File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
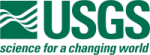 |
Isis 3 Programmer Reference
|
7 #include "Orthographic.h"
14 #include "Constants.h"
15 #include "IException.h"
16 #include "TProjection.h"
19 #include "PvlKeyword.h"
39 Orthographic::Orthographic(
Pvl &label,
bool allowDefaults) :
49 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterLongitude"))) {
58 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterLatitude"))) {
72 QString msg =
"The center longitude cannot exceed [-360, 360]. "
100 double sinphi, cosphi, coslon;
127 QString msg =
"The longitude range cannot exceed 360 degrees.";
149 QString message =
"Invalid label group [Mapping]";
167 if (!Projection::operator==(proj))
return false;
181 return "Orthographic";
224 double lonRadians = lon *
PI / 180.0;
229 double latRadians = lat;
231 latRadians *=
PI / 180.0;
235 double sinphi = sin(latRadians);
236 double cosphi = cos(latRadians);
237 double coslon = cos(deltaLon);
241 if ((g <= 0.0) && (fabs(g) > 1.0e-10)) {
273 double rho, con, z, sinz, cosz;
274 const double epsilon = 1.0e-10;
290 if (fabs(rho) <= epsilon) {
295 if (con > 1.0) con = 1.0;
296 if (con < -1.0) con = -1.0;
301 if (con > 1.0) con = 1.0;
302 if (con < -1.0) con = -1.0;
305 if (fabs(con) <= epsilon) {
315 if ((fabs(con) >= epsilon) || (fabs(
GetX()) >= epsilon)) {
374 double &minY,
double &maxY) {
381 bool correctedMinLon =
false;
383 if (adjustedMinLon >= adjustedMaxLon) {
384 adjustedMinLon -= 360;
385 correctedMinLon =
true;
439 for (
double angle = 0.0; angle <= 360.0; angle += 0.01) {
450 adjustedLon <= adjustedMaxLon &&
452 adjustedLon >= adjustedMinLon) {
530 bool allowDefaults) {
double m_maximumLatitude
Contains the maximum latitude for the entire ground range.
bool XYRange(double &minX, double &maxX, double &minY, double &maxY)
This method is used to determine the x/y range which completely covers the area of interest specified...
const double HALFPI
The mathematical constant PI/2.
double m_longitude
This contains the currently set longitude value.
int m_longitudeDomain
This integer is either 180 or 360 and is read from the labels.
QString Version() const
Returns the version of the map projection.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
@ Io
A type of error that occurred when performing an actual I/O operation.
double m_minimumLongitude
Contains the minimum longitude for the entire ground range.
double m_latitude
This contains the currently set latitude value.
const double PI
The mathematical constant PI.
A single keyword-value pair.
PvlGroup Mapping()
This function returns the keywords that this projection uses.
LongitudeDirection m_longitudeDirection
An enumerated type indicating the LongitudeDirection read from the labels.
double m_minimumLatitude
Contains the minimum latitude for the entire ground range.
@ PositiveWest
Longitude values increase in the westerly direction.
virtual double MinimumLongitude() const
This returns the minimum longitude of the area of interest.
double m_minimumY
See minimumX description.
double GetX() const
Calculates the unrotated form of current x value.
QString Name() const
Returns the name of the map projection, "Orthographic".
Orthographic Map Projection.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void SetXY(double x, double y)
This protected method is a helper for derived classes.
double ToPlanetocentric(const double lat) const
This method converts a planetographic latitude to a planetocentric latitude.
void XYRangeCheck(const double latitude, const double longitude)
This convience function is established to assist in the development of the XYRange virtual method.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
PvlGroup m_mappingGrp
Mapping group that created this projection.
double GetY() const
Calculates the unrotated form of the current y value.
bool SetCoordinate(const double x, const double y)
This method is used to set the projection x/y.
@ Traverse
Search child objects.
bool SetGround(const double lat, const double lon)
This method is used to set the latitude/longitude (assumed to be of the correct LatitudeType,...
double m_minimumX
The data elements m_minimumX, m_minimumY, m_maximumX, and m_maximumY are convience data elements when...
Contains multiple PvlContainers.
bool m_good
Indicates if the contents of m_x, m_y, m_latitude, and m_longitude are valid.
Base class for Map TProjections.
static double To180Domain(const double lon)
This method converts a longitude into the -180 to 180 domain.
~Orthographic()
Destroys the Orthographic object.
virtual double MaximumLongitude() const
This returns the maximum longitude of the area of interest.
PvlGroup MappingLongitudes()
This function returns the longitude keywords that this projection uses.
virtual PvlGroup MappingLongitudes()
This function returns the longitude keywords that this projection uses.
double m_sinph0
Sine of the center latitude.
void SetComputedXY(double x, double y)
This protected method is a helper for derived classes.
bool operator==(const Projection &proj)
Compares two Projection objects to see if they are equal.
Namespace for the standard library.
double m_maximumLongitude
Contains the maximum longitude for the entire ground range.
virtual PvlGroup MappingLatitudes()
This function returns the latitude keywords that this projection uses.
double ToPlanetographic(const double lat) const
This method converts a planetocentric latitude to a planetographic latitude.
const double E
Sets some basic constants for use in ISIS programming.
PvlGroup MappingLatitudes()
This function returns the latitude keywords that this projection uses.
virtual PvlGroup Mapping()
This function returns the keywords that this projection uses.
double m_equatorialRadius
Polar radius of the target.
static double To360Domain(const double lon)
This method converts a longitude into the 0 to 360 domain.
bool IsPlanetocentric() const
This indicates if the latitude type is planetocentric (as opposed to planetographic).
double m_cosph0
Cosine of the center latitude.
double m_maximumY
See minimumX description.
Base class for Map Projections.
double m_maximumX
See minimumX description.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
double TrueScaleLatitude() const
Returns the center latitude, in degrees.
double m_centerLongitude
The center longitude for the map projection.
double m_centerLatitude
The center latitude for the map projection.