File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
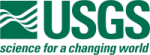 |
Isis 3 Programmer Reference
|
12 #include "DbProfile.h"
14 #include "DatabaseFactory.h"
17 #include <QStringList>
24 QString Database::_actualConnectionName =
"";
54 _name = _actualConnectionName;
55 if((dbConn ==
Connect) && isValid()) {
57 QString mess =
"Failed to open database default database [" +
_name;
77 _name = _actualConnectionName;
78 if((dbConn ==
Connect) && isValid()) {
80 QString mess =
"Failed to open database specified as " +
_name;
105 QSqlDatabase(init(connName, driverType)), _name(connName) {
106 _name = _actualConnectionName;
126 _name(profile.Name()) {
127 _name = _actualConnectionName;
128 if((dbConn ==
Connect) && isValid()) {
130 QString mess =
"Failed to open database with profile " +
_name;
344 const QString &driverType) {
346 _actualConnectionName = connName;
352 if(connName.isEmpty() && driverType.isEmpty()) {
354 _actualConnectionName = factory->
getDefault();
355 return (factory->
create(_actualConnectionName));
366 if((!connName.isEmpty()) && (driverType.isEmpty())) {
368 _actualConnectionName = connName;
369 return (factory->
create(connName));
380 return (factory->
create(driverType, connName));
406 mess <<
"Database/profile [" << profile.
Name() <<
"] is not valid!" << ends;
410 _actualConnectionName = profile.
Name();
419 _actualConnectionName = profile(
"Name");
425 QString mess =
"Failed to connect to database using profile " +
433 QString mess =
"Unable to create database from " + profile.
Name();
437 QString mess =
"Unknown exception while creating database from profile "
456 if(profile.
exists(
"Host")) {
457 db.setHostName(profile(
"Host"));
460 if(profile.
exists(
"DbName")) {
461 db.setDatabaseName(profile(
"DbName"));
464 if(profile.
exists(
"User")) {
465 db.setUserName(profile(
"User"));
468 if(profile.
exists(
"Password")) {
469 db.setPassword(profile(
"Password"));
472 if(profile.
exists(
"Port")) {
474 db.setPort(profile(
"Port").
toInt(&ok));
477 mess <<
"Invalid port number [" << profile(
"Port") <<
"] in profile "
478 << profile(
"Name") << ends;
483 if(profile.
exists(
"Options")) {
484 db.setConnectOptions(profile(
"Options"));
512 return (tables(QSql::Tables));
525 return (tables(QSql::Views));
537 return (tables(QSql::SystemTables));
551 QString errmess = message +
" - DatabaseError = " +
bool isPersistant() const
Checks persistancy state of a database instantiation.
QSqlDatabase create(const QString &driver, const QString &dbname)
Create a database using the named driver.
QString Name() const
Returns the name of this property.
void tossDbError(const QString &message, const char *f, int l) const
Generic exception tosser.
void setDefault(const QString &name)
Sets the default name of the database.
bool exists(const QString &key) const
Checks for the existance of a keyword.
@ Connect
Connect to database immediately.
static DbProfile getProfile(const QString &name)
Retrieves the named database access profile.
static void remove(const QString &name)
Removes the named database from pool.
DbProfile getProfile(const QString &name="") const
Get the specified database access profile.
void remove(const QString &dbname)
Removes the database from the connection pool.
QSqlDatabase init(const DbProfile &profile, Access dbConn=Connect)
Create and initialize a new database connection from a DbProfile.
bool isPersistant(const QString &name) const
Checks if the database resource is persistant.
void destroy(const QString &dbname)
Removes the database from the connection pool and destroys it.
QStringList getViews() const
Returns a vector string containing all views within the database.
static bool addAccessConfig(const QString &confFile)
Adds a user specifed access configuration file to system.
bool isAvailable(const QString &dbname="") const
Check for availablity of a database connection resource.
void configureAccess(QSqlDatabase &db, const DbProfile &profile)
Set access parameters from a database DbProfile access specification.
void makePersistant()
Makes this instance persistant.
void add(const QSqlDatabase &db, const QString &name, bool setAsDefault=false)
Adds the database to the connection pool making it persistant.
virtual ~Database()
Database destructor.
int toInt(const QString &string)
Global function to convert from a string to an integer.
bool addAccessProfile(const QString &profileFile)
Establishes an access profile for subsequent database connections.
bool isValid() const
Reports if this is a valid profile.
Database clone(const QString &name) const
Clones this database into another giving it another name.
A DbProfile is a container for access parameters to a database.
@ DoNotConnect
Do not connect to database.
void setAsDefault()
Sets this database connection/profile as the default.
Create database interfaces using access profiles or generic drivers.
QStringList getSystemTables() const
Returns vector strings of all available system tables in the database.
static DatabaseFactory * getInstance()
Returns and instance of this DatabaseFactory singleton.
QStringList getTables() const
Returns a vector string containing all the tables in the database.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
Isis database class providing generalized access to a variety of databases.
QString getDefault() const
Returns the name of the default database.
Access
Access status for database creation.
Database()
Default database constructor.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
QString _name
Needed due to peculiar issues with Database construction techniques.