(std::istream &is, CSVReader &csv)
Input read operator for input stream sources.
int numberOfBlocks()
Returns total number of blocks in matrix.
void wipe()
Deletes all pointer elements and removes them from the map.
bool insertMatrixBlock(int nColumnBlock, int nRowBlock, int nRows, int nCols)
Inserts a "newed" boost LinearAlgebra::Matrix pointer of size (nRows, nCols) into the matrix at nColu...
SparseBlockMatrix & operator=(const SparseBlockMatrix &src)
"Equals" operator.
This is free and unencumbered software released into the public domain.