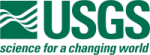 |
Isis 3 Programmer Reference
|
11 #include "ImageImporter.h"
12 #include "PixelType.h"
36 virtual bool isRgb()
const;
37 virtual bool isArgb()
const;
41 virtual int getPixel(
int s,
int l)
const;
43 virtual int getGray(
int pixel)
const;
44 virtual int getRed(
int pixel)
const;
45 virtual int getGreen(
int pixel)
const;
46 virtual int getBlue(
int pixel)
const;
47 virtual int getAlpha(
int pixel)
const;
virtual int getGreen(int pixel) const
Retrieves the green component of the given pixel from the second band of the input buffer.
virtual bool isArgb() const
Tests to see if the input image is quadruple-banded, implying RGBA.
File name manipulation and expansion.
virtual int getAlpha(int pixel) const
Retrieves the alpha component of the given pixel from the fourth band of the input buffer.
char ** m_buffer
Buffer that stores a line of JPEG 2000 data and all its color bands.
Imports JPEG 2000 images as Isis cubes.
Imports images with standard formats into Isis as cubes.
JP2Importer(FileName inputName)
Construct the importer.
virtual int getRed(int pixel) const
Retrieves the red component of the given pixel from the first band of the input buffer.
JP2Decoder * m_decoder
Takes a raw stream of JPEG 2000 data and reads it into a buffer.
virtual int getPixel(int s, int l) const
Returns a representation of a pixel for the input format that can then be broken down into specific g...
virtual int getGray(int pixel) const
Retrieves the gray component of the given pixel.
virtual int getBlue(int pixel) const
Retrieves the blue component of the given pixel from the third band of the input buffer.
PixelType
Enumerations for Isis Pixel Types.
Isis::PixelType m_pixelType
Pixel type of the input image needed for reading data into the buffer.
virtual ~JP2Importer()
Destruct the importer.
virtual void updateRawBuffer(int line, int band) const
Updates the buffer used to store chunks of the input data at a time.
virtual bool isGrayscale() const
Tests to see if the input image is single-banded, implying grayscale (no RGB/A).
int getFromBuffer(int s, int b) const
Retrieves the pixel value from the input buffer corresponding to the given sample and band (the buffe...
virtual bool isRgb() const
Tests to see if the input image is triple-banded, implying RGB (no alpha).
This is free and unencumbered software released into the public domain.