Loading [MathJax]/jax/output/NativeMML/config.js
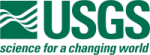 |
Isis 3 Programmer Reference
|
1 #ifndef LinearAlgebra_h
2 #define LinearAlgebra_h
13 #include <boost/numeric/ublas/matrix.hpp>
14 #include <boost/numeric/ublas/io.hpp>
22 #define BOOST_UBLAS_NO_STD_CERR
100 typedef boost::numeric::ublas::matrix<double>
Matrix;
110 typedef boost::numeric::ublas::symmetric_matrix<double, boost::numeric::ublas::upper>
SymmetricMatrix;
120 typedef boost::numeric::ublas::vector<double>
Vector;
203 static void setVec3(
Vector *v,
double v0,
double v1,
double v2);
204 static void setVec4(
Vector *v,
double v0,
double v1,
double v2,
double v3);
206 static Vector vector(
double v0,
double v1,
double v2,
double v3);
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
static Matrix multiply(const Matrix &matrix1, const Matrix &matrix2)
Returns the product of two matrices.
static Vector add(const Vector &vector1, const Vector &vector2)
Adds the two given vectors.
static Vector project(const Vector &vector1, const Vector &vector2)
Compute the vector projection of vector1 onto vector2.
This is free and unencumbered software released into the public domain.
static bool isIdentity(const Matrix &matrix)
Determines whether the given matrix is the identity.
static bool isOrthogonal(const Matrix &matrix)
Determines whether the given matrix is orthogonal by verifying that the matrix and its tranpose are i...
static Vector subtract(const Vector &vector1, const Vector &vector2)
Subtracts the right vector from the left vector.
~LinearAlgebra()
Destructor for a LinearAlgebra object.
QPair< Vector, Angle > AxisAngle
Definition for an Axis-Angle pair.
static double determinant(const Matrix &matrix)
Returns the determinant of the given 3x3 matrix.
static Vector perpendicular(const Vector &vector1, const Vector &vector2)
Finds the unique vector P such that A = V + P, V is parallel to B and P is perpendicular to B,...
static bool isZero(const Matrix &matrix)
Determines whether the given matrix is filled with zereos.
static double absoluteMaximum(const Vector &vector)
Returns the maximum norm (L-infinity norm) for the given vector.
static Matrix zeroMatrix(int rows, int columns)
Returns a matrix with given dimensions that is filled with zeroes.
static double innerProduct(const Vector &vector1, const Vector &vector2)
Computes the inner product of the given vectors.
static Vector rotate(const Vector &vector, const Vector &axis, Angle angle)
Rotates a vector about an axis vector given a specified angle.
static double magnitude(const Vector &vector)
Computes the magnitude (i.e., the length) of the given vector using the Euclidean norm (L2 norm).
static Matrix transpose(const Matrix &matrix)
Returns the transpose of the given matrix.
static Vector vector(double v0, double v1, double v2)
Constructs a 3 dimensional vector with the given component values.
static bool isEmpty(const Vector &vector)
Determines whether the given vector is empty (i.e.
static void setRow(Matrix &matrix, const Vector &vector, int rowIndex)
Sets the row of the given matrix to the values of the given vector.
boost::numeric::ublas::matrix< double > Matrix
Definition for an Isis::LinearAlgebra::Matrix of doubles.
static Vector row(const Matrix &matrix, int rowIndex)
Returns a vector whose components match those of the given matrix row.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
LinearAlgebra()
Default constructor for a LinearAlgebra object.
static Matrix inverse(const Matrix &matrix)
Returns the inverse of a 2x2 or 3x3 matrix.
static Matrix outerProduct(const Vector &vector1, const Vector &vector2)
Computes the outer product of the given vectors.
static bool isRotationMatrix(const Matrix &matrix)
Determines whether the given matrix is a rotation matrix.
static double dotProduct(const Vector &vector1, const Vector &vector2)
Computes the dot product of the given vectors.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
static Vector column(const Matrix &matrix, int columnIndex)
Returns a vector whose components match those of the given matrix column.
static AxisAngle toAxisAngle(const Matrix &rotationMatrix)
Converts a rotation's representation from a matrix to a axis of rotation and its corresponding rotati...
static Matrix pseudoinverse(const Matrix &matrix)
Returns the pseudoinverse of a matrix.
boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper > SymmetricMatrix
Definition for an Isis::LinearAlgebra::SymmetrixMatrix of doubles with an upper configuration.
static Vector zeroVector(int size)
Returns a vector of given length that is filled with zeroes.
static Vector normalize(const Vector &vector)
Returns a unit vector that is codirectional with the given vector by dividing each component of the v...
static Matrix toMatrix(const AxisAngle &axisAngle)
Converts a rotation's representation from an axis of rotation and its corresponding rotation angle to...
Defines an angle and provides unit conversions.
static QList< EulerAngle > toEulerAngles(const Matrix &rotationMatrix, const QList< int > axes)
Converts a rotation's representation from a matrix to a set of Euler angles with corresponding axes.
static void setVec4(Vector *v, double v0, double v1, double v2, double v3)
Fills the first four elements of the given vector with the given values.
This is free and unencumbered software released into the public domain.
static void setVec3(Vector *v, double v0, double v1, double v2)
Fills the first three elements of the given vector with the given values.
static Matrix identity(int size)
Returns the identity matrix of size NxN.
static Vector crossProduct(const Vector &vector1, const Vector &vector2)
Returns the cross product of two vectors.
QPair< Angle, int > EulerAngle
Definition for an EulerAngle pair.
static Vector normalizedCrossProduct(const Vector &vector1, const Vector &vector2)
Divides each vector by its corresponding absolute maximum, computes the cross product of the new vect...
static bool isUnit(const Vector &vector)
Determines whether the given vector is a unit vector.
This class holds all static methods to perform linear algebra operations on vectors and matrices.
static Vector subVector(const Vector &v, int start, int size)
Constructs a vector of given size using the given vector and starting index.
static void setColumn(Matrix &matrix, const Vector &vector, int columnIndex)
Sets the column of the given matrix to the values of the given vector.
This is free and unencumbered software released into the public domain.
static Vector toQuaternion(const Matrix &rotationMatrix)
Converts a rotation's representation from a matrix to a unit quaternion.