File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
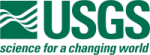 |
Isis 3 Programmer Reference
|
10 #include "tnt/tnt_array2d.h"
36 Matrix(
const int n,
const int m,
const double value = 0.0);
37 Matrix(TNT::Array2D<double> matrix);
43 return p_matrix.dim1();
45 inline int Columns() {
46 return p_matrix.dim2();
63 inline double *operator[](
int index) {
64 return p_matrix[index];
75 Matrix operator* (
double scalar) {
80 TNT::Array2D<double> p_matrix;
std::vector< double > Eigenvalues()
Compute the eigenvalues of the matrix.
Matrix Eigenvectors()
Compute the eigenvectors of the matrix and return them as columns of a matrix in ascending order.
Matrix Transpose()
Compute the transpose of the matrix.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
Matrix Add(Matrix &matrix)
Add the two matrices.
Matrix(const int n, const int m, const double value=0.0)
Constructs an n x m Matrix containing the specified default value.
Matrix Multiply(Matrix &matrix)
Multiply the two matrices.
Matrix Inverse()
Compute the inverse of the matrix.
double Trace()
Compute the determinant of the matrix.
double Determinant()
Compute the determinant of the matrix.
static Matrix Identity(const int n)
Create an n x n identity matrix.
Matrix Subtract(Matrix &matrix)
Subtract the input matrix from this matrix.
~Matrix()
Destroys the Matrix object.
Matrix MultiplyElementWise(Matrix &matrix)
Multiply the two matrices element-wise (ie compute C such that C[i][j] = A[i][j]*B[i][j])
This is free and unencumbered software released into the public domain.