File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
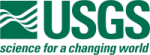 |
Isis 3 Programmer Reference
|
7 #include "PvlFlatMap.h"
12 #include "IException.h"
15 #include "PvlContainer.h"
16 #include "PvlFlatMap.h"
18 #include "PvlKeyword.h"
19 #include "PvlObject.h"
29 PvlConstraints::PvlConstraints() {
38 PvlConstraints::PvlConstraints(
const QString &keyListFile) {
80 PvlConstraints::~PvlConstraints() {
91 int PvlConstraints::excludeSize()
const {
92 return (m_excludes.size());
103 int PvlConstraints::includeSize()
const {
104 return (m_includes.size());
117 int PvlConstraints::keyListSize()
const {
118 return (m_keylist.size());
134 void PvlConstraints::addExclude(
const QString &name) {
135 m_excludes.append(name);
150 void PvlConstraints::addInclude(
const QString &name) {
151 m_includes.append(name);
163 void PvlConstraints::addKeyToList(
const QString &name) {
164 m_keylist.append(name);
203 void PvlConstraints::addKeyToList(
const FileName &fileName) {
204 readKeyListFile(fileName);
219 bool PvlConstraints::isExcluded(
const QString &name)
const {
220 return (m_excludes.contains(name, Qt::CaseInsensitive));
234 bool PvlConstraints::isIncluded(
const QString &name)
const {
235 return (m_includes.contains(name, Qt::CaseInsensitive));
248 bool PvlConstraints::isKeyInList(
const QString &name)
const {
249 return (m_keylist.contains(name, Qt::CaseInsensitive));
304 void PvlConstraints::readKeyListFile(
const FileName &keyListFile) {
332 QString keywordName =
"";
333 while (keyList.
GetLine(keywordName)) {
334 addKeyToList(keywordName);
437 return (contains(key.toLower()));
471 const int index)
const {
489 const QString &value) {
501 insert(key.
name().toLower(), key);
515 const QString &value) {
533 for (
int i = 0; i < key.
size(); i++) {
534 kw.value().addValue(key[i]);
541 for (
int i = 1; i < key.
size(); i++) {
558 return (
bool (remove(key.toLower())));
577 const int &index)
const {
580 QString mess =
"Keyword " + key +
" does not exist!";
583 if (index >= k.value().size()) {
584 QString mess =
"Index " +
toString(index) +
" does not exist for keyword " + key +
"!";
588 return (k.value()[index]);
605 const QString &defValue,
606 const int &index)
const {
608 if (end() == k || index >= k.value().size()) {
612 return (k.value()[index]);
663 QString mess =
"Keyword " + key +
" does not exist!";
683 while ( keys != other.end() ) {
737 bool isExcluded = constraints.
isExcluded(
object.name());
738 bool isIncluded = constraints.
isIncluded(
object.name());
750 else if ( isExcluded ) {
765 PvlObject::ConstPvlObjectIterator objs;
766 for (objs =
object.beginObject() ; objs !=
object.endObject() ; ++objs) {
793 PvlObject::ConstPvlGroupIterator group;
794 for (group =
object.beginGroup() ; group !=
object.endGroup() ; group++) {
836 else if ( isExcluded ) {
880 for (key = pvl.
begin() ; key != pvl.
end() ; ++key) {
889 for (key = pvl.
begin() ; key != pvl.
end() ; ++key) {
int count(const QString &key) const
Returns the number of values associated with a given keyword.
bool isIncluded(const QString &name) const
Determines if a PvlObject or PvlGroup is included.
void add(const QString &key, const QString &value)
Adds PvlKeyword with the given name and value to the PvlFlatMap.
QString name() const
Returns the keyword name.
bool isKeyInList(const QString &name) const
Determines if a PvlKeyword is included.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
QList< PvlKeyword >::const_iterator ConstPvlKeywordIterator
The const keyword iterator.
QString operator()(const QString &name) const
Gets the first value of a keyword in the PvlFlatMap.
bool isNull(const QString &key, const int index=0) const
Determines if the value of a keyword is Null.
Provides a flat map of PvlKeywords.
File name manipulation and expansion.
PvlKeyword keyword(const QString &key) const
Finds a keyword in the PvlFlatMap.
int keyListSize() const
Returns the number of PvlKeywords to include.
bool exists(const QString &key) const
Determines whether a given keyword exists in the PvlFlatMap.
int loadKeywords(const PvlContainer &pvl, const PvlConstraints &constraints)
Loads PvlKeywords within a PvlContainer into the PvlFlatMap.
Provides access to sequential ASCII stream I/O.
bool GetLine(QString &line, const bool skipComments=true)
Gets next line from file.
void addExclude(const QString &name)
Adds a PvlObject/PvlGroup exclusion constraint.
int excludeSize() const
Returns the number of PvlObjects and PvlGroups to exclude.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void append(const PvlKeyword &key)
Appends the given PvlKeyword's values to the PvlFlatMap.
QStringList allValues(const QString &key) const
Gets all the values associated with a keyword in the PvlFlatMap.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
int merge(const PvlFlatMap &other)
Adds the keywords from another PvlFlatMap.
Contains multiple PvlContainers.
int includeSize() const
Returns the number of PvlObjects and PvlGroups to include.
int loadGroups(const PvlObject &object, const PvlConstraints &constraints)
Loads PvlGroups into the PvlFlatMap.
QString get(const QString &key, const int &index=0) const
Gets the value of a keyword in the PvlFlatMap.
void addInclude(const QString &name)
Adds a PvlObject/PvlGroup inclusion constraint.
virtual ~PvlFlatMap()
Destructor.
QString name() const
Returns the container name.
QMap< QString, PvlKeyword >::iterator PvlFlatMapIterator
An iterator for the underlying QMap that PvlFlatMap is built on.
bool isExcluded(const QString &name) const
Determines if a PvlObject or PvlGroup is excluded.
bool erase(const QString &key)
Erases a keyword from the PvlFlatMap.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
static QStringList keywordValues(const PvlKeyword &keyword)
Gets all of a PvlKeyword's values.
int size() const
Returns the number of values stored in this keyword.
This is free and unencumbered software released into the public domain.
PvlKeywordIterator end()
Return the ending iterator.
int loadGroup(const PvlGroup &group, const PvlConstraints &constraints)
Loads a PvlGroup into the PvlFlatMap.
Contains more than one keyword-value pair.
PvlFlatMap()
Default constructor.
int loadObject(const PvlObject &object, const PvlConstraints &constraints)
Loads PvlObjects into the PvlFlatMap.
PvlKeywordIterator begin()
Return the beginning iterator.
This class can be used to define import/export behavior of Pvl structures when used in the PvlFlatMap...
This is free and unencumbered software released into the public domain.