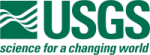 |
Isis 3 Programmer Reference
|
8 #include "NormModelFactory.h"
11 #include "IException.h"
40 QString algorithm =
"";
42 algorithm = QString(algo[
"NormName"]);
45 algorithm = QString(algo[
"Name"]);
48 QString msg =
"Keyword [Name] or keyword [NormName] must ";
49 msg +=
"exist in [Group = Algorithm]";
57 p->
read(
"NormModel.plugin");
60 p->
read(
"$ISISROOT/lib/NormModel.plugin");
67 return (*plugin)(pvl, pmodel);
96 QString algorithm =
"";
98 algorithm = QString(algo[
"NormName"]);
101 algorithm = QString(algo[
"Name"]);
104 IString msg =
"Keyword [Name] or keyword [NormName] must ";
105 msg +=
"exist in [Group = Algorithm]";
113 p->
read(
"NormModel.plugin");
116 p->
read(
"$ISISROOT/lib/NormModel.plugin");
125 return (*plugin)(pvl, pmodel, amodel);
File name manipulation and expansion.
bool fileExists() const
Returns true if the file exists; false otherwise.
Isotropic atmos scattering model.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
static NormModel * Create(Pvl &pvl, PhotoModel &pmodel)
Create a NormModel object using a PVL specification.
@ Traverse
Search child objects.
Contains multiple PvlContainers.
void read(const QString &file)
Loads PVL information from a stream.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
QFunctionPointer GetPlugin(const QString &group)
This method returns a void pointer to a C function (i.e., the plugin) It does this by looking in itse...
Adds specific functionality to C++ strings.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
Loads plugins from a shared library.