File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
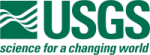 |
Isis 3 Programmer Reference
|
12 #include <QToolButton>
14 #include "GuiParameter.h"
16 #include "Application.h"
18 #include "GuiHelperAction.h"
19 #include "UserInterface.h"
21 #include "GuiFilenameParameter.h"
22 #include "GuiCubeParameter.h"
27 int group,
int param) :
QObject() {
33 p_fileButton =
new QToolButton();
34 p_lineEdit =
new QLineEdit();
36 p_label =
new QLabel(p_name);
37 p_label->setAlignment(Qt::AlignRight | Qt::AlignVCenter);
38 p_label->setToolTip(p_ui->
ParamBrief(group, param));
39 grid->addWidget(p_label, param, 0, Qt::AlignTop);
42 whatsThis = (QString)(
"<b>Parameter:</b> " + p_ui->
ParamName(group, param));
43 whatsThis += (QString)(
"<p><b>Type:</b> " + p_ui->
ParamType(group, param) +
"</p>");
44 whatsThis += (QString)(
"<p><b>Brief:</b> " + p_ui->
ParamBrief(group, param) +
"</p>");
46 if(def ==
"") def =
"None";
47 whatsThis +=
"<p><b>Default: </b>" + QString(def) +
"</p>";
50 whatsThis +=
"<p><b>Internal Default: </b> " + QString(intdef) +
"</p>";
52 QString pixtype = p_ui->
PixelType(group, param);
54 whatsThis +=
"<p><b>Pixel Type: </b> " + QString(pixtype) +
"</p>";
59 whatsThis +=
"<p><b>Greater Than Or Equal To: </b>" +
60 QString(pmin) +
"</p>";
63 whatsThis +=
"<p><b>Greater Than: </b>" + QString(pmin) +
"</p>";
69 whatsThis +=
"<p><b>Less Than Or Equal To: </b>" +
70 QString(pmax) +
"</p>";
73 whatsThis +=
"<p><b>Less Than: </b>" + QString(pmax) +
"</p>";
77 whatsThis +=
"<p><b>Less Than: </b>" +
80 whatsThis +=
", " + QString(p_ui->
ParamLessThan(group, param, l));
85 whatsThis +=
"<p><b>Less Than Or Equal: </b>" +
94 whatsThis +=
"<p><b>Not Equal: </b>" +
97 whatsThis +=
", " + QString(p_ui->
ParamNotEqual(group, param, l));
102 whatsThis +=
"<p><b>Greater Than: </b>" +
111 whatsThis +=
"<p><b>Greater Than Or Equal: </b>" +
120 whatsThis +=
"<p><b>Inclusions: </b>" +
129 whatsThis +=
"<p><b>Exclusions: </b>" +
137 if(p_ui->
ParamOdd(group, param) !=
"") {
138 whatsThis +=
"<p><b>Odd: </b>" +
139 QString(p_ui->
ParamOdd(group, param)) +
"</p>";
141 p_label->setWhatsThis(whatsThis);
154 p_widgetList.clear();
184 if(Value() == p_ui->
ParamDefault(p_group, p_param))
return false;
190 if(Value() ==
"")
return false;
200 else if(p_ui->
ParamDefault(p_group, p_param).size() > 0) {
213 p_widgetList.push_back(w);
218 if(p_type != ComboWidget) {
219 p_label->setEnabled(enabled);
220 p_label->setVisible(
true);
221 if(isParentCombo && !enabled) {
222 p_label->setVisible(
false);
224 for(
int i = 0; i < p_widgetList.size(); i++) {
225 p_widgetList[i]->setEnabled(enabled);
226 p_widgetList[i]->setVisible(
true);
227 if(isParentCombo && !enabled) {
228 p_widgetList[i]->setVisible(
false);
233 p_label->setEnabled(enabled);
234 p_widgetList[0]->setEnabled(enabled);
240 std::vector<QString> list;
250 if(p_ui->
HelperIcon(p_group, p_param, 0) !=
"") {
253 action->setIcon(QIcon(QPixmap(file)));
258 action->setToolTip(p_ui->
HelperBrief(p_group, p_param, 0));
259 QString helperText =
"<p><b>Function:</b> " +
261 action->setWhatsThis(helperText);
262 connect(action, SIGNAL(trigger(
const QString &)),
this,
263 SIGNAL(HelperTrigger(
const QString &)));
265 QToolButton *helper =
new QToolButton();
267 helper->setDefaultAction(action);
269 if(p_ui->
HelperIcon(p_group, p_param, 0) !=
"") {
273 helper->setIconSize(QSize(22, 22));
274 helper->setIcon(QIcon(QPixmap(file)));
277 helper->setFixedWidth(helper->fontMetrics().width(
278 " " + helper->text() +
" "));
288 "Can not call GuiParameter::AddHelpers twice",
292 p_helperMenu =
new QMenu();
297 if(p_ui->
HelperIcon(p_group, p_param, 0) !=
"") {
300 action->setIcon(QIcon(QPixmap(file)));
305 connect(action, SIGNAL(trigger(
const QString &)),
this,
306 SIGNAL(HelperTrigger(
const QString &)));
309 QToolButton *helper =
new QToolButton();
312 helper->setMenu(p_helperMenu);
313 helper->setPopupMode(QToolButton::MenuButtonPopup);
314 helper->setDefaultAction(action);
315 helper->setToolTip(p_ui->
HelperBrief(p_group, p_param, 0));
316 QString text =
"<p><b>Function:</b> " +
318 "<p><b>Hint: </b> Click on the arrow to see more helper functions</p>";
319 helper->setWhatsThis(text);
321 if(p_ui->
HelperIcon(p_group, p_param, 0) !=
"") {
325 helper->setIconSize(QSize(22, 22));
326 helper->setIcon(QIcon(QPixmap(file)));
329 helper->setFixedWidth(helper->fontMetrics().width(
330 " " + helper->text() +
" "));
336 action2->setText(p_ui->
HelperBrief(p_group, p_param, 0));
337 action2->setToolTip(p_ui->
HelperBrief(p_group, p_param, 0));
338 QString helperText =
"<p><b>Function:</b> " +
340 action2->setWhatsThis(helperText);
341 connect(action2, SIGNAL(trigger(
const QString &)),
this,
342 SIGNAL(HelperTrigger(
const QString &)));
343 p_helperMenu->addAction(action2);
347 for(
int i = 1; i < p_ui->
HelpersSize(p_group, p_param); i++) {
350 helperAction->setText(p_ui->
HelperBrief(p_group, p_param, i));
351 helperAction->setToolTip(p_ui->
HelperBrief(p_group, p_param, i));
352 QString helperText =
"<p><b>Function:</b> " +
354 helperAction->setWhatsThis(helperText);
355 connect(helperAction, SIGNAL(trigger(
const QString &)),
this,
356 SIGNAL(HelperTrigger(
const QString &)));
357 p_helperMenu->addAction(helperAction);
void RememberWidget(QWidget *w)
Add widgets to a list for enabling/disabling.
QString HelperIcon(const int &group, const int ¶m, const int &helper) const
Returns the name of the icon for the helper button.
void Update()
Update the value on the GUI with the value in the UI.
void SetToDefault()
Change the parameter to the default value.
QString HelperDescription(const int &group, const int ¶m, const int &helper) const
Returns the long description of the helper button.
bool IsEnabled() const
Is the parameter enabled.
GuiParameter(QGridLayout *grid, UserInterface &ui, int group, int param)
Constructor.
QString GetAsString(const QString ¶mName) const
Allows the retrieval of a value for a parameter of any type.
QString ParamMinimumInclusive(const int &group, const int ¶m) const
Returns whether the minimum value is inclusive or not.
virtual ~GuiParameter()
Destructor.
File name manipulation and expansion.
QString ParamBrief(const int &group, const int ¶m) const
Returns the brief description of a parameter in a specified group.
QString ParamGreaterThanOrEqual(const int &group, const int ¶m, const int &great) const
Returns the name of the specified greaterThanOrEqual parameter.
int HelpersSize(const int &group, const int ¶m) const
Returns the number of helpers the parameter has.
void SetEnabled(bool enabled, bool isParentCombo=false)
Enable or disable the parameter.
QString ParamMaximum(const int &group, const int ¶m) const
Returns the maximum value of a parameter in a specified group.
QString HelperFunction(const int &group, const int ¶m, const int &helper) const
Returns the name of the helper function.
QString ParamGreaterThan(const int &group, const int ¶m, const int &great) const
Returns the name of the specified greaterThan parameter.
virtual bool IsModified()
Return if the parameter value is different from the default value.
QString ParamDefault(const int &group, const int ¶m) const
Returns the default for a parameter in a specified group.
int ParamIncludeSize(const int &group, const int ¶m) const
Returns the number of parameters included in this parameter's inclusions.
QString ParamType(const int &group, const int ¶m) const
Returns the parameter type of a parameter in a specified group.
QString ParamInternalDefault(const int &group, const int ¶m) const
Returns the internal default for a parameter in a specified group.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
int ParamNotEqualSize(const int &group, const int ¶m) const
Returns the number of values in the not equal list.
QString HelperBrief(const int &group, const int ¶m, const int &helper) const
Returns the brief description of the helper button.
virtual std::vector< QString > Exclusions()
Return list of current exclusions.
QString ParamExclude(const int &group, const int ¶m, const int &exclude) const
Returns the name of the specified excluded parameter.
QString ParamNotEqual(const int &group, const int ¶m, const int ¬Eq) const
Returns the name of the specified notEqual parameter.
QString ParamMinimum(const int &group, const int ¶m) const
Returns the minimum value of a parameter in a specified group.
int ParamExcludeSize(const int &group, const int ¶m) const
Returns the number of parameters excluded in this parameter's exclusions.
QString ParamInclude(const int &group, const int ¶m, const int &include) const
Returns the name of the specified included parameter.
void SetToCurrent()
Change the parameter to the current user interface value.
QString PixelType(const int &group, const int ¶m) const
Returns the default pixel type from the XML.
int ParamGreaterThanOrEqualSize(const int &group, const int ¶m) const
Returns the number of values in the parameters greater than or equal list.
int ParamGreaterThanSize(const int &group, const int ¶m) const
Returns the number of values in the parameters greater than list.
QString ParamLessThan(const int &group, const int ¶m, const int &great) const
Returns the name of the specified lessThan parameter.
bool WasEntered(const QString ¶mName) const
Returns a true if the parameter has a value, and false if it does not.
int ParamLessThanSize(const int &group, const int ¶m) const
Returns the number of values in the parameters less than list.
@ Programmer
This error is for when a programmer made an API call that was illegal.
int ParamLessThanOrEqualSize(const int &group, const int ¶m) const
Returns the number of values in the parameters less than or equal list.
Command Line and Xml loader, validation, and access.
QString HelperButtonName(const int &group, const int ¶m, const int &helper) const
Returns the name of the helper button.
QString ParamLessThanOrEqual(const int &group, const int ¶m, const int &les) const
Returns the name of the specified lessThanOrEqual parameter.
QString ParamMaximumInclusive(const int &group, const int ¶m) const
Returns whether the maximum value is inclusive or not.
QString ParamOdd(const int &group, const int ¶m) const
Returns whether the selected parameter has a restriction on odd values or not.
QString ParamName(const int &group, const int ¶m) const
Returns the parameter name.
This is free and unencumbered software released into the public domain.
QWidget * AddHelpers(QObject *lo)
Sets up helper button.