Loading [MathJax]/jax/output/NativeMML/config.js
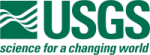 |
Isis 3 Programmer Reference
|
7 #include "SurfaceModel.h"
25 std::vector<double> vec;
34 const double *z,
const int n) {
35 for(
int i = 0; i < n; i++) {
43 const std::vector<double> &y,
44 const std::vector<double> &z) {
45 for(
int i = 0; i < (int)x.size(); i++) {
58 std::vector<double> vec;
91 double det = 4.0 * d * f - e * e;
92 if(det == 0.0)
return 1;
95 x = (c * e - 2.0 * b * f) / det;
96 y = (b * e - 2.0 * c * d) / det;
int Solve(Isis::LeastSquares::SolveMethod method=SVD)
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
void Solve()
Fit a surface to the input triplets.
double Evaluate(const std::vector< double > &input)
Invokes the BasisFunction Evaluate method.
Nth degree Polynomial with two variables.
void AddTriplets(const double *x, const double *y, const double *z, const int n)
Add an array of (x,y,z) triplet to the list of knowns After all knowns are added invoke the Solve met...
void AddTriplet(const double x, const double y, const double z)
Add a single (x,y,z) triplet to the list of knowns. After all knowns are added invoke the Solve metho...
Generic least square fitting class.
SurfaceModel()
Constructor.
double Evaluate(const double x, const double y)
Evaluate at x,y to compute z. This is available after the Solve method is invoked.
~SurfaceModel()
Destructor.
void AddKnown(const std::vector< double > &input, double expected, double weight=1.0)
Invoke this method for each set of knowns.
This is free and unencumbered software released into the public domain.
int MinMax(double &x, double &y)
After invoking Solve, a coordinate (x,y) at a local minimum (or maximum) of the surface model can be ...
double Coefficient(int i) const
Returns the ith coefficient.