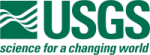 |
Isis 3 Programmer Reference
|
7 #include "InlineCalculator.h"
15 #include <QStringList>
20 #include <boost/foreach.hpp>
28 #include "IException.h"
29 #include "InlineInfixToPostfix.h"
31 #include "NaifStatus.h"
110 tokenOps = tokenOps.simplified();
113 QString error =
"Errors parsing inline equation[" +
equation +
"].";
121 while ( !tokenList.isEmpty() ) {
122 QString token = tokenList.takeFirst();
123 if ( !token.isEmpty() ) {
149 error =
"Equation element (" + token +
") invalid - token not recognized.";
167 return (nerrors == 0);
197 "Calculation with variable pool failed.",
225 QString msg =
"Too many operands in the equation [" +
m_equation +
"].";
256 if (
scalar.isEmpty())
return (
false);
312 if (variablePool->
exists(key)) {
319 QString error =
"Could not find variable [" + key +
"] in variable pool.";
363 BOOST_FOREACH(
double d, degree) {
364 result.push_back(d * rpd_c());
378 BOOST_FOREACH(
double r,
radians) {
379 result.push_back(r * dpr_c());
397 if ( inputA.size() != inputB.size() ) {
398 QString msg =
"Failed performing logical or operation, "
399 "input vectors are of differnet lengths.";
402 for (
int i = 0; i < inputA.size(); i++) {
403 results.push_back( inputA[i] || inputB[i] );
421 if ( inputA.size() != inputB.size() ) {
422 QString msg =
"Failed performing logical and operation, "
423 "input vectors are of differnet lengths.";
426 for (
int i = 0; i < inputA.size(); i++) {
427 results.push_back( inputA[i] && inputB[i] );
481 m_fxPool.insert(function->name(),
function);
484 QString msg =
"Function operator [" +
function->name() +
485 "] exists! Cannot replace existing functions in the pool :-(";
541 "Request for nonexistent variable pool.",
569 FxPoolType::iterator fx =
m_fxPool.find(fxname);
570 if (
m_fxPool.end() == fx )
return NULL;
692 const int &index)
const {
693 QString mess =
"No implementation in Calculator variable pool to provide "
694 " value for variable [" + variable +
"].";
712 QString mess =
"No implementation in Calculator variable pool to add "
713 " value for variable [" + key +
"].";
769 return (QVariant(
m_name));
virtual QVariant args()
Accesses the arguments for this function.
void Push(double scalar)
Push a scalar onto the stack.
FxPoolType m_fxPool
The map between function names and equation lists.
void Arcsine()
Pops one element and push the arcsine.
QString m_name
Name of function.
void Cosine()
Pops one element and push the cosine.
FxTypePtr find(const QString &fxname)
Gets a pointer to the function from the current pool that corresponds to the given function name.
InlineCalculator * m_calc
The Calculator used to evaluate this function.
void Tangent()
Pops one element and push the tangent.
calcOp m_func
The InlineCalculator operator that takes parameters.
virtual ~FxBinder()
Destroys the FxBinder object.
QString m_equation
The equation to be evaluated.
void Add()
Pops two elements, adds them, then pushes the sum on the stack.
void initialize()
Adds the recognized functions to the function pool.
void Cosecant()
Pops one element and push the cosecant.
void Or()
Pop two elements, OR them, then push the result on the stack.
void destruct()
Discard of all the function pool and class resources.
FxBinder(const QString &name)
Constructs a function binder given a name.
void popVariables()
Removes the last variable pool in the current variable pool list.
void Multiply()
Pops two elements, multiplies them, then pushes the product on the stack.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void Modulus()
Pops two elements, mods them, then pushes the result on the stack.
void Equal()
Pop two elements off the stack and compare them to see where one is equal to the other,...
QString convert(const QString &infix)
This method converts infix to postfix.
virtual ~ParameterFx()
Destroys the ParameterFx object.
void Arctangent()
Pops one element and push the arctangent.
calcOp m_func
The InlineCalculator operator that takes no parameters.
void logicalOr()
Pops the top two vectors off the current stack and performs a logical or on each pair.
virtual ~InlineCalculator()
Destroys the InlineCalculator object.
QString equation() const
Accesses the string representation of the current equation, in postfix format.
void variable(const QVariant &variable)
Pushes the given value onto the stack as a variable.
virtual bool orphanTokenHandler(const QString &token)
Default token handler if it is undefined during parsing/compilation.
void eConstant()
Pushes the Euler constant (e) onto the current stack.
This class is used to bind function names with corresponding Calculator functions that take a paramet...
void Sine()
Pops one element and push the sine.
void Divide()
Pops two, divides them, then pushes the quotient on the stack.
ParameterFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs a Parameter function from the given name (containing the appropriate parameters),...
~CalculatorVariablePool()
Destroys the CalculatorVariablePool object.
virtual void dispatch()=0
This method defines how to execute this function.
void floatModulus()
Pops the top two vectors off the current stack and performs the floatModulusOperator() on the corresp...
void Subtract()
Pops two elements, subtracts them, then pushes the difference on the stack.
void append(const IException &exceptionSource)
Appends the given exception (and its list of previous exceptions) to this exception's causational exc...
void radians()
Pops the top vector off the current stack and converts from degrees to radians.
double floatModulusOperator(double a, double b)
Determines the remainder of the quotient a/b whose sign is the same as that of a.
void pushVariables(CalculatorVariablePool *variablePool)
Push the given variable pool onto the current variable pool list.
virtual ~InlineVoidFx()
Destroys the InlineVoidFx object.
void AbsoluteValue()
Pop an element, compute its absolute value, then push the result on the stack.
QVector< double > evaluate()
Evaluate compiled equation with existing variable pool.
CalculatorVariablePool()
Constructs a CalculatorVariablePool object.
void dispatch()
Calls the function corresponding to this object using its stored InlineCalculator and InlineCalculato...
QList< CalculatorVariablePool * > m_variablePoolList
The list of variable pool pointers.
A parser for converting equation strings to postfix.
void Cotangent()
Pops one element and push the cotangent.
void Log10()
Pop an element, compute its base 10 log, then push the result on the stack.
calcOp m_func
The Calculator operator that takes no parameters.
Provides a calculator for inline equations.
int size() const
Accesses the number of functions, operators, variables, and scalars to be executed.
virtual void add(const QString &key, QVector< double > &values)
Add a parameter to the variable pool.
void degrees()
Pops the top vector off the current stack and converts from radians to degrees.
virtual QVector< double > value(const QString &variable, const int &index=0) const
Return vector of doubles for Calculator functions.
void SquareRoot()
Pop an element, compute its square root, then push the root on the stack.
void dispatch()
Calls the function corresponding to this object using its stored Calculator and Calculator operator.
void RightShift()
Pop the top element, then perform a right shift with zero fill.
bool isVariable(const QString &str)
Determines whether the given string is a variable.
bool fxExists(const QString &fxname) const
Determines whether the given function name exists in the current function pool.
void Arccosine()
Pops one element and push the arccosine.
FxEqList m_functions
The list of pointers to function equations for the calculator.
void LeftShift()
Pop the top element, then perform a left shift with zero fill.
This is a simple class to model a Calculator Variable Pool.
InlineCalculator * m_calc
The InlineCalculator used to evaluate this function.
void GreaterThanOrEqual()
Pop two elements off the stack and compare them to see where one is greater than or equal to the othe...
InlineVoidFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs an InlineVoid function from the given name, InlineCalculator operator, and InlineCalculato...
CalculatorVariablePool * variables()
Accesses the last variable pool in the current pool list.
void LessThan()
Pop two elements off the stack and compare them to see where one is less than the other,...
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
QVector< double > Pop(bool keepSpecials=false)
Pop an element off the stack.
FxTypePtr addFunction(FxTypePtr function)
Adds a function to the function pool.
void GreaterThan()
Pop two elements off the stack and compare them to see where one is greater than the other,...
This class is used to bind function names with corresponding InlineCalculator functions that do not t...
void dispatch()
Calls the function corresponding to this object using its stored InlineCalculator,...
void PerformOperation(QVector< double > &results, QVector< double >::iterator arg1Start, QVector< double >::iterator arg1End, double operation(double))
Performs the mathematical operations on each argument.
void LessThanOrEqual()
Pop two elements off the stack and compare them to see where one is less than or equal to the other,...
virtual bool exists(const QString &variable) const
Returns true so the real error can be reported.
const double E
Sets some basic constants for use in ISIS programming.
bool isScalar(const QString &scalar)
Determines whether the given string contains a scalar value (i.e.
void Arctangent2()
Pops two elements and push the arctangent.
virtual QString toPostfix(const QString &equation) const
Converts the given string from infix to postfix format.
virtual ~VoidFx()
Destroys the VoidFx object.
InlineCalculator * m_calc
The InlineCalculator used to evaluate this function.
void execute()
Executes the function.
void operator()()
Executes the function.
void Exponent()
Pops two elements, computes the power then pushes the result on the stack The exponent has to be a sc...
int StackSize()
Returns the current stack size.
virtual void Clear()
Clear out the stack.
void Log()
Pop an element, compute its log, then push the result on the stack.
void Secant()
Pops one element and push the secant.
This class is used to bind function names with corresponding Calculator functions that do not take pa...
InlineCalculator()
Constructs an InlineCalculator object by initializing the operator lookup list.
bool compile(const QString &equation)
Compiles the given equation for evaluation.
void MaximumPixel()
Pop two elements, then push the maximum on a pixel by pixel basis back on the stack.
QString name() const
The name assigned to this function binder.
VoidFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs a Void function from the given name, Calculator operator, and Calculator.
void pi()
Pushes the PI constant onto the current stack.
void logicalAnd()
Pops the top two vectors off the current stack and performs a logical and on each pair.
This is free and unencumbered software released into the public domain.
void NotEqual()
Pop two elements off the stack and compare them to see where one is not equal to the other,...
void MinimumPixel()
Pop two elements, then push the minimum on a pixel by pixel basis back on the stack.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
void Negative()
Pops an element, negates it, then pushes the result.
void scalar(const QVariant &scalar)
Pushes the given value onto the stack as a scalar.
void And()
Pop two elements, AND them, then push the result on the stack.
This is the parent class to the various function classes.