File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
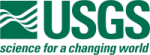 |
Isis 3 Programmer Reference
|
7 #include "Statistics.h"
13 #include <QXmlStreamWriter>
17 #include "IException.h"
21 #include "PvlKeyword.h"
22 #include "XmlStackedHandlerReader.h"
57 m_sumsum(other.m_sumsum),
58 m_minimum(other.m_minimum),
59 m_maximum(other.m_maximum),
60 m_validMinimum(other.m_validMinimum),
61 m_validMaximum(other.m_validMaximum),
62 m_totalPixels(other.m_totalPixels),
63 m_validPixels(other.m_validPixels),
64 m_nullPixels(other.m_nullPixels),
65 m_lrsPixels(other.m_lrsPixels),
66 m_lisPixels(other.m_lisPixels),
67 m_hrsPixels(other.m_hrsPixels),
68 m_hisPixels(other.m_hisPixels),
69 m_underRangePixels(other.m_underRangePixels),
70 m_overRangePixels(other.m_overRangePixels),
71 m_removedData(other.m_removedData) {
142 for(
unsigned int i = 0; i < count; i++) {
143 double value = data[i];
176 else if (AboveRange(data)) {
179 else if (BelowRange(data)) {
208 for(
unsigned int i = 0; i < count; i++) {
209 double value = data[i];
235 else if (AboveRange(data)) {
238 else if (BelowRange(data)) {
248 QString msg =
"You are removing non-existant data in [Statistics::RemoveData]";
255 void Statistics::SetValidRange(
const double minimum,
const double maximum) {
261 QString msg =
"Invalid Range: Minimum [" +
toString(minimum)
262 +
"] must be less than the Maximum [" +
toString(maximum) +
"].";
269 double Statistics::ValidMinimum()
const {
274 double Statistics::ValidMaximum()
const {
279 bool Statistics::InRange(
const double value) {
280 return (!BelowRange(value) && !AboveRange(value));
284 bool Statistics::AboveRange(
const double value) {
289 bool Statistics::BelowRange(
const double value) {
331 if (temp < 0.0) temp = 0.0;
368 if (temp < 0.0) temp = 0.0;
384 QString msg =
"Minimum is invalid since you removed data";
405 QString msg =
"Maximum is invalid since you removed data";
525 bool Statistics::RemovedData()
const {
546 if ((percent <= 0.0) || (percent >= 100.0)) {
547 QString msg =
"Invalid value for percent";
552 double k = sqrt(1.0 / (1.0 - percent / 100.0));
573 if ((percent <= 0.0) || (percent >= 100.0)) {
574 QString msg =
"Invalid value for percent";
579 double k = sqrt(1.0 / (1.0 - percent / 100.0));
651 if (value ==
Maximum())
return 0;
653 QString msg =
"Undefined Z-score. Standard deviation is zero and the input value["
669 m_sum = inStats[
"Sum"];
696 if (name.isEmpty()) {
726 void Statistics::save(QXmlStreamWriter &stream,
const Project *project)
const {
728 stream.writeStartElement(
"statistics");
734 stream.writeStartElement(
"range");
739 stream.writeEndElement();
741 stream.writeStartElement(
"pixelCounts");
751 stream.writeEndElement();
754 stream.writeEndElement();
759 Statistics::XmlHandler::XmlHandler(Statistics *statistics, Project *project) {
760 m_xmlHandlerStatistics = statistics;
761 m_xmlHandlerProject = project;
762 m_xmlHandlerCharacters =
"";
766 Statistics::XmlHandler::~XmlHandler() {
769 m_xmlHandlerProject = NULL;
773 bool Statistics::XmlHandler::startElement(
const QString &namespaceURI,
774 const QString &localName,
775 const QString &qName,
776 const QXmlAttributes &atts) {
777 m_xmlHandlerCharacters =
"";
778 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
785 bool Statistics::XmlHandler::characters(
const QString &ch) {
786 m_xmlHandlerCharacters += ch;
787 return XmlStackedHandler::characters(ch);
791 bool Statistics::XmlHandler::endElement(
const QString &namespaceURI,
const QString &localName,
792 const QString &qName) {
793 if (!m_xmlHandlerCharacters.isEmpty()) {
794 if (localName ==
"id") {
798 if (localName ==
"sum") {
799 m_xmlHandlerStatistics->m_sum =
toDouble(m_xmlHandlerCharacters);
801 if (localName ==
"sumSquares") {
802 m_xmlHandlerStatistics->m_sumsum =
toDouble(m_xmlHandlerCharacters);
804 if (localName ==
"minimum") {
805 m_xmlHandlerStatistics->m_minimum =
toDouble(m_xmlHandlerCharacters);
807 if (localName ==
"maximum") {
808 m_xmlHandlerStatistics->m_maximum =
toDouble(m_xmlHandlerCharacters);
810 if (localName ==
"validMinimum") {
811 m_xmlHandlerStatistics->m_validMinimum =
toDouble(m_xmlHandlerCharacters);
813 if (localName ==
"validMaximum") {
814 m_xmlHandlerStatistics->m_validMaximum =
toDouble(m_xmlHandlerCharacters);
816 if (localName ==
"totalPixels") {
817 m_xmlHandlerStatistics->m_totalPixels =
toBigInt(m_xmlHandlerCharacters);
819 if (localName ==
"validPixels") {
820 m_xmlHandlerStatistics->m_validPixels =
toBigInt(m_xmlHandlerCharacters);
822 if (localName ==
"nullPixels") {
823 m_xmlHandlerStatistics->m_nullPixels =
toBigInt(m_xmlHandlerCharacters);
825 if (localName ==
"lisPixels") {
826 m_xmlHandlerStatistics->m_lisPixels =
toBigInt(m_xmlHandlerCharacters);
828 if (localName ==
"lrsPixels") {
829 m_xmlHandlerStatistics->m_lrsPixels =
toBigInt(m_xmlHandlerCharacters);
831 if (localName ==
"hisPixels") {
832 m_xmlHandlerStatistics->m_hisPixels =
toBigInt(m_xmlHandlerCharacters);
834 if (localName ==
"hrsPixels") {
835 m_xmlHandlerStatistics->m_hrsPixels =
toBigInt(m_xmlHandlerCharacters);
837 if (localName ==
"underRangePixels") {
838 m_xmlHandlerStatistics->m_underRangePixels =
toBigInt(m_xmlHandlerCharacters);
840 if (localName ==
"overRangePixels") {
841 m_xmlHandlerStatistics->m_overRangePixels =
toBigInt(m_xmlHandlerCharacters);
843 if (localName ==
"removedData") {
844 m_xmlHandlerStatistics->m_removedData =
toBool(m_xmlHandlerCharacters);
846 m_xmlHandlerCharacters =
"";
848 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
857 stream.setByteOrder(QDataStream::LittleEndian);
879 QDataStream &Statistics::read(QDataStream &stream) {
882 qint64 totalPixels, validPixels, nullPixels, lrsPixels, lisPixels,
883 hrsPixels, hisPixels, underRangePixels, overRangePixels;
886 stream.setByteOrder(QDataStream::LittleEndian);
925 return statistics.write(stream);
930 return statistics.read(stream);
double SumSquare() const
Returns the sum of all the squared data.
BigInt NullPixels() const
Returns the total number of NULL pixels encountered.
This class is used to accumulate statistics on double arrays.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
double Sum() const
Returns the sum of all the data.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
BigInt TotalPixels() const
Returns the total number of pixels processed (valid and invalid).
A single keyword-value pair.
double m_validMinimum
Minimum valid pixel value.
BigInt m_lrsPixels
Count of low instrument saturation pixels processed.
BigInt m_hisPixels
Count of high instrument representation pixels processed.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
BigInt m_underRangePixels
Count of pixels less than the valid range.
bool IsNullPixel(const double d)
Returns if the input pixel is null.
void fromPvl(const PvlGroup &inStats)
Unserializes a Statistics object from a pvl group.
double Maximum() const
Returns the absolute maximum double found in all data passed through the AddData method.
void Reset()
Reset all accumulators and counters to zero.
BigInt HrsPixels() const
Returns the total number of high representation saturation (HRS) pixels encountered.
bool IsHrsPixel(const double d)
Returns if the input pixel is high representation saturation.
BigInt ValidPixels() const
Returns the total number of valid pixels processed.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
BigInt OverRangePixels() const
Returns the total number of pixels over the valid range encountered.
Manage a stack of content handlers for reading XML files.
double m_sum
The sum accumulator, i.e. the sum of added data values.
BigInt m_totalPixels
Count of total pixels processed.
The main project for ipce.
void RemoveData(const double *data, const unsigned int count)
Remove an array of doubles from the accumulators and counters.
BigInt HisPixels() const
Returns the total number of high instrument saturation (HIS) pixels encountered.
double Variance() const
Computes and returns the variance.
BigInt UnderRangePixels() const
Returns the total number of pixels under the valid range encountered.
BigInt LisPixels() const
Returns the total number of low instrument saturation (LIS) pixels encountered.
double ChebyshevMaximum(const double percent=99.5) const
This method returns a maximum such that X percent of the data will fall with K standard deviations of...
double Rms() const
Computes and returns the rms.
Contains multiple PvlContainers.
BigInt toBigInt(const QString &string)
Global function to convert from a string to a "big" integer.
BigInt m_hrsPixels
Count of high instrument saturation pixels processed.
double StandardDeviation() const
Computes and returns the standard deviation.
double Minimum() const
Returns the absolute minimum double found in all data passed through the AddData method.
double m_sumsum
The sum-squared accumulator, i.e.
long long int BigInt
Big int.
double BestMaximum(const double percent=99.5) const
This method returns the better of the absolute maximum or the Chebyshev maximum.
double m_minimum
Minimum double value encountered.
double ZScore(const double value) const
This method returns the better of the z-score of the given value.
BigInt m_lisPixels
Count of low representation saturation pixels processed.
double m_validMaximum
Maximum valid pixel value.
double m_maximum
Maximum double value encountered.
bool IsLisPixel(const double d)
Returns if the input pixel is low instrument saturation.
BigInt OutOfRangePixels() const
Returns the total number of pixels outside of the valid range encountered.
PvlGroup toPvl(QString name="Statistics") const
Serialize statistics as a pvl group.
bool IsLrsPixel(const double d)
Returns if the input pixel is low representation saturation.
double Average() const
Computes and returns the average.
bool IsHisPixel(const double d)
Returns if the input pixel is high instrument saturation.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
double BestMinimum(const double percent=99.5) const
This method returns the better of the absolute minimum or the Chebyshev minimum.
BigInt m_validPixels
Count of valid pixels (non-special) processed.
Statistics(QObject *parent=0)
Constructs an IsisStats object with accumulators and counters set to zero.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
QDataStream & write(QDataStream &stream) const
Order saved must match the offsets in the static compoundH5DataType() method.
double ChebyshevMinimum(const double percent=99.5) const
This method returns a minimum such that X percent of the data will fall with K standard deviations of...
virtual ~Statistics()
Destroys the IsisStats object.
BigInt m_nullPixels
Count of null pixels processed.
BigInt LrsPixels() const
Returns the total number of low representation saturation (LRS) pixels encountered.
std::istream & operator>>(std::istream &is, CSVReader &csv)
Input read operator for input stream sources.
bool m_removedData
Indicates the RemoveData method was called which implies m_minimum and m_maximum are invalid.
This is free and unencumbered software released into the public domain.
BigInt m_overRangePixels
Count of pixels greater than the valid range.