File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
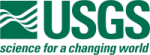 |
Isis 3 Programmer Reference
|
13 #include <QStringList>
16 #include "LeastSquares.h"
17 #include "OverlapNormalization.h"
23 class OverlapStatistics;
127 void addHolds(QString holdListName);
129 void calculateStatistics(
double samplingPercent,
133 void recalculateStatistics(QString inStatsFileName);
134 void importStatistics(QString instatsFileName);
135 void applyCorrection(QString toListName);
138 void write(QString outstatsFileName);
140 double evaluate(
double dn,
int imageIndex,
int bandIndex)
const;
155 void addGain(
double gain) {
156 gains.push_back(gain);
159 void addOffset(
double offset) {
160 offsets.push_back(offset);
163 void addAverage(
double average) {
164 avgs.push_back(average);
167 double getGain(
int index)
const {
171 double getOffset(
int index)
const {
172 return offsets[index];
175 double getAverage(
int index)
const {
179 double evaluate(
double dn,
int index)
const {
180 double result =
Null;
182 double gain = gains[index];
183 if (m_sType != OverlapNormalization::GainsWithoutNormalization) {
184 double offset = offsets[index];
185 double avg = avgs[index];
186 result = (dn - avg) * gain + offset + avg;
196 vector<double> gains;
197 vector<double> offsets;
221 m_linc = (int) (100.0 / percent + 0.5);
226 void operator()(
Buffer &in)
const;
229 virtual void addStats(
Buffer &in)
const;
246 m_adjustment = adjustment;
257 void loadInputs(QString fromListName);
258 void setInput(
int index, QString value);
263 void calculateBandStatistics();
264 void calculateOverlapStatistics();
266 virtual void fillOutList(
FileList &outList, QString toListName);
267 virtual void errorCheck(QString fromListName);
269 void generateOutputs(
FileList &outList);
270 void loadOutputs(
FileList &outList, QString toListName);
275 void clearAdjustments();
278 void clearNormalizations();
279 void clearOverlapStatistics();
281 void addValid(
int count);
282 void addInvalid(
int count);
285 void setSolved(
bool solved);
286 bool isSolved()
const;
290 QVector<int> validateInputStatistics(QString instatsFileName);
310 vector<ImageAdjustment *> m_adjustments;
This class is used to accumulate statistics on double arrays.
SolutionType
Enumeration for whether user/programmer wants to calculate new gains, offsets, or both when solving.
Contains Pvl Groups and Pvl Objects.
LeastSquares::SolveMethod m_lsqMethod
Least squares method for solving normalization correcitve factors.
CalculateFunctor(Statistics *stats, double percent)
Constructs a CalculateFunctor.
vector< int > m_holdIndices
Indices of images being held.
bool m_recalculating
Indicates if recalculating with loaded statistics.
int m_maxCube
Number of input images.
Container for cube-like labels.
Pvl * m_results
Calculation results and normalization corrective factors (if solved)
bool m_normsSolved
Indicates if corrective factors were solved.
Buffer for reading and writing cube data.
bool m_wtopt
Whether or not overlaps should be weighted.
This class is used as a functor to apply adjustments (equalize) to an image.
OverlapNormalization::SolutionType m_sType
The normalization solution type for solving normalizations (offsets, gains, or both)
Contains multiple PvlContainers.
int m_invalidCnt
Number of invalid overlaps.
Calculate the bases and multipliers for normalizing overlapping "data sets" (e.g.,...
int m_linc
Line increment value when calculating statistics.
vector< OverlapNormalization * > m_overlapNorms
Normalization data for input images.
This class is used as a functor calculate image statistics.
int m_maxBand
Number of bands in each input image.
vector< OverlapStatistics * > m_overlapStats
Calculated overlap statistics.
const double Null
Value for an Isis Null pixel.
This class can be used to calculate, read in, and/or apply equalization statistics for a list of file...
QStringList m_badFiles
List of image names that don't overlap.
int m_mincnt
Minimum number of pixels for an overlap to be considered valid.
Namespace for the standard library.
const ImageAdjustment * m_adjustment
ImageAdjustment to equalize image.
vector< bool > m_alreadyCalculated
Which images that have statistics already calculated.
double m_samplingPercent
Percentage of the lines to consider when gathering cube and overlap statistics (process-by-line)
Internalizes a list of files.
vector< bool > m_doesOverlapList
Which images have a valid overlap.
int m_validCnt
Number of valid overlaps.
Statistics * m_stats
Calculated statistics.
This is free and unencumbered software released into the public domain.