Loading [MathJax]/jax/output/NativeMML/config.js
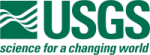 |
Isis 3 Programmer Reference
|
18 #include <QStringList>
19 #include <QCoreApplication>
20 #include <QSqlDatabase>
22 #include "DatabaseFactory.h"
24 #include "Preference.h"
31 DatabaseFactory *DatabaseFactory::_factory = 0;
57 DatabaseFactory::DatabaseFactory() : _defProfName(
""), _profiles(),
58 _defDatabase(
""), _dbList() {
64 QCoreApplication *cApp = QCoreApplication::instance();
66 static char **argv = 0;
70 argv[0] =
new char[1024];
71 strcpy(argv[0],
"DatabaseFactory");
76 new QCoreApplication(argc, argv);
169 if(acp.
exists(
"DefaultProfile")) {
208 std::vector<QString> plist;
236 QString profName(name);
262 std::vector<QString> dblist;
263 for(
int i = 0 ; i < drivers.
size() ; i++) {
264 dblist.push_back(drivers.
key(i).ToQt());
281 if(!drivers.
exists(dbname)) {
284 return (QSqlDatabase::isDriverAvailable(drivers.
get(dbname)));
299 QString name(dbname);
303 return (dbdrivers.
exists(name));
316 QString name(dbname);
320 return (QSqlDatabase::contains(name));
359 const QString &dbname) {
364 QString mess =
"Driver [" + driver +
"] for database [" + dbname
365 +
"] does not exist";
371 return (QSqlDatabase::addDatabase(drivers.
get(driver), dbname));
393 QString mess =
"Database [" + dbname +
"] does not exist";
437 QSqlDatabase::removeDatabase(name);
487 Preference &userPref = Preference::Preferences();
520 if(drivers) dblist += QSqlDatabase::drivers();
521 if(connections) dblist += QSqlDatabase::connectionNames();
523 for(
int i = 0 ; i < dblist.size() ; i++) {
524 QString dbname(dblist.at(i));
525 dbDrivers.
add(dbname, dbname);
531 if(dbDrivers.
exists(
"QPSQL")) {
532 dbDrivers.
add(
"PostgreSQL",
"QPSQL");
536 if(dbDrivers.
exists(
"QMYSQL")) {
537 dbDrivers.
add(
"MySQL",
"QMYSQL");
541 if(dbDrivers.
exists(
"QOCI")) {
542 dbDrivers.
add(
"Oracle",
"QOCI");
546 if(dbDrivers.
exists(
"QSQLITE")) {
547 dbDrivers.
add(
"SQLite",
"QSQLITE");
565 QSqlDatabase::drivers();
580 QStringList dblist = QSqlDatabase::connectionNames();
581 for(
int i = 0 ; i < dblist.size() ; i++) {
582 QSqlDatabase::removeDatabase(dblist[i]);
bool isConnected(const QString &dbname) const
Determines if the database resource is connected.
DatabaseFactory()
Constructor establishing the startup state of this singleton.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
QSqlDatabase create(const QString &driver, const QString &dbname)
Create a database using the named driver.
QString Name() const
Returns the name of this property.
bool exists(const QString &key) const
Checks for the existance of a keyword.
std::vector< QString > getProfileList() const
Return list of names of currently available profiles.
DbProfile getProfile(const QString &name="") const
Get the specified database access profile.
DbAccess manages programatic access to a database through profiles.
File name manipulation and expansion.
void init()
Initializes this object upon instantiation.
QString _defDatabase
Name of default database.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void remove(const QString &dbname)
Removes the database from the connection pool.
bool fileExists() const
Returns true if the file exists; false otherwise.
T & get(const K &key)
Returns the value associated with the name provided.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
int size() const
Returns the size of the collection.
int remove(const K &key)
Removes and entry from the list.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
std::vector< QString > available() const
Determine what database drivers are available.
bool isDriverAvailable(const QString &driver) const
Check for the existance of a specific database driver.
void addProfile(const DbProfile &profile)
Adds a database access profile to the list of profiles.
bool isPersistant(const QString &name) const
Checks if the database resource is persistant.
void destroy(const QString &dbname)
Removes the database from the connection pool and destroys it.
bool isAvailable(const QString &dbname="") const
Check for availablity of a database connection resource.
void add(const QSqlDatabase &db, const QString &name, bool setAsDefault=false)
Adds the database to the connection pool making it persistant.
bool exists(const K &key) const
Checks the existance of a particular key in the list.
Contains multiple PvlContainers.
const K & key(int nth) const
Returns the nth key in the collection.
void selfDestruct()
Destroy all elements associated with this object.
void loadDrivers()
Load any drivers explicity.
static DatabaseFactory * _factory
Pointer to self (singleton)
bool addAccessProfile(const QString &profileFile)
Establishes an access profile for subsequent database connections.
Databases _dbList
Maintains active databases.
Reads user preferences from a data file.
A DbProfile is a container for access parameters to a database.
void add(const K &key, const T &value)
Adds the element to the list.
Create database interfaces using access profiles or generic drivers.
static DatabaseFactory * getInstance()
Returns and instance of this DatabaseFactory singleton.
Namespace for the standard library.
Collector/container for arbitrary items.
int profileCount() const
Reports the number of user profiles to access this database.
Drivers getResourceList(bool drivers, bool connections) const
Get a list of available database drivers and connections.
bool Equal(const std::string &str) const
Compare a string to the object IString.
const DbProfile getProfile(const QString &name="") const
Retrieves the specified access profile.
static void DieAtExit()
Exit termination routine.
QString value(const QString &key, int nth=0) const
Returns the specified value for the given keyword.
~DatabaseFactory()
Destructor implements self-destruction of this object.
QString _defProfName
Default profile name.
void initPreferences()
Initializes user database preferences.
This is free and unencumbered software released into the public domain.
QString ToQt() const
Retuns the object string as a QString.
Profiles _profiles
Maintain list of profiles.