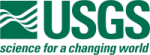 |
Isis 3 Programmer Reference
|
7 #include "ProcessImport.h"
14 #include "Application.h"
15 #include "BoxcarCachingAlgorithm.h"
18 #include "CubeAttribute.h"
19 #include "IException.h"
21 #include "JP2Decoder.h"
22 #include "LineManager.h"
23 #include "PixelType.h"
26 #include "PvlTokenizer.h"
27 #include "SpecialPixel.h"
28 #include "UserInterface.h"
30 #define EXPONENT_MASK ((char) 0x7F)
70 p_vax_convert =
false;
93 for(
unsigned int j = 0; j <
p_dataPre.size(); j++) {
95 for(
unsigned int i = 0; i < temp.size(); i++)
delete [] temp[i];
98 for(
unsigned int j = 0; j <
p_dataPost.size(); j++) {
100 for(
unsigned int i = 0; i < temp.size(); i++)
delete [] temp[i];
120 unsigned int VAX_NULL = 0xFFFFFFFF;
121 unsigned int VAX_MIN = 0xFFEFFFFF;
122 unsigned int VAX_LRS = 0xFFFEFFFF;
123 unsigned int VAX_LIS = 0xFFFDFFFF;
124 unsigned int VAX_HIS = 0xFFFCFFFF;
125 unsigned int VAX_HRS = 0xFFFBFFFF;
129 memcpy(&x, vax,
sizeof(
unsigned int));
133 n = memcmp(&VAX_NULL, &x,
sizeof(
unsigned int));
134 if (n == 0)
return true;
137 n = memcmp(&VAX_LRS, &x,
sizeof(
unsigned int));
138 if (n == 0)
return true;
141 n = memcmp(&VAX_LIS, &x,
sizeof(
unsigned int));
142 if (n == 0)
return true;
145 n = memcmp(&VAX_HIS, &x,
sizeof(
unsigned int));
146 if (n == 0)
return true;
149 n = memcmp(&VAX_HRS, &x,
sizeof(
unsigned int));
150 if (n == 0)
return true;
153 n = memcmp(&VAX_MIN, &x,
sizeof(
unsigned int));
154 if (n == 0)
return true;
175 bool swap_bytes =
false;
176 bool swap_words =
true;
184 unsigned int *oli, *ili;
190 out_order = Isis::Lsb;
194 out_order = Isis::Msb;
197 if (in_order != out_order) {
201 oli = (
unsigned int * ) ibuf;
202 ili = (
unsigned int * ) ibuf;
209 return Isis::LOW_INSTR_SAT8;
213 return Isis::LOW_REPR_SAT8;
217 return Isis::HIGH_INSTR_SAT8;
221 return Isis::HIGH_REPR_SAT8;
225 return Isis::VALID_MIN8;
230 *oli = (*ili <<16) | (*ili >> 16);
233 osi = (
unsigned short* ) oli;
238 osi[0] = (osi[0] >> 8 ) | (osi[0] << 8);
239 osi[1] = (osi[1] >> 8 ) | (osi[1] << 8);
243 oci = (
char *) &osi[exp_word];
245 if ( (oci[exp_byte] & EXPONENT_MASK) != exp_mask) {
246 oci[exp_byte] += exp_adjust;
249 result = *(
float *)oli;
250 dresult =
static_cast<double>(result);
266 if ((type == Isis::Double) || (type == Isis::Real) || (type == Isis::SignedWord) ||
267 (type == Isis::UnsignedWord) || (type == Isis::UnsignedByte) ||
268 (type == Isis::SignedInteger) || type==Isis::UnsignedInteger) {
272 QString msg =
"Unsupported pixel type [" +
280 if ((type == Isis::Double) || (type == Isis::Real) || (type == Isis::SignedWord) ||
281 (type == Isis::UnsignedWord) || (type == Isis::UnsignedByte)) {
285 QString msg =
"Unsupported pixel type [" +
305 if (ns > 0 && nl > 0 && nb > 0) {
311 QString msg =
"Illegal dimension [" +
toString(ns) +
", " +
354 QString msg =
"Illegal file header size [" +
toString(bytes) +
"]";
384 QString msg =
"Illegal file trailer size [" +
toString(bytes) +
"]";
413 QString msg =
"Illegal data header size [" +
toString(bytes) +
"]";
419 void ProcessImport::SetSuffixOffset(
int samples,
int lines,
int coreBands,
int itemBytes) {
449 QString msg =
"Illegal data trailer size [" +
toString(bytes) +
"]";
479 QString msg =
"Illegal data prefix size [" +
toString(bytes) +
"]";
509 QString msg =
"Illegal data suffix size [" +
toString(bytes) +
"]";
534 QString msg =
"File header bytes equals 0. There is nothing to save. "
535 "Use SetFileHeaderBytes() first.";
561 QString msg =
"File trailer bytes equals 0. There is nothing to save. "
562 "Use SetFileTrailerBytes() first.";
590 QString msg =
"Data header bytes equals 0. There is nothing to save. "
591 "Use SetDataHeaderBytes() first.";
619 QString msg =
"Data trailer bytes equals 0. There is nothing to save. "
620 "Use SetDataTrailerBytes() first.";
647 QString msg =
"Data prefix bytes equals 0. There is nothing to save. "
648 "Use SetDataPrefixBytes() first.";
676 QString msg =
"Data suffix bytes equals 0. There is nothing to save. "
677 "Use SetDataSuffixBytes() first.";
757 QString msg =
"File header was not saved. Use SaveFileHeader().";
783 QString msg =
"File trailer was not saved. Use SaveFileTrailer()";
811 QString msg =
"Data header was not saved. Use SaveDataHeader()";
839 QString msg =
"Data trailer was not saved. Use SaveDataTrailer()";
867 QString msg =
"Data prefix was not saved. Use SaveDataPrefix()";
895 QString msg =
"Data suffix was not saved. Use SaveDataSuffix()";
920 p_vax_convert = vax_convert;
994 const double lis,
const double hrs,
1100 if (pixelMin == DBL_MAX || pixelMax == -DBL_MAX)
return;
1105 (pixelMin < p_null_min && pixelMax >
p_null_max))) {
1107 QString msg =
"The " + pixelName +
" range [" +
toString(pixelMin) +
1108 "," +
toString(pixelMax) +
"] overlaps the NULL range [" +
1116 (pixelMin < p_lrs_min && pixelMax >
p_lrs_max))) {
1117 QString msg =
"The " + pixelName +
" range [" +
toString(pixelMin) +
1118 "," +
toString(pixelMax) +
"] overlaps the LRS range [" +
1126 (pixelMin < p_lis_min && pixelMax >
p_lis_max))) {
1127 QString msg =
"The " + pixelName +
" range [" +
toString(pixelMin) +
1128 "," +
toString(pixelMax) +
"] overlaps the LIS range [" +
1136 (pixelMin < p_hrs_min && pixelMax >
p_hrs_max))) {
1137 QString msg =
"The " + pixelName +
" range [" +
toString(pixelMin) +
1138 "," +
toString(pixelMax) +
"] overlaps the HRS range [" +
1146 (pixelMin < p_his_min && pixelMax >
p_his_max))) {
1147 QString msg =
"The " + pixelName +
" range [" +
toString(pixelMin) +
1148 "," +
toString(pixelMax) +
"] overlaps the HIS range [" +
1168 if (pixel <= p_null_max && pixel >=
p_null_min) {
1171 else if (pixel <= p_hrs_max && pixel >=
p_hrs_min) {
1172 return Isis::HIGH_REPR_SAT8;
1174 else if (pixel <= p_lrs_max && pixel >=
p_lrs_min) {
1175 return Isis::LOW_REPR_SAT8;
1177 else if (pixel <= p_his_max && pixel >=
p_his_min) {
1178 return Isis::HIGH_INSTR_SAT8;
1180 else if (pixel <= p_lis_max && pixel >=
p_lis_min) {
1181 return Isis::LOW_INSTR_SAT8;
1204 min = Isis::VALID_MIN4;
1205 max = Isis::VALID_MAX4;
1208 min = Isis::IVALID_MIN4;
1209 max = Isis::IVALID_MAX4;
1213 min = Isis::VALID_MINUI4;
1214 max = Isis::VALID_MAXUI4;
1229 QString msg =
"Unsupported pixel type [" +
1317 +
"] is not in a supported organization.";
1346 QString msg =
"File [" +
p_inFile +
"] is not in a supported organization.";
1366 readBytes = readBytes *
p_ns;
1367 char *in =
new char [readBytes];
1371 tok = tok.toUpper();
1377 QString inFileName(inFile.
expanded());
1378 fin.open(inFileName.toLatin1().data(), ios::in | ios::binary);
1379 if (!fin.is_open()) {
1380 QString msg =
"Cannot open input file [" +
p_inFile +
"]";
1385 streampos pos = fin.tellg();
1397 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1398 toString((
int)pos) +
"]. Byte count [" +
1406 if (funct != NULL) {
1418 for(
int band = 0; band <
p_nb; band++) {
1442 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1443 toString((
int)pos) +
"]. Byte count [" +
1449 vector<char *> tempPre, tempPost;
1452 for(
int line = 0; line <
p_nl; line++) {
1466 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1467 toString((
int)pos) +
"]. Byte count [" +
1474 fin.read(in, readBytes);
1476 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1477 toString((
int)pos) +
"]. Byte count [" +
1484 for(
int samp = 0; samp <
p_ns; samp++) {
1486 case Isis::UnsignedByte:
1487 (*out)[samp] = (double)((
unsigned char *)in)[samp];
1489 case Isis::UnsignedWord:
1493 case Isis::SignedWord:
1494 (*out)[samp] = (double)swapper.
ShortInt((
short int *)in+samp);
1496 case Isis::SignedInteger:
1497 (*out)[samp] = (double)swapper.
Int((
int *)in+samp);
1500 case Isis::UnsignedInteger:
1501 (*out)[samp] = (double)swapper.
Uint32_t((
unsigned int *)in+samp);
1508 (*out)[samp] = (double)swapper.
Float((
float *)in+samp);
1512 (*out)[samp] = (double)swapper.
Double((
double *)in+samp);
1521 (*out)[samp] = mult * ((*out)[samp]) + base;
1525 if (funct == NULL) {
1551 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1552 toString((
int)pos) +
"]. Byte count [" +
1582 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1583 toString((
int)pos) +
"]. Byte count [" +
1593 fin.seekg(0, ios_base::end);
1594 streampos e = fin.tellg();
1602 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1603 toString((
int)pos) +
"]. Byte count [" +
1630 readBytes = readBytes *
p_ns;
1631 char *in =
new char [readBytes];
1635 tok = tok.toUpper();
1641 QString inFileName(inFile.
expanded());
1642 fin.open(inFileName.toLatin1().data(), ios::in | ios::binary);
1643 if (!fin.is_open()) {
1644 QString msg =
"Cannot open input file [" +
p_inFile +
"]";
1649 streampos pos = fin.tellg();
1662 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1663 toString((
int)pos) +
"]. Byte count [" +
1671 if (funct != NULL) {
1685 for(
int line = 0; line <
p_nl; line++) {
1687 for(
int band = 0; band <
p_nb; band++) {
1701 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1702 toString((
int)pos) +
"]. Byte count [" +
1708 vector<char *> tempPre, tempPost;
1722 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1723 toString((
int)pos) +
"]. Byte count [" +
1731 fin.read(in, readBytes);
1733 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1734 toString((
int)pos) +
"]. Byte count [" +
1741 for(
int samp = 0; samp <
p_ns; samp++) {
1743 case Isis::UnsignedByte:
1744 (*out)[samp] = (double)((
unsigned char *)in)[samp];
1746 case Isis::UnsignedWord:
1750 case Isis::SignedWord:
1751 (*out)[samp] = (double)swapper.
ShortInt((
short int *)in+samp);
1753 case Isis::SignedInteger:
1754 (*out)[samp] = (double)swapper.
Int((
int *)in+samp);
1756 case Isis::UnsignedInteger:
1757 (*out)[samp] = (double)swapper.
Uint32_t((
unsigned int *)in+samp);
1764 (*out)[samp] = (double)swapper.
Float((
float *)in+samp);
1768 (*out)[samp] = (double)swapper.
Double((
double *)in+samp);
1778 (*out)[samp] = mult * ((*out)[samp]) + base;
1782 if (funct == NULL) {
1805 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1806 toString((
int)pos) +
"]. Byte count [" +
1825 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1826 toString((
int)pos) +
"]. Byte count [" +
1838 fin.seekg(0, ios_base::end);
1839 streampos e = fin.tellg();
1847 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1848 toString((
int)pos) +
"]. Byte count [" +
1875 tok = tok.toUpper();
1881 QString inFileName(inFile.
expanded());
1882 fin.open(inFileName.toLatin1().data(), ios::in | ios::binary);
1883 if (!fin.is_open()) {
1884 QString msg =
"Cannot open input file [" +
p_inFile +
"]";
1889 streampos pos = fin.tellg();
1901 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1902 toString((
int)pos) +
"]. Byte count [" +
1912 if (funct != NULL) {
1925 int readBytes =
p_ns * sampleBytes;
1926 char *in =
new char [readBytes];
1929 for(
int line = 0; line <
p_nl; line++) {
1932 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1933 toString((
int)pos) +
"]. Byte count [" +
1939 vector<char *> tempPre, tempPost;
1943 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1944 toString((
int)pos) +
"]. Byte count [" +
1951 fin.read(in, readBytes);
1953 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
1954 toString((
int)pos) +
"]. Byte count [" +
1960 for(
int band = 0; band <
p_nb; band++) {
1976 for(
int samp = 0; samp <
p_ns; samp++) {
1979 case Isis::UnsignedByte:
1980 (*out)[osamp] = (double)((
unsigned char *)in)[bufferIndex];
1982 case Isis::UnsignedWord:
1986 case Isis::SignedWord:
1987 (*out)[osamp] = (double)swapper.
ShortInt(&in[bufferIndex]);
1989 case Isis::SignedInteger:
1990 (*out)[samp] = (double)swapper.
Int(&in[bufferIndex]);
1992 case Isis::UnsignedInteger:
1993 (*out)[samp] = (double)swapper.
Uint32_t(&in[bufferIndex]);
2000 (*out)[osamp] = (double)swapper.
Float(&in[bufferIndex]);
2004 (*out)[osamp] = (double)swapper.
Double(&in[bufferIndex]);
2011 (*out)[osamp] =
TestPixel((*out)[osamp]);
2014 (*out)[osamp] = mult * ((*out)[osamp]) + base;
2019 if (funct == NULL) {
2032 for(
int samp = 0; samp <
p_ns; samp++) {
2035 tempPre.push_back(samplePrefix);
2041 for(
int samp = 0; samp <
p_ns; samp++) {
2045 tempPost.push_back(sampleSuffix);
2053 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
2054 toString((
int)pos) +
"]. Byte count [" +
2071 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
2072 toString((
int)pos) +
"]. Byte count [" +
2084 fin.seekg(0, ios_base::end);
2085 streampos e = fin.tellg();
2093 QString msg =
"Cannot read file [" +
p_inFile +
"]. Position [" +
2094 toString((
int)pos) +
"]. Byte count [" +
2133 p_ns = JP2_decoder->GetSampleDimension();
2134 p_nl = JP2_decoder->GetLineDimension();
2135 p_nb = JP2_decoder->GetBandDimension();
2141 int endsamp = startsamp +
p_ns;
2142 int readBytes = sizeofpixel *
p_ns *
p_nb
2144 char **in =
new char* [
p_nb];
2145 for(
int i = 0; i <
p_nb; i++) {
2146 in[i] =
new char [readBytes];
2152 if (funct != NULL) {
2164 for(
int line = 0; line <
p_nl; line++) {
2166 JP2_decoder->
Read((
unsigned char **)in);
2169 JP2_decoder->
Read((
short int **)in);
2172 for(
int band = 0; band <
p_nb; band++) {
2185 vector<char *> tempPre, tempPost;
2196 for(
int samp = startsamp; samp < endsamp; samp++) {
2198 case Isis::UnsignedByte:
2199 (*out)[samp] = (double)((
unsigned char *)in[band])[samp];
2201 case Isis::UnsignedWord:
2202 (*out)[samp] = (double)((
unsigned short int *)in[band])[samp];
2204 case Isis::SignedWord:
2205 (*out)[samp] = (double)((
short int *)in[band])[samp];
2215 (*out)[samp] = mult * ((*out)[samp]) + base;
2219 if (funct == NULL) {
2267 void ProcessImport::AddLabel(
Isis::Pvl &label) {
2272 output->Find(
"IsisCube");
2273 output->Insert(label);
2282 void ProcessImport::AddImportLabel(
Isis::Pvl &importLab) {
2287 output->Find(
"IsisCube");
2289 output->Insert(importLab);
2305 QString msg =
"File [" + file +
"] does not exist";
2321 QString msg =
"No input file has been set";
float Float(void *buf)
Swaps a floating point value.
unsigned short int UnsignedShortInt(void *buf)
Swaps an unsigned short integer value.
std::vector< char * > DataHeader()
This method returns a pointer to the data header.
int SizeOf(Isis::PixelType pixelType)
Returns the number of bytes of the specified PixelType.
std::vector< double > p_mult
An array containing the core multiplier for each band.
Isis::CubeAttributeOutput & GetOutputAttribute(const QString ¶mName)
Gets the attributes for an output cube.
void SetDataPrefixBytes(const int bytes)
This method sets the number of bytes at the beginning of each data record of a file.
void SetLIS(const double lis_min, const double lis_max)
Sets the range that will be considered Isis::Null.
void SetDataHeaderBytes(const int bytes)
This method sets the number of bytes in the header of each datablock of a file.
bool IsVAXSpecial(unsigned int *vax, VAXSpecialPixel pix)
Determines if the VAX encoded pixel value is special or not.
double p_lrs_min
The pixel value which is the lower bound of LRS data.
void setMaximum(double max)
Set the output cube attribute maximum.
@ Io
A type of error that occurred when performing an actual I/O operation.
Buffer manager, for moving through a cube in lines.
void ProcessBsq(void funct(Isis::Buffer &out)=NULL)
Process the import data as a band sequential file.
Isis::PixelType p_suffixPixelType
The pixel type of the suffix data.
void OpenFile()
Open the JPEG2000 file.
void setPixelType(PixelType type)
Set the pixel type to that given by the parameter.
void CheckStatus()
Checks and updates the status.
This algorithm is designed for applications that jump around between a couple of spots in the cube wi...
double p_null_max
The pixel value which is the upper bound of NULL data.
int p_dataPreBytes
Number of bytes of non-image data preceding each data record, such as line prefix data in a band sequ...
double p_lis_max
The pixel value which is the upper bound of LIS data.
void SetBase(const double base)
Sets the core base of the input cube.
void ProcessBip(void funct(Isis::Buffer &out)=NULL)
Function to process files stored as Band Interleaved by Pixel.
QString InputFile()
Sets the name of the input file to be read in the import StartProcess method and verifies its existan...
void SetVAXConvert(const bool vax_convert)
Sets the VAX flag of the input cube.
int DataSuffixBytes() const
This method returns the number of data duffix bytes.
std::vector< char * > p_dataHeader
The data header.
uint32_t Uint32_t(void *buf)
Swaps a 32bit unsigned integer.
void SetFileTrailerBytes(const int bytes)
This method sets the number of bytes in the trailer of a file.
bool IsLsb()
Return true if this host is an LSB first machine and false if it is not.
File name manipulation and expansion.
void SetMaximumSteps(const int steps)
This sets the maximum number of steps in the process.
std::vector< std::vector< char * > > DataSuffix()
This method returns a pointer to the data suffix.
void Read(unsigned char **inbuf)
Read data from JP2 file containing 8-bit data.
std::vector< std::vector< char * > > p_dataPost
The data suffix.
@ BIL
Band Interleaved By Line Format (i.e.
char * p_fileHeader
The file header.
QString GetFileName(const QString ¶mName, QString extension="") const
Allows the retrieval of a value for a parameter of type "filename".
bool p_saveFileHeader
Flag indicating whether to save the file header or not.
std::vector< Isis::Cube * > OutputCubes
A vector of pointers to allocated Cube objects.
Base class for all cube processing derivatives.
std::vector< char * > p_dataTrailer
The data trailer.
Container for cube-like labels.
Manipulate and parse attributes of output cube filenames.
double p_lrs_max
The pixel value which is the upper bound of LRS data.
void addObject(const PvlObject &object)
Add a PvlObject.
void SetAttributes(CubeAttributeOutput &att)
Given a CubeAttributeOutput object, set min/max to propagate if propagating min/max attributes was re...
Isis::PixelType p_pixelType
Pixel type of input data.
virtual ~ProcessImport()
Destroys the Import object.
double p_his_max
The pixel value which is the upper bound of HIS data.
double p_hrs_max
The pixel value which is the upper bound of HRS data.
char * p_fileTrailer
The file trailer.
void SaveDataTrailer()
This method marks the data block trailers to be saved.
bool p_saveDataPost
Flag indicating whether to save the data suffix or not.
void SaveDataHeader()
This method marks the data block headers to be saved.
short int ShortInt(void *buf)
Swaps a short integer value.
Buffer for containing a three dimensional section of an image.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
ByteOrder
Tests the current architecture for byte order.
void SaveDataSuffix()
This method marks the data suffix to be saved.
Buffer for reading and writing cube data.
void SaveDataPrefix()
This method marks the data prefix to be saved.
std::vector< char * > DataTrailer()
This method returns a pointer to the data trailer.
ProcessImport::Interleave p_organization
The format of the input file.
std::vector< std::vector< char * > > DataPrefix()
This method returns a pointer to the data prefix.
static UserInterface & GetUserInterface()
Returns the UserInterface object.
void SetDimensions(const int ns, const int nl, const int nb)
Sets the physical size of the input cube.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Isis::Cube * SetOutputCube(const QString ¶meter)
Create the output file.
double p_lis_min
The pixel value which is the lower bound of LIS data.
Isis::ByteOrder p_byteOrder
Byte order of data.
int DataPrefixBytes() const
This method returns the number of data prefix bytes.
double VAXConversion(void *ibuf)
Conversion routine which translates VAX_REAL to IEEE_REAL.
int p_ns
Number of samples.
int p_dataTrailerBytes
Number of bytes of non-image data after the image data of each data block, such as band trailer data ...
int p_suffixData
The number of bytes past the file header bytes where the suffix data bands are stored.
int p_fileHeaderBytes
The number of bytes of non-image data at the beginning of a file.
double p_his_min
The pixel value which is the lower bound of HIS data.
void SetInputFile(const QString &file)
Sets the name of the input file to be read in the import StartProcess method and verifies its existan...
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
Interleave Organization() const
Gets the organization of the input cube.
void SetHIS(const double his_min, const double his_max)
Sets the range that will be considered Isis::Null.
void SetSpecialValues(const double null, const double lrs, const double lis, const double hrs, const double his)
Sets a mapping of input pixel values to output special pixel values.
void SetDataSuffixBytes(const int bytes)
This method sets the number of bytes at the end of each data record of a file.
bool propagateMinimumMaximum() const
Return true if the min/max are to be propagated from an input cube.
int FileTrailerBytes() const
This method returns the number of file trailer bytes.
std::vector< double > p_base
An array containing the core base for each band.
bool p_saveDataHeader
Flag indicating whether to save the data header or not.
IO Handler for Isis Cubes.
void SetPixelType(const Isis::PixelType type)
Sets the pixel type of the input file.
void SaveFileTrailer()
This method marks the file trailer to be saved.
int DataTrailerBytes() const
This method returns the number of data trailer bytes.
void SetOrganization(const ProcessImport::Interleave org)
Sets the organization of the input cube.
virtual void StartProcess()
Process the input file and write it to the output.
Interleave
This enum includes how the document should be read: by BSQ, BIL, BIP, JP2, or InterleaveUndefined.
virtual Isis::Cube * SetOutputCube(const QString ¶meter)
Allocates a user-specified output cube whose size matches the first input cube.
bool p_saveDataTrailer
Flag indicating whether to save the data trailer or not.
void SetNull(const double null_min, const double null_max)
Sets the range that will be considered Isis::Null.
int FileHeaderBytes() const
This method returns the number of file header bytes.
int Int(void *buf)
Swaps a 4 byte integer value.
PixelType
Enumerations for Isis Pixel Types.
double p_null_min
The pixel value which is the lower bound of NULL data.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
bool IsValidPixel(const double d)
Returns if the input pixel is valid.
Command Line and Xml loader, validation, and access.
void SetMultiplier(const double mult)
Sets the core multiplier of the input cube.
void ProcessJp2(void funct(Isis::Buffer &out)=NULL)
Function to process files containing compressed JPEG2000 data (which is always BSQ but is processed a...
QString PixelTypeName(Isis::PixelType pixelType)
Returns string name of PixelType enumeration entered as input parameter.
void SaveFileHeader()
This method marks the file header to be saved.
int DataHeaderBytes() const
This method returns the number of data header bytes.
QString p_inFile
Input file name.
int p_fileTrailerBytes
The number of bytes of non-image data at the end of a file.
void SetByteOrder(const Isis::ByteOrder order)
Sets the byte order of the input file.
double TestPixel(const double pixel)
Tests the pixel.
std::vector< std::vector< char * > > p_dataPre
The data prefix.
Isis::Progress * p_progress
Pointer to a Progress object.
@ BSQ
Band Sequential Format (i.e.
void ProcessBil(void funct(Isis::Buffer &out)=NULL)
Function to process files stored as Band Interleaved by Line.
int p_dataPostBytes
Number of bytes of non-image data following each data record, such as line suffix data in a band sequ...
void SetFileHeaderBytes(const int bytes)
This method sets the number of bytes in the header of a file.
void CheckPixelRange(QString pixelName, double min, double max)
Checks the special pixel range of the given against all other special pixel value ranges,...
@ JP2
Jpeg 2000 Format (always band sequential).
bool p_saveFileTrailer
Flag indicating whether to save the file trailer or not.
char * FileTrailer()
This method returns a pointer to the file trailer.
bool propagatePixelType() const
Return true if the pixel type is to be propagated from an input cube.
bool p_saveDataPre
Flag indicating whether to save the data prefix or not.
double Double(void *buf)
Swaps a double precision value.
int p_dataHeaderBytes
Number of bytes of non-image data after the file header and before the image data of each data block,...
void setMinimum(double min)
Set the output cube attribute minimum.
void SetLRS(const double lrs_min, const double lrs_max)
Sets the range that will be considered Isis::Null.
void SetDataTrailerBytes(const int bytes)
This method sets the number of bytes in the trailer of each datablock of a file.
double p_hrs_min
The pixel value which is the lower bound of HRS data.
This is free and unencumbered software released into the public domain.
@ BIP
Band Interleaved By Pixel Format (i.e.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
void SetHRS(const double hrs_min, const double hrs_max)
Sets the range that will be considered Isis::Null.
char * FileHeader()
This method returns a pointer to the file header.