File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
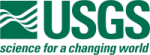 |
Isis 3 Programmer Reference
|
7 #include "ObservationNumberList.h"
10 #include "IException.h"
12 #include "SerialNumberList.h"
26 ObservationNumberList::ObservationNumberList(
const QString &listfile,
bool checkTarget) :
53 if (snlist->
size() == 0) {
54 QString msg =
"Serial number list is empty";
58 map<QString, int> observationMap;
64 for (
int isn = 0; isn < snlist->
size(); isn++) {
69 observationIndex = currentIndex++;
97 if (snlist->
size() == 0) {
98 QString msg =
"Cannot remove, serial number list is empty";
105 map<QString, int> observationMap;
107 int currentIndex = 0;
108 int observationIndex;
111 for (
int isn = 0; isn < this->
size(); isn++) {
120 observationIndex = currentIndex++;
155 QString observationNumber) {
158 nextset.serialNumberIndex = isn;
159 nextset.observationNumberIndex = observationIndex;
162 m_sets.push_back(nextset);
163 m_indexMap.insert(pair<int, int>(isn, observationIndex));
186 for (
unsigned index = 0; index <
m_pairs.size(); index++) {
228 QString msg =
"Requested filename [" +
FileName(filename).
expanded() +
"] ";
229 msg +=
"does not exist in the list";
233 return m_pairs[index].observationNumber;
247 if (index >= 0 && index < (
int)
m_pairs.size()) {
248 return m_pairs[index].observationNumber;
251 QString msg =
"Index [" +
toString(index) +
"] is invalid";
268 vector<QString> filenames;
269 for (
unsigned index = 0; index <
m_pairs.size(); index++) {
271 filenames.push_back(
m_pairs[index].filename);
274 if (filenames.size() > 0) {
278 QString msg =
"Requested observation number [" + on +
"] ";
279 msg +=
"does not exist in the list";
int observationSize() const
How many unique observations are in the list?
std::vector< QString > possibleFileNames(const QString &on)
Return possible filenames given an observation number.
QString observationNumber(int index)
Return a observation number given an index.
int size() const
How many serial number / filename combos are in the list.
int serialNumberIndex(const QString &sn)
Return a list index given a serial number.
File name manipulation and expansion.
An observation consiting of a serial number index to the ObservationNumberList, an observation number...
QString observationNumber(const QString &filename)
Return an observation number given a filename.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Serial Number list generator.
void remove(SerialNumberList *snlist)
Removes all of the listed serial numbers from the observation.
std::map< QString, int > m_fileMap
Maps filenames to their positions in the list.
int m_numberObservations
Count of observations in the observation number list.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
int observationNumberMapIndex(const int serialNumberIndex)
Return a observation index given a serial number index.
int fileNameIndex(const QString &filename)
Return a list index given a filename.
~ObservationNumberList()
Destructor.
void init(SerialNumberList *snlist)
Initiates the ObservationNumberList.
bool hasObservationNumber(const QString &on)
Determines whether or not the requested observation number exists in the list.
std::vector< Pair > m_pairs
List of serial number Pair entities.
std::multimap< int, int > m_indexMap
Maps serial number index to observation number index.
void add(int isn, const int observationIndex, QString observationNumber)
Adds a new serial number index / observation number index / observation number to the SerialNumberLis...
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
std::vector< ObservationSet > m_sets
List of observation sets.
bool hasSerialNumber(QString sn)
Determines whether or not the requested serial number exists in the list.
ObservationNumberList(const QString &list, bool checkTarget=true)
Creates an ObservationNumberList from a filename.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....