File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
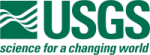 |
Isis 3 Programmer Reference
|
13 #include "Constants.h"
15 #include "SpecialPixel.h"
16 #include "XmlStackedHandler.h"
20 class QXmlStreamWriter;
24 class XmlStackedHandlerReader;
107 void AddData(
const double *data,
const unsigned int count);
108 void AddData(
const double data);
110 void RemoveData(
const double *data,
const unsigned int count);
116 double ValidMinimum()
const;
117 double ValidMaximum()
const;
118 bool InRange(
const double value);
119 bool AboveRange(
const double value);
120 bool BelowRange(
const double value);
133 double BestMinimum(
const double percent = 99.5)
const;
134 double BestMaximum(
const double percent = 99.5)
const;
135 double ZScore(
const double value)
const;
147 bool RemovedData()
const;
151 void save(QXmlStreamWriter &stream,
const Project *project)
const;
154 QDataStream &
write(QDataStream &stream)
const;
155 QDataStream &read(QDataStream &stream);
173 virtual bool startElement(
const QString &namespaceURI,
const QString &localName,
174 const QString &qName,
const QXmlAttributes &atts);
175 virtual bool characters(
const QString &ch);
176 virtual bool endElement(
const QString &namespaceURI,
const QString &localName,
177 const QString &qName);
185 QString m_xmlHandlerCharacters;
const double ValidMaximum
The maximum valid double value for Isis pixels.
double SumSquare() const
Returns the sum of all the squared data.
BigInt NullPixels() const
Returns the total number of NULL pixels encountered.
This class is used to accumulate statistics on double arrays.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
double Sum() const
Returns the sum of all the data.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
BigInt TotalPixels() const
Returns the total number of pixels processed (valid and invalid).
double m_validMinimum
Minimum valid pixel value.
BigInt m_lrsPixels
Count of low instrument saturation pixels processed.
BigInt m_hisPixels
Count of high instrument representation pixels processed.
BigInt m_underRangePixels
Count of pixels less than the valid range.
void fromPvl(const PvlGroup &inStats)
Unserializes a Statistics object from a pvl group.
double Maximum() const
Returns the absolute maximum double found in all data passed through the AddData method.
void Reset()
Reset all accumulators and counters to zero.
BigInt HrsPixels() const
Returns the total number of high representation saturation (HRS) pixels encountered.
BigInt ValidPixels() const
Returns the total number of valid pixels processed.
BigInt OverRangePixels() const
Returns the total number of pixels over the valid range encountered.
Manage a stack of content handlers for reading XML files.
double m_sum
The sum accumulator, i.e. the sum of added data values.
BigInt m_totalPixels
Count of total pixels processed.
The main project for ipce.
void RemoveData(const double *data, const unsigned int count)
Remove an array of doubles from the accumulators and counters.
BigInt HisPixels() const
Returns the total number of high instrument saturation (HIS) pixels encountered.
double Variance() const
Computes and returns the variance.
BigInt UnderRangePixels() const
Returns the total number of pixels under the valid range encountered.
BigInt LisPixels() const
Returns the total number of low instrument saturation (LIS) pixels encountered.
double ChebyshevMaximum(const double percent=99.5) const
This method returns a maximum such that X percent of the data will fall with K standard deviations of...
double Rms() const
Computes and returns the rms.
Contains multiple PvlContainers.
BigInt m_hrsPixels
Count of high instrument saturation pixels processed.
double StandardDeviation() const
Computes and returns the standard deviation.
double Minimum() const
Returns the absolute minimum double found in all data passed through the AddData method.
double m_sumsum
The sum-squared accumulator, i.e.
long long int BigInt
Big int.
double BestMaximum(const double percent=99.5) const
This method returns the better of the absolute maximum or the Chebyshev maximum.
double m_minimum
Minimum double value encountered.
double ZScore(const double value) const
This method returns the better of the z-score of the given value.
BigInt m_lisPixels
Count of low representation saturation pixels processed.
double m_validMaximum
Maximum valid pixel value.
double m_maximum
Maximum double value encountered.
BigInt OutOfRangePixels() const
Returns the total number of pixels outside of the valid range encountered.
PvlGroup toPvl(QString name="Statistics") const
Serialize statistics as a pvl group.
double Average() const
Computes and returns the average.
const double ValidMinimum
The minimum valid double value for Isis pixels.
double BestMinimum(const double percent=99.5) const
This method returns the better of the absolute minimum or the Chebyshev minimum.
BigInt m_validPixels
Count of valid pixels (non-special) processed.
Statistics(QObject *parent=0)
Constructs an IsisStats object with accumulators and counters set to zero.
XML Handler that parses XMLs in a stack-oriented way.
QDataStream & write(QDataStream &stream) const
Order saved must match the offsets in the static compoundH5DataType() method.
double ChebyshevMinimum(const double percent=99.5) const
This method returns a minimum such that X percent of the data will fall with K standard deviations of...
virtual ~Statistics()
Destroys the IsisStats object.
BigInt m_nullPixels
Count of null pixels processed.
BigInt LrsPixels() const
Returns the total number of low representation saturation (LRS) pixels encountered.
std::istream & operator>>(std::istream &is, CSVReader &csv)
Input read operator for input stream sources.
bool m_removedData
Indicates the RemoveData method was called which implies m_minimum and m_maximum are invalid.
This is free and unencumbered software released into the public domain.
BigInt m_overRangePixels
Count of pixels greater than the valid range.