File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
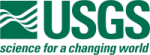 |
Isis 3 Programmer Reference
|
17 #include "AbstractPlate.h"
18 #include "IException.h"
20 #include "NaifDskApi.h"
22 #include "Longitude.h"
23 #include "SurfacePoint.h"
30 Intercept::Intercept() : m_observer(), m_raydir(), m_point(0), m_shape(0) { }
52 m_observer(observer.copy()), m_raydir(raydir.copy()),
53 m_point(ipoint), m_shape(shape) {}
74 if (
m_point.isNull() ) valid =
false;
75 if (
m_shape.isNull() ) valid =
false;
117 verify(
isValid(),
"Unable to return Intercept location. Invalid/undefined Intercept point.");
130 verify(
isValid(),
"Unable to return Intercept normal. Invalid/undefined Intercept point.");
152 "Unable to return Intercept emission angle. Invalid/undefined Intercept point." );
156 m_point->ToNaifArray(&point[0]);
159 vsub_c(&
m_observer[0], &point[0], &raydir[0]);
184 "Unable to return Intercept separation angle. Invalid/undefined Intercept point.");
185 return (
m_shape->separationAngle(raydir));
207 if ( (
Throw == action ) && ( !test ) ) {
Angle separationAngle(const NaifVector &raydir) const
Returns the separation angle of the observer and the plate normal.
TNT::Array1D< SpiceDouble > NaifVertex
1-D Buffer[3]
SurfacePoint location() const
Returns the location of the intercept location on the shape.
@ Throw
Throw an exception if an error occurs.
NaifVector normal() const
Gets the normal vector to the shape for this plate.
Angle emission() const
Compute the emission of the intercept point from the observer.
NaifVertex m_observer
Three dimensional coordinate position of the observer, in body fixed.
Abstract interface to a TIN plate.
virtual ~Intercept()
Empty destructor.
const NaifVector & lookDirectionRay() const
Accessor for the look direction of the intercept.
QSharedPointer< SurfacePoint > m_point
Surface point of the intercept location on the body, in body fixed.
Defines an angle and provides unit conversions.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
ErrAction
Enumeration to indicate whether to throw an exception if an error occurs.
const NaifVertex & observer() const
Accessor for the observer position of the intercept.
bool verify(const bool &test, const QString &errmsg, const ErrAction &action=Throw) const
Convenient error handler.
bool isValid() const
This method tests the vailidty of the intercept point.
TNT::Array1D< SpiceDouble > NaifVector
Namespace to contain type definitions of NAIF DSK fundamentals.
bool validate(const NaifVertex &v)
Verifies that the given NaifVector or NaifVertex is 3 dimensional.
NaifVector m_raydir
Three dimensional ray representing the look direction.
Intercept()
Default empty constructor.
This class defines a body-fixed surface point.
const AbstractPlate * shape() const
Access the plate for this intercept.
This is free and unencumbered software released into the public domain.
QSharedPointer< AbstractPlate > m_shape
Shape Model for the intercept point.