File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
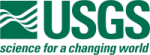 |
Isis 3 Programmer Reference
|
14 #include "TgoCassisDistortionMap.h"
33 QString od =
"INS" +
toString(naifIkCode) +
"_OD_";
35 for(
int i = 0; i < 6; i++) {
QList< double > m_A1_corr
Coefficients for rational distortion model used to compute ideal x from distorted x.
double m_width
The width of the ccd in pixels.
double p_focalPlaneX
Distorted focal plane x.
QList< double > m_A2_dist
Coefficients for rational distortion model used to compute distorted y from ideal y.
double p_undistortedFocalPlaneY
Undistorted focal plane y.
double p_undistortedFocalPlaneX
Undistorted focal plane x.
double m_height
The height of the ccd in pixels.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
double m_pixelPitch
The pixel pitch of the camera.
double chiDotA(double x, double y, QList< double > A)
Evaluate the value for the multi-variate polynomial, given the list of 6 coefficients.
QList< double > m_A1_dist
Coefficients for rational distortion model used to compute distorted x from ideal x.
Camera * p_camera
The camera to distort/undistort.
Distort/undistort focal plane coordinates.
QList< double > m_A3_dist
Coefficients for rational distortion model used to find scaling factor used when computing distorted ...
TgoCassisDistortionMap(Camera *parent, int naifIkCode)
Exomars TGO CaSSIS distortion map constructor.
QList< double > m_A2_corr
Coefficients for rational distortion model used to compute ideal y from distorted y.
virtual bool SetUndistortedFocalPlane(const double ux, const double uy)
Compute distorted focal plane (x,y) given an undistorted focal plane (x,y).
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
virtual bool SetFocalPlane(const double dx, const double dy)
Compute undistorted focal plane (x,y) coordinate given the distorted (x,y).
virtual ~TgoCassisDistortionMap()
Exomars TGO CaSSIS distortion map destructor.
double p_focalPlaneY
Distorted focal plane y.
This is free and unencumbered software released into the public domain.
QList< double > m_A3_corr
Coefficients for rational distortion model used to find scaling factor used when computing ideal coor...