File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
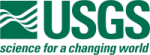 |
Isis 3 Programmer Reference
|
1 #ifndef EmbreeTargetShape_h
2 #define EmbreeTargetShape_h
13 #include <embree2/rtcore.h>
14 #include <embree2/rtcore_ray.h>
17 #include <pcl/io/auto_io.h>
18 #include <pcl/point_types.h>
19 #include <pcl/PolygonMesh.h>
29 #include "LinearAlgebra.h"
47 const std::vector<double> &direction);
86 const std::vector<double> &direction);
147 QString
name()
const;
166 void initMesh(pcl::PolygonMesh::Ptr mesh);
static void multiHitFilter(void *userDataPtr, RTCMultiHitRay &ray)
Filter function for collecting multiple hits during ray intersection.
RTCDevice m_device
!< The point cloud representation of the target.
pcl::PointCloud< pcl::PointXYZ > m_cloud
!< A boost shared pointer to the polygon mesh representation of the target.
int lastHit
Index of the last hit in the hit containers.
int numberOfVertices() const
Return the number of vertices in the target shape.
int v0
The index of the first vertex in the tin.
File name manipulation and expansion.
RTCOcclusionRay()
Default constructor for RTCOcclussionRay.
float a
Extra float for aligning memory.
float y
Vertex y position.
Container for cube-like labels.
double maximumSceneDistance() const
Return the maximum distance within the scene.
virtual ~EmbreeTargetShape()
Desctructor.
int numberOfPolygons() const
Return the number of polygons in the target shape.
unsigned hitGeomIDs[16]
IDs of the geometries (bodies) hit.
int lastHit
Index of the last hit in the hit containers.
unsigned hitPrimIDs[16]
IDs of the primitives (trinagles) hit.
Struct for capturing occluded plates when using embree::rtcintersectscene.
float hitUs[16]
Barycentric u coordinate of the hits.
void addIndices(int geomID)
Adds the polygon vertex indices from the internalized polygon mesh to the Embree scene.
pcl::PolygonMesh::Ptr readPC(FileName file)
Read a PointCloudLibrary file into a PointCloudLibrary polygon mesh.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
void initMesh(pcl::PolygonMesh::Ptr mesh)
Internalize a PointCloudLibrary polygon mesh in the target shape.
Struct for capturing multiple intersections when using embree::rtcintersectscene.
int v2
The index of the third vertex in the tin.
RTCBounds sceneBounds() const
Returns the bounds of the Embree scene.
RTCScene m_scene
!< The Embree device for rendering the scene.
void addVertices(int geomID)
Adds the vertices from the internalized vertex point cloud to the Embree scene.
Embree Target Shape for planetary bodies.
RayHitInformation getHitInformation(RTCMultiHitRay &ray, int hitIndex)
Extract the intersection point and unit surface normal from an RTCMultiHitRay that has been intersect...
float z
Vertex z position.
unsigned ignorePrimID
IDs of the primitives (trinagles) which should be ignored.
bool isOccluded(RTCOcclusionRay &ray)
Check if a ray intersects the target body.
void intersectRay(RTCMultiHitRay &ray)
Intersect a ray with the target shape.
float x
Vertex x position.
pcl::PolygonMesh::Ptr m_mesh
!< The name of the target.
EmbreeTargetShape()
Default empty constructor.
RTCMultiHitRay()
Default constructor for RTCMultiHitRay.
Container for a tin, or triangular polygon.
static void occlusionFilter(void *userDataPtr, RTCOcclusionRay &ray)
Filter function for collecting multiple primitiveIDs This function is called by the Embree library du...
int v1
The index of the second vertex in the tin.
float hitVs[16]
Barycentric v coordinate of the hits.
QString name() const
Return the name of the target shape.
bool isValid() const
Return if a valid mesh is internalized and ready for use.
pcl::PolygonMesh::Ptr readDSK(FileName file)
Read a NAIF type 2 DSK file into a PointCloudLibrary polygon mesh.
This is free and unencumbered software released into the public domain.