Loading [MathJax]/jax/output/NativeMML/config.js
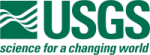 |
Isis 3 Programmer Reference
|
12 #include "SpectralDefinition1D.h"
13 #include "CSVReader.h"
33 QString msg = QObject::tr(
"Input calibration file [%1] must have 2 columns with "
34 "the format: wavelength centers, wavelength widths").
40 QString msg = QObject::tr(
"Input calibration file [%1] must have at least 2 lines.").
51 if (centers[0] < centers[1]) {
58 double lastWavelength = centers[0].toDouble();
61 for (
int i=0; i <csv.
rows(); i++) {
70 Spectel temp(Isis::NULL8, Isis::NULL8, i+1, Isis::NULL8, centers[i].
toDouble(),
72 tempList->append(temp);
73 lastWavelength = centers[i].toDouble();
89 QString msg = QObject::tr(
"Unable to open input file [%1]. Is it a valid CSV?").
102 temp +=
"----Section ";
103 temp +=QString::number(i);
106 temp+=
"Wavelength= ";
107 temp+=QString::number(
m_spectelList->at(i)->at(j).centerWavelength());
109 temp+=QString::number(
m_spectelList->at(i)->at(j).filterWidth());
180 if (tempBand <= m_spectelList->at(i)->length()) {
229 double bestDiff = DBL_MAX;
230 double bestBand = -DBL_MAX;
233 QString msg = QObject::tr(
"Input section number is greater than total number of sections.");
239 if (std::abs(diff) < std::abs(bestDiff)) {
246 if (bestBand == -DBL_MAX) {
bool m_ascendingWavelengths
Do the wavelengths in a given section ascend? Used to determine sections.
CSVAxis getColumn(int index) const
Parse and return a column specified by index order.
Parser::TokenList CSVAxis
Row/Column token list.
int columns() const
Determine the number of columns in the input source.
Stores information about a "Spectral pixel" or spectel.
Reads strings and parses them into tokens separated by a delimiter character.
This is free and unencumbered software released into the public domain.
virtual int sectionCount() const
Returns the number of sections in this Spectral Definition.
File name manipulation and expansion.
@ Unknown
A type of error that cannot be classified as any of the other error types.
int m_numSections
The number of different sections of the Spectral Definition.
double centerWavelength() const
Gets central wavelength of spectel.
int m_nb
Number of bands in input Cube.
virtual ~SpectralDefinition1D()
destructor
Spectel findSpectelByWavelength(double wavelength, int sectionNumber) const
Finds the Spectel with the closest center wavelength (in the given sectionNumber) to the input wavele...
QString toString()
Returns the QString representation of the SpectralDefinition1D.
int sectionNumber(int s, int l, int b) const
Returns the section number that a spectel is in.
int m_nl
Number of lines in input Cube.
SpectralDefinition1D()
construct an empty 1D SpectralDef
QString toString() const
Returns a QString of the full file name including the file path, excluding the attributes with any Is...
const double Null
Value for an Isis Null pixel.
double toDouble(const QString &string)
Global function to convert from a string to a double.
QList< QList< Spectel > * > * m_spectelList
Stores each center wavelength and width.
Spectel findSpectel(const int sample, const int line, const int band) const
Get the Spectel from this SpectralDefinition at a (s,l,b).
int m_ns
Number of samples in input Cube.
This is free and unencumbered software released into the public domain.
int rows() const
Reports the number of rows in the table.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....