Loading [MathJax]/jax/output/NativeMML/config.js
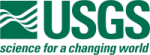 |
Isis 3 Programmer Reference
|
12 #include "DbProfile.h"
16 #include "SqlRecord.h"
76 _throwIfFailed(true) {
91 _throwIfFailed(other._throwIfFailed) { }
112 bool SqlQuery::exec(
const std::string &query) {
115 string mess =
"Query \'" + query +
"\' failed to execute";
151 return (record().count());
199 std::vector<std::string> fields;
201 for(
int i = 0 ; i < rec.count() ; i++) {
216 std::vector<std::string> types;
218 for(
int i = 0 ; i < rec.count() ; i++) {
219 types.push_back(rec.
getType(i).toLatin1().data());
279 string errmess = message +
" - QueryError = " +
std::string fieldName(int index) const
Returns the column name of the resulting query at the given index.
static std::string ToStd(const QString &str)
Converts a Qt string into a std::string.
int fieldIndex(const std::string &name) const
Returns index of column for given name.
std::vector< std::string > fieldNameList() const
Returns the names of all fields in the resulting query.
QString getType(int index) const
Returns the type of a field/column at the specified index.
std::vector< std::string > fieldTypeList() const
Returns the types of each field/column in a resutling query.
bool exec(const std::string &query)
Execute an SQL query provided in the query string.
std::string getQuery() const
Returns the executed query string.
int nFields() const
Returns the number of fields (columns) from query.
void tossQueryError(const std::string &message, const char *f, int l) const
Issues an IException from various sources of error states in this class.
Namespace for the standard library.
Isis database class providing generalized access to a variety of databases.
SqlRecord getRecord() const
Returns a SqlRecord for the current query row.
int nRows() const
Returns the count of rows resulting from the query.
SqlQuery()
Default constructor.
Construct and execute a query on a database and manage result.
This is free and unencumbered software released into the public domain.
QString ToQt() const
Retuns the object string as a QString.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
bool isThrowing() const
Report error status when executing queries.
Provide simplified access to resulting SQL query row.