File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
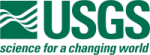 |
Isis 3 Programmer Reference
|
7 #include "JP2Importer.h"
10 #include "IException.h"
11 #include "JP2Decoder.h"
12 #include "ProcessImport.h"
35 int pixelBytes =
m_decoder->GetPixelBytes();
36 if (pixelBytes == 1) {
39 else if (pixelBytes == 2) {
40 bool signedData =
m_decoder->GetSignedData();
41 m_pixelType = signedData ? Isis::SignedWord : Isis::UnsignedWord;
45 "The file [" +
filename().expanded() +
46 "] contains unsupported data type",
54 for (
int i = 0; i <
bands(); i++)
m_buffer[i] =
new char [readBytes];
58 "The file [" + inputName.
expanded() +
59 "] cannot be opened as a JPEG 2000 file",
84 return m_decoder->GetBandDimension() == 1;
94 return m_decoder->GetBandDimension() == 3;
104 return m_decoder->GetBandDimension() == 4;
229 case Isis::UnsignedByte:
230 value = (int) ((
unsigned char *)
m_buffer[b])[s];
232 case Isis::UnsignedWord:
233 value = (int) ((
unsigned short int *)
m_buffer[b])[s];
235 case Isis::SignedWord:
236 value = (int) ((
short int *)
m_buffer[b])[s];
int SizeOf(Isis::PixelType pixelType)
Returns the number of bytes of the specified PixelType.
virtual int getGreen(int pixel) const
Retrieves the green component of the given pixel from the second band of the input buffer.
virtual int convertRgbToGray(int pixel) const
Convert the current pixel, taken from an RGB/A image, and blend its RGB components into a single gray...
void setSamples(int s)
Set the sample dimension (width) of the output image.
void OpenFile()
Open the JPEG2000 file.
virtual bool isArgb() const
Tests to see if the input image is quadruple-banded, implying RGBA.
File name manipulation and expansion.
void Read(unsigned char **inbuf)
Read data from JP2 file containing 8-bit data.
virtual int getAlpha(int pixel) const
Retrieves the alpha component of the given pixel from the fourth band of the input buffer.
char ** m_buffer
Buffer that stores a line of JPEG 2000 data and all its color bands.
Imports images with standard formats into Isis as cubes.
JP2Importer(FileName inputName)
Construct the importer.
virtual int getRed(int pixel) const
Retrieves the red component of the given pixel from the first band of the input buffer.
int bands() const
The band dimension (depth) of the output image.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
int samples() const
The sample dimension (width) of the output image.
JP2Decoder * m_decoder
Takes a raw stream of JPEG 2000 data and reads it into a buffer.
virtual int getPixel(int s, int l) const
Returns a representation of a pixel for the input format that can then be broken down into specific g...
void setBands(int b)
Set the band dimension (depth) of the output image.
virtual int getGray(int pixel) const
Retrieves the gray component of the given pixel.
void setLines(int l)
Set the line dimension (height) of the output image.
virtual int getBlue(int pixel) const
Retrieves the blue component of the given pixel from the third band of the input buffer.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Isis::PixelType m_pixelType
Pixel type of the input image needed for reading data into the buffer.
FileName filename() const
The filename of the input image this instance was constructed with.
virtual ~JP2Importer()
Destruct the importer.
virtual void updateRawBuffer(int line, int band) const
Updates the buffer used to store chunks of the input data at a time.
Adds specific functionality to C++ strings.
virtual bool isGrayscale() const
Tests to see if the input image is single-banded, implying grayscale (no RGB/A).
int getFromBuffer(int s, int b) const
Retrieves the pixel value from the input buffer corresponding to the given sample and band (the buffe...
virtual bool isRgb() const
Tests to see if the input image is triple-banded, implying RGB (no alpha).
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....