File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
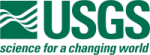 |
Isis 3 Programmer Reference
|
1 #ifndef InlineCalculator_h
2 #define InlineCalculator_h
11 #include "Calculator.h"
22 class CalculatorVariablePool;
31 #define CALL_MEMBER_FN(object, ptrToMember) ((object).*(ptrToMember))
87 bool fxExists(
const QString &fxname)
const;
126 virtual bool exists(
const QString &variable)
const;
128 const int &index = 0)
const;
145 QString
name()
const;
154 virtual QVariant
args();
virtual QVariant args()
Accesses the arguments for this function.
FxPoolType m_fxPool
The map between function names and equation lists.
QString m_name
Name of function.
FxTypePtr find(const QString &fxname)
Gets a pointer to the function from the current pool that corresponds to the given function name.
InlineCalculator * m_calc
The Calculator used to evaluate this function.
calcOp m_func
The InlineCalculator operator that takes parameters.
virtual ~FxBinder()
Destroys the FxBinder object.
QString m_equation
The equation to be evaluated.
void initialize()
Adds the recognized functions to the function pool.
This is free and unencumbered software released into the public domain.
void destruct()
Discard of all the function pool and class resources.
FxBinder(const QString &name)
Constructs a function binder given a name.
void(InlineCalculator::* calcOp)(const QVariant &arg)
Defines an InlineCalculator function that takes arguments.
void popVariables()
Removes the last variable pool in the current variable pool list.
virtual ~ParameterFx()
Destroys the ParameterFx object.
void(InlineCalculator::* calcOp)()
Defines an InlineCalculator function that takes no arguments.
void(Calculator::* calcOp)()
Defines a Calculator function that takes no arguments.
calcOp m_func
The InlineCalculator operator that takes no parameters.
void logicalOr()
Pops the top two vectors off the current stack and performs a logical or on each pair.
virtual ~InlineCalculator()
Destroys the InlineCalculator object.
QString equation() const
Accesses the string representation of the current equation, in postfix format.
void variable(const QVariant &variable)
Pushes the given value onto the stack as a variable.
virtual bool orphanTokenHandler(const QString &token)
Default token handler if it is undefined during parsing/compilation.
void eConstant()
Pushes the Euler constant (e) onto the current stack.
This class is used to bind function names with corresponding Calculator functions that take a paramet...
FxBinder * FxTypePtr
Defintion for a FxTypePtr, a pointer to a function binder (FxBinder)
ParameterFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs a Parameter function from the given name (containing the appropriate parameters),...
~CalculatorVariablePool()
Destroys the CalculatorVariablePool object.
virtual void dispatch()=0
This method defines how to execute this function.
void floatModulus()
Pops the top two vectors off the current stack and performs the floatModulusOperator() on the corresp...
void radians()
Pops the top vector off the current stack and converts from degrees to radians.
double floatModulusOperator(double a, double b)
Determines the remainder of the quotient a/b whose sign is the same as that of a.
void pushVariables(CalculatorVariablePool *variablePool)
Push the given variable pool onto the current variable pool list.
virtual ~InlineVoidFx()
Destroys the InlineVoidFx object.
QVector< double > evaluate()
Evaluate compiled equation with existing variable pool.
CalculatorVariablePool()
Constructs a CalculatorVariablePool object.
void dispatch()
Calls the function corresponding to this object using its stored InlineCalculator and InlineCalculato...
QVector< FxTypePtr > FxEqList
Definition for a FxEqList, a vector of function type pointers.
QList< CalculatorVariablePool * > m_variablePoolList
The list of variable pool pointers.
calcOp m_func
The Calculator operator that takes no parameters.
Provides a calculator for inline equations.
int size() const
Accesses the number of functions, operators, variables, and scalars to be executed.
virtual void add(const QString &key, QVector< double > &values)
Add a parameter to the variable pool.
void degrees()
Pops the top vector off the current stack and converts from radians to degrees.
virtual QVector< double > value(const QString &variable, const int &index=0) const
Return vector of doubles for Calculator functions.
void dispatch()
Calls the function corresponding to this object using its stored Calculator and Calculator operator.
bool isVariable(const QString &str)
Determines whether the given string is a variable.
bool fxExists(const QString &fxname) const
Determines whether the given function name exists in the current function pool.
FxEqList m_functions
The list of pointers to function equations for the calculator.
This is a simple class to model a Calculator Variable Pool.
InlineCalculator * m_calc
The InlineCalculator used to evaluate this function.
InlineVoidFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs an InlineVoid function from the given name, InlineCalculator operator, and InlineCalculato...
CalculatorVariablePool * variables()
Accesses the last variable pool in the current pool list.
FxTypePtr addFunction(FxTypePtr function)
Adds a function to the function pool.
This class is used to bind function names with corresponding InlineCalculator functions that do not t...
void dispatch()
Calls the function corresponding to this object using its stored InlineCalculator,...
virtual bool exists(const QString &variable) const
Returns true so the real error can be reported.
bool isScalar(const QString &scalar)
Determines whether the given string contains a scalar value (i.e.
virtual QString toPostfix(const QString &equation) const
Converts the given string from infix to postfix format.
virtual ~VoidFx()
Destroys the VoidFx object.
InlineCalculator * m_calc
The InlineCalculator used to evaluate this function.
void execute()
Executes the function.
void operator()()
Executes the function.
This class is used to bind function names with corresponding Calculator functions that do not take pa...
InlineCalculator()
Constructs an InlineCalculator object by initializing the operator lookup list.
bool compile(const QString &equation)
Compiles the given equation for evaluation.
QString name() const
The name assigned to this function binder.
VoidFx(const QString &name, calcOp function, InlineCalculator *calculator)
Constructs a Void function from the given name, Calculator operator, and Calculator.
void pi()
Pushes the PI constant onto the current stack.
QMap< QString, FxTypePtr > FxPoolType
Definition for a FxPoolType, a map between a string and function type pointer.
void logicalAnd()
Pops the top two vectors off the current stack and performs a logical and on each pair.
This is free and unencumbered software released into the public domain.
void scalar(const QVariant &scalar)
Pushes the given value onto the stack as a scalar.
This is the parent class to the various function classes.