File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
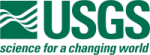 |
Isis 3 Programmer Reference
|
7 #include "SerialNumberList.h"
11 #include "IException.h"
14 #include "ObservationNumber.h"
17 #include "SerialNumber.h"
54 if (progress != NULL) {
55 progress->
SetText(
"Creating Isis serial numbers from list file.");
59 for (
int i = 0; i < flist.size(); i++) {
61 if (progress != NULL) {
67 QString msg =
"Can't open or invalid file list [" + listfile +
"].";
131 if (cubeObj.
hasGroup(
"Instrument")) {
132 targetGroup = cubeObj.
findGroup(
"Instrument");
134 else if (def2filename) {
137 targetGroup = cubeObj.
findGroup(
"Mapping");
140 QString msg =
"Unable to find Instrument or Mapping group in "
141 + filename +
" for comparing target.";
147 QString msg =
"Unable to find Instrument group in " + filename
148 +
" for comparing target.";
152 target = targetGroup[
"TargetName"][0];
153 target = target.toUpper();
158 QString msg =
"Target name of [" + target +
"] from file ["
159 + filename +
"] does not match [" +
m_target +
"].";
167 if (sn ==
"Unknown") {
168 QString msg =
"Invalid serial number [Unknown] from file ["
174 QString msg =
"Duplicate serial number [" + sn +
"] from files ["
181 nextpair.serialNumber = sn;
182 nextpair.observationNumber = on;
189 nextpair.spacecraftName = cubeObj.
findGroup(
"CsmInfo")[
"CSMPlatformID"][0];
190 nextpair.instrumentId = cubeObj.
findGroup(
"CsmInfo")[
"CSMInstrumentId"][0];
196 else if (cubeObj.
hasGroup(
"Instrument")) {
199 nextpair.spacecraftName = cubeObj.
findGroup(
"Instrument")[
"SpacecraftName"][0];
200 nextpair.instrumentId = cubeObj.
findGroup(
"Instrument")[
"InstrumentId"][0];
206 m_fileMap.insert(std::pair<QString, int>(nextpair.filename, (
int)(
m_pairs.size() - 1)));
210 "] can not be added to serial number list.";
261 if (cubeObj.
hasGroup(
"Instrument")) {
262 targetGroup = cubeObj.
findGroup(
"Instrument");
264 else if (cubeObj.
hasGroup(
"Mapping")) {
266 targetGroup = cubeObj.
findGroup(
"Mapping");
269 QString msg =
"Unable to find Instrument or Mapping group in "
270 + filename +
" for comparing target.";
274 target = targetGroup[
"TargetName"][0];
275 target = target.toUpper();
280 QString msg =
"Target name of [" + target +
"] from file ["
281 + filename +
"] does not match [" +
m_target +
"].";
288 QString msg =
"Invalid serial number [Unknown] from file ["
294 QString msg =
"Duplicate, serial number [" +
serialNumber +
"] from files ["
296 +
"] and [" +
fileName(index) +
"].";
305 if (!cubeObj.
hasGroup(
"Instrument")) {
306 QString msg =
"Unable to find Instrument group in " + filename
307 +
" needed for performing bundle adjustment.";
312 QString msg =
"Unable to find SpacecraftName or InstrumentId keywords in " + filename
313 +
" needed for performing bundle adjustment.";
321 QString msg =
"Unable to find CSMPlatformID or CSMInstrumentId keywords in " + filename
322 +
" needed for performing bundle adjustment.";
337 nextpair.spacecraftName = cubeObj.
findGroup(
"CsmInfo")[
"CSMPlatformID"][0];
338 nextpair.instrumentId = cubeObj.
findGroup(
"CsmInfo")[
"CSMInstrumentId"][0];
344 else if (cubeObj.
hasGroup(
"Instrument")) {
347 nextpair.spacecraftName = cubeObj.
findGroup(
"Instrument")[
"SpacecraftName"][0];
348 nextpair.instrumentId = cubeObj.
findGroup(
"Instrument")[
"InstrumentId"][0];
354 m_fileMap.insert(std::pair<QString, int>(nextpair.filename, (
int)(
m_pairs.size() - 1)));
357 QString msg =
"[SerialNumber, FileName] = [" +
serialNumber +
", "
359 +
"] can not be added to serial number list.";
402 return m_pairs[index].filename;
405 QString msg =
"Unable to get the FileName. The given serial number ["
406 + sn +
"] does not exist in the list.";
428 QString msg =
"Unable to get the SerialNumber. The given file name ["
433 return m_pairs[index].serialNumber;
447 if (index >= 0 && index < (
int)
m_pairs.size()) {
448 return m_pairs[index].serialNumber;
451 QString msg =
"Unable to get the SerialNumber. The given index ["
452 +
toString(index) +
"] is invalid.";
469 if (index >= 0 && index < (
int)
m_pairs.size()) {
470 return m_pairs[index].observationNumber;
473 QString msg =
"Unable to get the ObservationNumber. The given index ["
474 +
toString(index) +
"] is invalid.";
495 QString msg =
"Unable to get the SerialNumber index. The given serial number ["
496 + sn +
"] does not exist in the list.";
518 std::map<QString, int>::iterator pos;
520 QString msg =
"Unable to get the FileName index. The given file name ["
538 if (index >= 0 && index < (
int)
m_pairs.size()) {
539 return m_pairs[index].filename;
542 QString msg =
"Unable to get the FileName. The given index ["
543 +
toString(index) +
"] is invalid.";
560 if (index >= 0 && index < (
int)
m_pairs.size()) {
561 QString scid = (
m_pairs[index].spacecraftName +
"/" +
m_pairs[index].instrumentId).toUpper();
564 (void)scid.simplified();
565 return scid.replace(
" ",
"");
568 QString msg =
"Unable to get the Spacecraft InstrumentId. The given index ["
569 +
toString(index) +
"] is invalid.";
588 QString scid = (
m_pairs[index].spacecraftName +
"/" +
m_pairs[index].instrumentId).toUpper();
591 (void)scid.simplified();
592 return scid.replace(
" ",
"");
595 QString msg =
"Unable to get the Spacecraft InstrumentId. The given serial number ["
596 + sn +
"] does not exist in the list.";
614 std::vector<QString> numbers;
615 for (
unsigned index = 0; index <
m_pairs.size(); index++) {
620 if (numbers.size() > 0) {
624 QString msg =
"Unable to get the possible serial numbers. The given observation number ["
625 + on +
"] does not exist in the list.";
QString observationNumber(int index)
Return a observation number given an index.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
QString m_target
Target name that the files must have if m_checkTarget is true
Contains Pvl Groups and Pvl Objects.
void CheckStatus()
Checks and updates the status.
int size() const
How many serial number / filename combos are in the list.
int serialNumberIndex(const QString &sn)
Return a list index given a serial number.
File name manipulation and expansion.
void SetMaximumSteps(const int steps)
This sets the maximum number of steps in the process.
std::vector< QString > possibleSerialNumbers(const QString &on)
Return possible serial numbers given an observation number.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a SerialNumber from a PVL.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
QString serialNumber(const QString &filename)
Return a serial number given a filename.
void remove(const QString &sn)
Remove the specified serial number from the list.
std::map< QString, int > m_fileMap
Maps filenames to their positions in the list.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Contains multiple PvlContainers.
int fileNameIndex(const QString &filename)
Return a list index given a filename.
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
void add(const QString &filename, bool def2filename=false)
Adds a new filename / serial number pair to the SerialNumberList.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a ObservationNumber from a PVL.
Program progress reporter.
std::vector< Pair > m_pairs
List of serial number Pair entities.
std::map< QString, int > m_serialMap
Maps serial numbers to their positions in the list.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool m_checkTarget
Specifies whether or not to check to make sure the target names match between files added to the seri...
QString fileName(const QString &sn)
Return a filename given a serial number.
A serial number list entity that contains the filename serial number pair.
virtual ~SerialNumberList()
Destructor.
Internalizes a list of files.
bool hasSerialNumber(QString sn)
Determines whether or not the requested serial number exists in the list.
QString spacecraftInstrumentId(int index)
Return the spacecraftname/instrumentid at the given index.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
SerialNumberList(bool checkTarget=true)
Creates an empty SerialNumberList.