File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
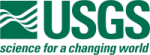 |
Isis 3 Programmer Reference
|
18 #include <QStringList>
104 KernelDb(
const unsigned int allowedKernelTypes);
105 KernelDb(
const QString &dbName,
const unsigned int allowedKernelTypes);
106 KernelDb(std::istream &dbStream,
const unsigned int allowedKernelTypes);
131 iTime timeToMatch,
int cameraVersion);
Kernel targetAttitudeShape(Pvl &lab)
This method finds the highest version of all Target Attitude Shape kernels (pck) identified by the da...
KernelDb(const unsigned int allowedKernelTypes)
Constructs a new KernelDb object with a given integer value representing the Kernel::Type enumeration...
QStringList files(PvlGroup &grp)
This method retrieves the values of all of the "File" keywords in the given PvlGroup.
This is free and unencumbered software released into the public domain.
Parse and return pieces of a time string.
Kernel instrument(Pvl &lab)
This method finds the last Instrument kernel found that matches the (ik) criteria in the database and...
Container for cube-like labels.
Kernel targetPosition(Pvl &lab)
This method finds the highest version of all Target Position kernels (tspk) identified by the databas...
~KernelDb()
Destructs KernelDb object.
void loadSystemDb(const QString &mission, const Pvl &lab)
Loads the appropriate kernel database files with the defined BASE and MISSION info for each type of k...
Kernel dem(Pvl &lab)
This method finds the highest version of all Digital Terrain Models (DEMs) found that match the crite...
QList< FileName > m_kernelDbFiles
List of the kernel database file names that were read in when the loadSystemDb() method is called.
Pvl m_kernelData
Pvl containing the information in the kernel database(s) that is read in from the constructor and whe...
Contains multiple PvlContainers.
Kernel instrumentAddendum(Pvl &lab)
This method finds the highest version of all Instrument Addendum kernels (iak) identified by the data...
Kernel spacecraftClock(Pvl &lab)
This method finds the highest version of all Spacecraft Clock kernels (sclk) identified by the databa...
QList< FileName > kernelDbFiles()
Accessor method to retrieve the list of kernel database files that were read in when loadSystemDb() i...
QString m_filename
The name of the kernel database file.
void readKernelDbFiles()
This method is called by loadSystemDb() to read kernel database file list compiled by loadKernelDbFil...
static bool matches(const Pvl &lab, PvlGroup &kernelDbGrp, iTime timeToMatch, int cameraVersion)
This static method determines whether the given cube label matches the given criteria.
Kernel findLast(const QString &entry, Pvl &lab)
Finds the highest priority Kernel object for the given entry based on the allowed Kernel types.
QList< std::priority_queue< Kernel > > findAll(const QString &entry, Pvl &lab)
Finds all of the Kernel objects for the given entry value based on the allowed Kernel types.
This class stores Kernel information, including Type and kernel file names.
Kernel frame(Pvl &lab)
This method finds the highest version of all Frame kernels (fk) identified by the database and the al...
Kernel spacecraftPosition(Pvl &lab)
This method finds the highest version of all Spacecraft Position kernels (spk) identified by the data...
void loadKernelDbFiles(PvlGroup &dataDir, QString directory, const Pvl &lab)
This method is called by loadSystemDb() to create a list of all appropriate kernel database files to ...
QList< std::priority_queue< Kernel > > spacecraftPointing(Pvl &lab)
This method finds a list of the highest versions of all Spacecraft Pointing kernels (ck) identified b...
Kernel leapSecond(Pvl &lab)
This method finds the top priority of all Leap Second kernels (lsk) identified by the database and th...
This is free and unencumbered software released into the public domain.
unsigned int m_allowedKernelTypes
This integer value represents which Kernel::Types are allowed.