File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
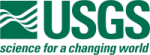 |
Isis 3 Programmer Reference
|
7 #include "ObservationNumber.h"
8 #include "IException.h"
12 #include "PvlToPvlTranslationManager.h"
42 if(!snTemp.isEmpty()) {
73 return Compose(p, def2filename);
85 static QString missionTransFile =
"$ISISROOT/appdata/translations/MissionName2DataDir.trn";
87 missionXlater.SetLabel(label);
88 QString mission = missionXlater.
Translate(
"MissionName");
91 static QString instTransFile =
"$ISISROOT/appdata/translations/Instruments.trn";
93 instrumentXlater.SetLabel(label);
94 QString instrument = instrumentXlater.
Translate(
"InstrumentName");
101 static std::map<QString, std::pair<PvlToPvlTranslationManager, PvlKeyword> > missionTranslators;
102 QString key = mission +
"_" + instrument;
103 std::map<QString, std::pair<PvlToPvlTranslationManager, PvlKeyword> >::iterator
104 translationIterator = missionTranslators.find(key);
106 if(translationIterator == missionTranslators.end()) {
108 FileName snFile((QString)
"$ISISROOT/appdata/translations/" + mission +
109 instrument +
"SerialNumber.trn");
114 if(translation.
hasKeyword(
"ObservationKeys")) {
115 observationKeys = translation[
"ObservationKeys"];
119 missionTranslators.insert(
120 std::pair<QString, std::pair<PvlToPvlTranslationManager, PvlKeyword> >
124 translationIterator = missionTranslators.find(key);
127 translationIterator->second.first.SetLabel(label);
128 translationIterator->second.first.Auto(outLabel);
132 if(!translationIterator->second.second.name().isEmpty()) {
133 snGroup += translationIterator->second.second;
151 std::vector<QString> sn;
152 for(
int i = 0; i < list.
size(); i++) {
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
A single keyword-value pair.
int size() const
How many serial number / filename combos are in the list.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
File name manipulation and expansion.
virtual QString Translate(QString translationGroupName, int findex=0)
Returns a translated value.
Container for cube-like labels.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Serial Number list generator.
QString serialNumber(const QString &filename)
Return a serial number given a filename.
virtual ~ObservationNumber()
Destroy a SerialNumber object.
Allows applications to translate simple text files.
std::vector< QString > PossibleSerial(const QString &on, SerialNumberList &list)
Creates a vector of plasible SerialNumbers from a string representing the ObservationNumber and a Ser...
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Contains multiple PvlContainers.
bool hasKeyword(const QString &kname, FindOptions opts) const
See if a keyword is in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within ...
QString fileName() const
Returns the filename used to initialise the Pvl object.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a ObservationNumber from a PVL.
IO Handler for Isis Cubes.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
static PvlGroup FindObservationTranslation(Pvl &label)
Get Groups by translating from correct Translation table.
int keywords() const
Returns the number of keywords contained in the PvlContainer.
static QString CreateSerialNumber(PvlGroup &snGroup, int key)
Create the SerialNumber string by concatenating the keywords in the label with '/' in between serialN...
ObservationNumber()
Create an empty SerialNumber object.
This is free and unencumbered software released into the public domain.