File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
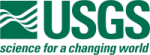 |
Isis 3 Programmer Reference
|
1 #ifndef ProcessMosaic_h
2 #define ProcessMosaic_h
200 static const int FLOAT_STORE_INT_PRECISELY_MAX_VALUE = 16777216;
201 static const int FLOAT_STORE_INT_PRECISELY_MIN_VALUE = -16777215;
212 NumImageOverlayOptions
224 virtual void StartProcess(
const int &piOutSample,
const int &piOutLine,
const int &piOutBand);
237 const int ss,
const int sl,
239 const int ns,
const int nl,
246 const int ss = 1,
const int sl = 1,
248 const int ns = -1,
const int nl = -1,
258 void SetBandBinMatch(
bool enforceBandBinMatch);
260 void SetBandKeyword(QString bandPriorityKeyName, QString bandPriorityKeyValue);
269 void SetTrackFlag(
bool trackingEnabled);
289 int bandPriorityInputBandNumber,
int bandPriorityOutputBandNumber,
294 int ins,
int inl,
int inb,
295 int bandPriorityInputBandNumber,
296 int bandPriorityOutputBandNumber);
324 bool m_trackingEnabled;
326 bool m_createOutputMosaic;
327 int m_bandPriorityBandNumber;
328 QString m_bandPriorityKeyName;
329 QString m_bandPriorityKeyValue;
330 bool m_bandPriorityUseMaxValue;
360 bool m_placeHighSatPixels;
361 bool m_placeLowSatPixels;
362 bool m_placeNullPixels;
int m_oss
The starting sample within the output cube.
void SetBandNumber(int bandPriorityBandNumber)
Set the band to use for priorities when using band priority.
bool GetTrackStatus()
This method searchs the mosaic label for a table with name "InputFile".
Contains Pvl Groups and Pvl Objects.
ImageOverlay
Enumeration for different Mosaic priorities (input, mosaic, band)
int m_osb
The starting band within the output cube.
Buffer for containing a two dimensional section of an image.
void BandComparison(int iss, int isl, int ins, int inl, int bandPriorityInputBandNumber, int bandPriorityOutputBandNumber, int index)
This method compares the specified band of the input and output using the criteria (lesser or greater...
ProcessMosaic()
ProcessMosaic Contructor.
void SetBandUseMaxValue(bool useMax)
Set whether to take the image with the max or min value when using band priority.
void SetHighSaturationFlag(bool placeHighSatPixels)
When true, high saturation (HRS, HIS) will be considered valid data for the purposes of placing pixel...
virtual void EndProcess()
Cleans up by closing input, output and tracking cubes.
void SetMatchDEM(bool matchDEM)
Set the DEM match flag.
@ AverageImageWithMosaic
average priority
void SetNullFlag(bool placeNullPixels)
When true, Null pixels will be considered valid data for the purposes of placing pixels in the output...
@ UseBandPlacementCriteria
band priority
void AddDefaultBandBinGroup()
AddDefaultBandBinGroup.
void AddBandBinGroup(int origIsb)
This method adds the BandBin group to the mosaic corresponding to the actual bands in the mosaic.
Base class for all cube processing derivatives.
void MatchBandBinGroup(int origIsb, int &inb)
This method matches the input BandBin group to the mosaic BandBin Group and allows band to be replace...
int m_iss
The starting sample within the input cube.
virtual void StartProcess(void funct())
In the base class, this method will invoked a user-specified function exactly one time.
int m_isb
The starting band within the input cube.
bool ProcessAveragePriority(int piPixel, Portal &pInPortal, Portal &pOutPortal, Portal &pOrigPortal)
Calculate DN value for a pixel for AverageImageWithMosaic priority and set the Count band portal.
PvlObject m_imagePositions
List of images placed on the mosaic.
void SetCreateFlag(bool createOutputMosaic)
Flag to indicate that the mosaic is being newly created Indication that the new label specific to the...
PvlObject imagePositions()
Accessor for the placed images and their locations.
@ PlaceImagesBeneath
beneath priority
static const char * TRACKING_TABLE_NAME
This is the name of the table in the Cube which will contain the image file names.
virtual Isis::Cube * SetInputCube(const QString ¶meter, const int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
bool m_enforceBandBinMatch
True/False value to determine whether to enforce the input cube bandbin matches the mosaic bandbin gr...
virtual void StartProcess(const int &piOutSample, const int &piOutLine, const int &piOutBand)
This method invokes the process by mosaic operation over a single input cube and single output cube.
void ResetCountBands()
Reset all the count bands to default at the time of mosaic creation.
int m_inl
The number of lines from the input cube.
void MatchDEMShapeModel()
Match the Shape Model for input and mosaic.
Isis::Cube * SetOutputCube(const QString &psParameter)
Opens an output cube specified by the user.
virtual Isis::Cube * SetInputCube(const QString ¶meter, const int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
@ PlaceImagesOnTop
ontop priority
Cube * m_trackingCube
Output tracking cube. NULL unless tracking is enabled.
int m_osl
The starting line within the output cube.
void SetBandKeyword(QString bandPriorityKeyName, QString bandPriorityKeyValue)
Set the keyword/value to use for comparing when using band priority.
static ImageOverlay StringToOverlay(QString)
Convert a QString to an ImageOverlay (case-insensitive).
IO Handler for Isis Cubes.
virtual ~ProcessMosaic()
Destroys the Mosaic object. It will close all opened cubes.
bool GetLowSaturationFlag() const
Command Line and Xml loader, validation, and access.
int GetOriginDefaultByPixelType()
This method returns the defaults(unassigned origin value) depending on the pixel type.
bool m_enforceMatchDEM
DEM of the input and mosaic should match.
void BandPriorityWithNoTracking(int iss, int isl, int isb, int ins, int inl, int inb, int bandPriorityInputBandNumber, int bandPriorityOutputBandNumber)
Mosaicking for Band Priority with no Tracking.
bool GetTrackFlag() const
int GetInputStartLineInMosaic() const
This is the line where the image was placed into the output mosaic.
int GetInputStartSampleInMosaic() const
This is the sample where the image was placed into the output mosaic.
Mosaic two cubes together.
bool GetHighSaturationFlag() const
int m_onb
The number of bands in the output cube.
int m_ins
The number of samples from the input cube.
int GetBandIndex(bool inputFile)
Get the Band Index in an image of type (input/output)
void SetLowSaturationFlag(bool placeLowSatPixels)
When true, low saturation (LRS, LIS) will be considered valid data for the purposes of placing pixels...
static QString OverlayToString(ImageOverlay)
Convert an ImageOverlay to a QString.
int m_inb
The number of bands from the input cube.
int GetInputStartBandInMosaic() const
This is the band where the image was placed into the output mosaic.
This is free and unencumbered software released into the public domain.
int m_isl
The starting line within the input cube.