File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
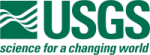 |
Isis 3 Programmer Reference
|
7 #include "PointPerspective.h"
13 #include "Constants.h"
14 #include "IException.h"
17 #include "PvlKeyword.h"
18 #include "TProjection.h"
39 PointPerspective::PointPerspective(
Pvl &label,
bool allowDefaults) :
47 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterLongitude"))) {
54 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterLatitude"))) {
83 QString message =
"Invalid label group [Mapping]";
101 if (!Projection::operator==(proj))
return false;
107 (point->
m_distance != this->m_distance))
return false;
117 return "PointPerspective";
164 double lonRadians = lon *
PI / 180.0;
169 double latRadians = lat;
171 latRadians *=
PI / 180.0;
175 double sinphi = sin(latRadians);
176 double cosphi = cos(latRadians);
177 double coslon = cos(deltaLon);
181 if (g < (1.0 /
m_P)) {
186 double ksp = (
m_P - 1.0) / (
m_P - g);
192 if (sqrt(x*x+y*y)> projectionRadius) {
224 double rho, rp, con, com, z, sinz, cosz;
225 const double epsilon = 1.0e-10;
232 if (rp > (sqrt(con / com))) {
239 if (fabs(rho) <= epsilon) {
247 sinz = (
m_P - sqrt(1.0 - rp * rp * com / con)) / (con / rp + rp / con);
253 if (con > 1.0) con = 1.0;
254 if (con < -1.0) con = -1.0;
258 if (fabs(con) <= epsilon) {
268 if ((fabs(con) >= epsilon) || (fabs(
GetX()) >= epsilon)) {
329 minX =
XCoord() - projectionRadius;
330 maxX =
XCoord() + projectionRadius;
331 minY =
YCoord() - projectionRadius;
332 maxY =
YCoord() + projectionRadius;
391 bool allowDefaults) {
PvlGroup MappingLatitudes()
This function returns the latitude keywords that this projection uses.
double m_maximumLatitude
Contains the maximum latitude for the entire ground range.
const double HALFPI
The mathematical constant PI/2.
QString Name() const
Returns the name of the map projection, "PointPerspective".
double m_longitude
This contains the currently set longitude value.
int m_longitudeDomain
This integer is either 180 or 360 and is read from the labels.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
@ Io
A type of error that occurred when performing an actual I/O operation.
double m_minimumLongitude
Contains the minimum longitude for the entire ground range.
PointPerspective Map Projection.
double m_latitude
This contains the currently set latitude value.
const double PI
The mathematical constant PI.
A single keyword-value pair.
LongitudeDirection m_longitudeDirection
An enumerated type indicating the LongitudeDirection read from the labels.
double m_minimumLatitude
Contains the minimum latitude for the entire ground range.
@ PositiveWest
Longitude values increase in the westerly direction.
PvlGroup MappingLongitudes()
This function returns the longitude keywords that this projection uses.
double m_P
Perspective Point.
~PointPerspective()
Destroys the PointPerspective object.
double m_centerLongitude
The center longitude for the map projection.
double GetX() const
Calculates the unrotated form of current x value.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void SetXY(double x, double y)
This protected method is a helper for derived classes.
double ToPlanetocentric(const double lat) const
This method converts a planetographic latitude to a planetocentric latitude.
double m_centerLatitude
The center latitude for the map projection.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
PvlGroup Mapping()
This function returns the keywords that this projection uses.
PvlGroup m_mappingGrp
Mapping group that created this projection.
double GetY() const
Calculates the unrotated form of the current y value.
bool SetGround(const double lat, const double lon)
This method is used to set the latitude/longitude (assumed to be of the correct LatitudeType,...
@ Traverse
Search child objects.
Contains multiple PvlContainers.
bool operator==(const Projection &proj)
Compares two Projection objects to see if they are equal.
bool m_good
Indicates if the contents of m_x, m_y, m_latitude, and m_longitude are valid.
Base class for Map TProjections.
double m_distance
Distance fromp perspective point to planet center.
double m_cosph0
Cosine of the center latitude.
static double To180Domain(const double lon)
This method converts a longitude into the -180 to 180 domain.
double TrueScaleLatitude() const
Returns the latitude of true scale, in degrees.
virtual PvlGroup MappingLongitudes()
This function returns the longitude keywords that this projection uses.
void SetComputedXY(double x, double y)
This protected method is a helper for derived classes.
Namespace for the standard library.
QString Version() const
Returns the version of the map projection.
double m_maximumLongitude
Contains the maximum longitude for the entire ground range.
virtual PvlGroup MappingLatitudes()
This function returns the latitude keywords that this projection uses.
double ToPlanetographic(const double lat) const
This method converts a planetocentric latitude to a planetographic latitude.
virtual PvlGroup Mapping()
This function returns the keywords that this projection uses.
double m_equatorialRadius
Polar radius of the target.
static double To360Domain(const double lon)
This method converts a longitude into the 0 to 360 domain.
bool SetCoordinate(const double x, const double y)
This method is used to set the projection x/y.
bool XYRange(double &minX, double &maxX, double &minY, double &maxY)
This method is used to determine the x/y range which completely covers the area of interest specified...
bool IsPlanetocentric() const
This indicates if the latitude type is planetocentric (as opposed to planetographic).
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
Base class for Map Projections.
double m_sinph0
Sine of the center latitude.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
This is free and unencumbered software released into the public domain.