File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
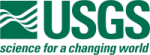 |
Isis 3 Programmer Reference
|
1 #ifndef ProcessImportPds_h
2 #define ProcessImportPds_h
9 #include "ProcessImport.h"
18 class PvlToPvlTranslationManager;
225 CombinedSpectrum = 128,
226 All =
Image | Qube | SpectralQube | L0 | Rdn | Loc | Obs | CombinedSpectrum
232 void SetPdsFile(
const QString &pdsLabelFile,
const QString &pdsDataFile,
233 Pvl &pdsLabel, PdsFileType allowedTypes = All);
234 void SetPdsFile(
const Pvl &pdsLabelPvl,
const QString &pdsDataFile,
235 PdsFileType allowedTypes = All);
236 void ProcessLabel(
const QString &pdsDataFile, PdsFileType allowedTypes);
270 const bool & calcOffsetOnly);
281 double &xmult,
double &ymult);
311 QString p_targetName;
336 double p_sampleProjectionOffset;
337 double p_lineProjectionOffset;
double p_maximumLatitude
Maximum latitude found in the PDS projection labels.
PvlGroup GetProjectionOffsetGroup()
Return the projection offsets.
void Finalize()
End the processing sequence and cleans up by closing cubes, freeing memory, etc.
void ProcessSpecialPixels(PvlToPvlTranslationManager &pdsXlater, const bool &isQube)
Handles all special pixel setting, ultimately, calls SetSpecialValues.
void StartProcess()
This method will write the cube and table data to the output cube.
Pvl p_pdsLabel
Internalized PDS label.
bool p_projectionOffsetChange
Whether the projection offsets were updated upon loading.
void TranslateIsis2Labels(Pvl &lab)
Translate as many of the ISIS2 labels as possible.
int p_longitudeDomain
Longitude domain found in the PDS projection labels.
void TranslatePdsLabels(Pvl &lab)
Translate as many of the PDS labels as possible.
Container for cube-like labels.
void ProcessPdsQubeLabel(const QString &pdsDataFile, const QString &transFile)
Process the PDS label of type QUBE or SPECTRALQUBE.
void TranslatePdsArchive(Pvl &lab)
Fill as many of the Isis BandBin labels as possible.
void TranslatePdsProjection(Pvl &lab)
Fills the passed in label with the projection information from the PDS label file.
double p_pixelResolution
Pixel resolution found in the PDS projection labels.
double p_minimumLatitude
Minimum latitude found in the PDS projection labels.
Buffer for reading and writing cube data.
void TranslateIsis2BandBin(Pvl &lab)
Fill as many of the Isis BandBin labels as possible.
Allows applications to translate simple text files.
double p_rotation
The rotation found in the PDS labels.
Contains multiple PvlContainers.
void TranslatePdsBandBin(Pvl &lab)
Fill as many of the Isis BandBin labels as possible.
double p_maximumLongitude
Maximum longitude found in the PDS projection labels.
void ProcessPixelBitandType(PvlToPvlTranslationManager &pdsXlater)
Handles PixelType and BitsPerPixel Calls SetPixelType with the correct values.
void ProcessPdsImageLabel(const QString &pdsDataFile)
Process the PDS label of type IMAGE.
void ProcessDataFilePointer(PvlToPvlTranslationManager &pdsXlater, const bool &calcOffsetOnly)
Handles the DataFilePointer keyword, aka ^QUBE or ^IMAGE.
double p_minimumLongitude
Minimum longitude found in the PDS projection labels.
Class for storing Table blobs information.
bool IsIsis2()
Return true if ISIS2 cube, else return false.
QString p_labelFile
The filename where the PDS label came from.
void OmitOriginalLabel()
Prevents the Original Label blob from being written out to the end of the cube.
ProcessImportPds()
Constructor.
This represents a cube in a project-based GUI interface.
std::vector< Table > p_tables
Vector of Isis Table objects that were imported from PDS and need to be added to the imported cube fi...
double p_polarRadius
The polar radius found in the PDS projection labels.
Table & ImportTable(QString pdsTableName)
This method will import the PDS table with the given name into an Isis Table object.
virtual void StartProcess()
Process the input file and write it to the output.
void ExtractPdsProjection(PvlToPvlTranslationManager &pdsXlater)
Extract all possible PDS projection parameters from the PDS label.
Convert PDS archive files to Isis format.
void ProcessPdsM3Label(const QString &pdsDataFile, PdsFileType fileType)
Process Chandrayaan M3 PDS label.
QString p_latitudeType
The latitude type found in the PDS projection labels.
void ProcessPdsCombinedSpectrumLabel(const QString &pdsDataFile)
Process the PDS label of type CombinedSpectrum.
void GetProjectionOffsetMults(double &xoff, double &yoff, double &xmult, double &ymult)
Read mults and offsets from a def file in order to calculate the upper left x/y.
double p_equatorialRadius
Equatorial radius found in the PDS projection labels.
QString p_transDir
Base data directory.
EncodingType p_encodingType
The encoding type of the image data.
QString p_jp2File
The name of the file containing the encoded JP2 data.
bool GetProjectionOffsetChange()
Return whether the projection offsets have changed.
bool p_keepOriginalLabel
determines whether or not to keep the OriginalLabel blob.
double p_scaleFactor
The scale factor found in the PDS projection labels.
QString p_projection
The name of the projection found in the PDS projection labels.
void SetPdsFile(const QString &pdsLabelFile, const QString &pdsDataFile, Pvl &pdsLabel, PdsFileType allowedTypes=All)
Set the input label file, data file and initialize a Pvl with the PDS labels.
void TranslateIsis2Instrument(Pvl &lab)
Fill as many of the Isis instrument labels as possible.
This is free and unencumbered software released into the public domain.
QString p_longitudeDirection
Longitude direction found in the PDS projection labels.
void IdentifySource(Pvl &lab)
Identify the source of this file PDS or ISIS2.
void ProcessLabel(const QString &pdsDataFile, PdsFileType allowedTypes)
Load the PDS labels after determining what type of data file was provided.
PvlGroup p_projectionOffsetGroup
Log information for projection offsets.