Loading [MathJax]/jax/output/NativeMML/config.js
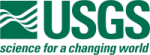 |
Isis 3 Programmer Reference
|
7 #include "TiffExporter.h"
13 #include "IException.h"
51 int mult = (type == Isis::UnsignedByte) ? 1 : 2;
59 "Could not allocate enough memory", _FILEINFO_);
74 QString compression) {
83 "Could not open output image", _FILEINFO_);
88 TIFFSetField(
m_image, TIFFTAG_ROWSPERSTRIP, 1);
89 if (compression ==
"packbits") {
90 TIFFSetField(
m_image, TIFFTAG_COMPRESSION, COMPRESSION_PACKBITS);
92 else if (compression ==
"lzw") {
93 TIFFSetField(
m_image, TIFFTAG_COMPRESSION, COMPRESSION_LZW);
95 else if (compression ==
"deflate") {
96 TIFFSetField(
m_image, TIFFTAG_COMPRESSION, COMPRESSION_ADOBE_DEFLATE);
98 else if (compression ==
"none") {
99 TIFFSetField(
m_image, TIFFTAG_COMPRESSION, COMPRESSION_NONE);
102 QString msg =
"Invalid TIFF compression algorithm: " + compression;
105 TIFFSetField(
m_image, TIFFTAG_PLANARCONFIG, PLANARCONFIG_CONTIG);
106 TIFFSetField(
m_image, TIFFTAG_PHOTOMETRIC,
107 bands() == 1 ? PHOTOMETRIC_MINISBLACK : PHOTOMETRIC_RGB);
110 int bps = (type == Isis::UnsignedByte) ? 8 : 16;
111 TIFFSetField(
m_image, TIFFTAG_BITSPERSAMPLE, bps);
113 TIFFSetField(
m_image, TIFFTAG_SAMPLESPERPIXEL,
bands());
129 int index = s *
bands() + b;
133 m_raster[index] = (
unsigned char) dn;
136 ((
short int *)
m_raster)[index] = (
short int) dn;
139 ((
short unsigned int *)
m_raster)[index] = (
short unsigned int) dn;
143 "Invalid pixel type for data [" +
toString(type) +
"]",
157 "Could not write image", _FILEINFO_);
170 return format ==
"tiff";
File name manipulation and expansion.
@ Unknown
A type of error that cannot be classified as any of the other error types.
TIFF * m_image
Object responsible for writing data to the output image.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
unsigned char * m_raster
Array containing all color channels for a line.
Exports cubes into a standard format in incremental pieces.
virtual void write(FileName outputName, int quality=100, QString compression="none")
Export the Isis cube channels to the given standard image.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
TiffExporter()
Construct the TIFF exporter.
FileName addExtension(const QString &extension) const
Adds a new extension to the file name.
virtual ~TiffExporter()
Destruct the exporter.
QString extension() const
Gets the extension for the output image.
PixelType
Enumerations for Isis Pixel Types.
@ Programmer
This error is for when a programmer made an API call that was illegal.
int lines() const
Number of lines (rows) in the output image.
int bands() const
Number of bands (channels) in the output image.
virtual void writeLine(int l) const
Writes a line of buffered data to the output image on disk.
virtual void setBuffer(int s, int b, int dn) const
Set the DN value at the given sample and band, resolved to a single index, of the line buffer.
PixelType pixelType() const
Returns the pixel type.
int samples() const
Number of samples (columns) in the output image.
static bool canWriteFormat(QString format)
Returns true if the format is "tiff".
virtual void write(FileName outputName, int quality=100, QString compression="none")
Open the output file for writing, initialize its fields, then let the base ImageExporter handle the g...
void setExtension(QString extension)
Sets the extension for the output image and generates the extension for the world file from it.
This is free and unencumbered software released into the public domain.
virtual void createBuffer()
Creates the buffer to store a chunk of streamed line data with one or more bands.