File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
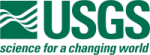 |
Isis 3 Programmer Reference
|
12 #include <QApplication>
15 #include <QHBoxLayout>
19 #include <QMessageBox>
21 #include <QStackedWidget>
22 #include <QToolButton>
26 #include "MdiCubeViewport.h"
27 #include "RubberBandTool.h"
28 #include "SpecialPixel.h"
30 #include "Workspace.h"
61 action->setIcon(QPixmap(
toolIconDir() +
"/color_line.png"));
62 action->setToolTip(
"Image Edit (E)");
63 action->setShortcut(Qt::Key_E);
66 "<b>Function:</b> Edit active viewport \
67 <p><b>Shortcut:</b> E</p> ";
68 action->setWhatsThis(text);
86 container->setObjectName(
"EditToolActiveToolBarWidget");
98 "<b>Function:</b> The shape in the image that will be replaced with \
99 a new value. If Horizontal line is chosen, clicking anywhere on the \
100 image will cause all samples on that line of the cube to be replaced \
101 with the replacement value. If Vertical Line is chosen, a v ...";
117 "<b>Function:</b> The value which will be used to replace image data. ";
127 "<b>Function:</b> This is the dn used to replace image data";
136 "<b>Function:</b> Undo last edit operation";
146 "<b>Function:</b> Redo last undo operation";
157 "<b>Function:</b> Save any changes made, these changes are finalized";
168 "<b>Function:</b> Save any changes made to the file specified, these changes are finalized";
174 QHBoxLayout *layout =
new QHBoxLayout;
175 layout->setMargin(0);
183 layout->addStretch(1);
184 container->setLayout(layout);
186 m_container = container;
265 if (vp == NULL)
return;
277 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cannot open cube read/write");
282 QMessageBox::information((
QWidget *)parent(),
283 "Error",
"Cannot edit in color mode");
287 int issamp, isline, iesamp, ieline;
288 double ssamp, sline, esamp, eline;
294 if (!rubberBandTool()->isValid())
return;
297 if ((r.width() < 1) || (r.height() < 1))
return;
302 issamp = (int)(ssamp + 0.5);
303 isline = (int)(sline + 0.5);
304 iesamp = (int)(esamp + 0.5);
305 ieline = (int)(eline + 0.5);
308 if (issamp < 0) issamp = 0;
309 if (iesamp < 0) iesamp = 0;
310 if (isline < 0) isline = 0;
311 if (ieline < 0) ieline = 0;
319 if (issamp > iesamp || isline > ieline) {
320 QMessageBox::information((
QWidget *)parent(),
321 "Error",
"Rectangle is out of bounds");
328 if (!rubberBandTool()->isValid())
return;
329 vp->
viewportToCube(rubberBandTool()->vertices()[0].rx(), rubberBandTool()->vertices()[0].ry(),
331 vp->
viewportToCube(rubberBandTool()->vertices()[1].rx(), rubberBandTool()->vertices()[1].ry(),
334 QLine l((
int)ssamp, (
int)sline, (
int)esamp, (
int)eline);
339 if (linePts->empty()) {
340 QMessageBox::information((
QWidget *)parent(),
341 "Error",
"No points in edit line");
346 issamp = std::min(linePts->front()->x(), linePts->back()->x());
347 isline = std::min(linePts->front()->y(), linePts->back()->y());
348 iesamp = std::max(linePts->front()->x(), linePts->back()->x());
349 ieline = std::max(linePts->front()->y(), linePts->back()->y());
357 writeToCube(iesamp, issamp, ieline, isline, linePts);
358 if (linePts)
delete linePts;
362 connect(newViewport, SIGNAL(saveChanges(
CubeViewport *)),
364 connect(newViewport, SIGNAL(discardChanges(
CubeViewport *)),
366 connect(newViewport, SIGNAL(destroyed(
QObject *)),
399 if (vp == NULL)
return;
417 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cannot open cube read/write");
422 QMessageBox::information((
QWidget *)parent(),
423 "Error",
"Cannot edit in color mode");
427 int issamp, isline, iesamp, ieline;
432 if (m == Qt::RightButton &&
436 issamp = (int)(ssamp + 0.5);
437 isline = (int)(sline + 0.5);
442 p_dn = (*pntBrick)[0];
452 if ((ssamp < 0.5) || (sline < 0.5) ||
454 QApplication::beep();
457 issamp = (int)(ssamp + 0.5);
458 isline = (int)(sline + 0.5);
490 int nsamps = iesamp - issamp + 1;
491 int nlines = ieline - isline + 1;
513 while(!temp->isEmpty()) {
527 for(
int i = 0; linePts && i < (int)linePts->size(); i++) {
528 QPoint *pt = (*linePts)[i];
531 int brickIndex = (il - isline) * nsamps + (is - issamp);
532 (*brick)[brickIndex] = (double)
p_dn;
536 for(
int i = 0; i < brick->
size(); i++)(*brick)[i] = (double)
p_dn;
558 QMessageBox::information((
QWidget *)parent(),
"Error",
"Not enough memory to complete this operation.");
569 Brick *redoBrick = NULL;
572 if (vp == NULL)
return;
576 QApplication::beep();
582 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cube is Read Only");
588 Brick *brick = s->top();
602 redo->push(redoBrick);
632 QMessageBox::information((
QWidget *)parent(),
"Error",
"Not enough memory to complete this operation.");
649 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cube is Read Only");
666 for(
int i = undo->count() - 1; i >= marker; i--) {
667 Brick *brick = undo->at(i);
674 marker = marker - undo->count();
682 for(
int i = redo->count() - 1; i >= redo->count() - marker; i--) {
683 Brick *brick = redo->at(i);
693 QMessageBox::information((
QWidget *)parent(),
"Error",
694 "Not enough memory to complete this operation.");
704 Brick *undoBrick = NULL;
707 if (vp == NULL)
return;
711 QApplication::beep();
717 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cube is Read Only");
722 Brick *brick = s->top();
738 undo->push(undoBrick);
767 QMessageBox::information((
QWidget *)parent(),
"Error",
"Not enough memory to complete this operation.");
811 while(!temp->isEmpty()) {
818 while(!temp->isEmpty()) {
847 int x, y, xinc, yinc;
853 int sx = line.p1().x();
854 int ex = line.p2().x();
855 int sy = line.p1().y();
856 int ey = line.p2().y();
876 slope = (double)(ex - sx) / (double)(ey - sy);
878 for(i = 0; i < ysize; i++) {
879 x = (int)(slope * (
double)(y - sy) + (
double) sx + 0.5);
882 if (x >= 0 && y >= 0 && x <= vp->cubeSamples() && y <= vp->
cubeLines()) {
883 QPoint *pt =
new QPoint;
886 points->push_back(pt);
891 else if (xsize == 1) {
893 if (sx >= 0 && sy >= 0 && sx <= vp->cubeSamples() && sy <= vp->
cubeLines()) {
894 QPoint *pt =
new QPoint;
897 points->push_back(pt);
901 slope = (double)(ey - sy) / (double)(ex - sx);
903 for(i = 0; i < xsize; i++) {
904 y = (int)(slope * (
double)(x - sx) + (
double) sy + 0.5);
907 if (x >= 0 && y >= 0 && x <= vp->cubeSamples() && y <= vp->
cubeLines()) {
908 QPoint *pt =
new QPoint;
911 points->push_back(pt);
929 rubberBandTool()->
enable(RubberBandTool::LineMode);
932 else if (index == 4) {
933 rubberBandTool()->
enable(RubberBandTool::RectangleMode);
int cubeLines() const
Return the number of lines in the cube.
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
void setCaption()
Change the caption on the viewport title bar.
Cube display widget for certain Isis MDI applications.
virtual QString fileName() const
Returns the opened cube's filename.
int SampleDimension() const
Returns the number of samples in the shape buffer.
This is free and unencumbered software released into the public domain.
void cubeContentsChanged(QRect rect)
Calle dhwen the contents of the cube changes.
This is free and unencumbered software released into the public domain.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
const double His
Value for an Isis High Instrument Saturation pixel.
Buffer for containing a three dimensional section of an image.
const double Hrs
Value for an Isis High Representation Saturation pixel.
const double Lrs
Value for an Isis Low Representation Saturation pixel.
int LineDimension() const
Returns the number of lines in the shape buffer.
Widget to display Isis cubes for qt apps.
int cubeSamples() const
Return the number of samples in the cube.
const double Null
Value for an Isis Null pixel.
const double Lis
Value for an Isis Low Instrument Saturation pixel.
bool isReadOnly() const
Test if the opened cube is read-only, that is write operations will fail if this is true.
PixelType pixelType() const
void cubeChanged(bool changed)
This method is called when the cube has changed or changes have been finalized.
void write(Blob &blob, bool overwrite=true)
This method will write a blob of data (e.g.
int size() const
Returns the total number of pixels in the shape buffer.
void viewportToCube(int x, int y, double &sample, double &line) const
Turns a viewport into a cube.
int Sample(const int index=0) const
Returns the sample position associated with a shape buffer index.
void open(const QString &cfile, QString access="r")
This method will open an isis cube for reading or reading/writing.
int Line(const int index=0) const
Returns the line position associated with a shape buffer index.
This is free and unencumbered software released into the public domain.
void reopen(QString access="r")
This method will reopen an isis sube for reading or reading/writing.