Loading [MathJax]/jax/output/NativeMML/config.js
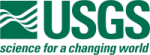 |
Isis 3 Programmer Reference
|
7 #include "ImageExporter.h"
11 #include "CubeAttribute.h"
12 #include "ExportDescription.h"
14 #include "JP2Exporter.h"
15 #include "PixelType.h"
16 #include "ProcessExport.h"
17 #include "QtExporter.h"
18 #include "TiffExporter.h"
34 m_exportDescription = NULL;
38 m_worldExtension =
"";
44 m_outputPixelMinimum = 0.0;
45 m_outputPixelMaximum = 0.0;
59 setExportDescription(desc);
69 delete m_exportDescription;
70 m_exportDescription = NULL;
85 (this->*m_writeMethod)(in);
102 QString compression) {
105 p.ProcessCubes(*
this);
109 createWorldFile(outputName);
152 return m_process->GetInputMinimum(channel);
165 return m_process->GetInputMaximum(channel);
182 m_outputPixelMinimum = outputPixelMinimum;
183 m_outputPixelMaximum = outputPixelMaximum;
194 m_extension = extension;
198 int last = extension.length() - 1;
199 m_worldExtension = extension.mid(0, 1) + extension.mid(last) +
"w";
218 *m_exportDescription = desc;
227 return *m_exportDescription;
247 switch (m_exportDescription->channelCount()) {
259 "Cannot export an image with [" + QString(m_exportDescription->channelCount()) +
265 Cube *cube = addChannel(0);
268 m_bands = m_exportDescription->channelCount();
270 for (
int i = 1; i < m_exportDescription->channelCount(); i++) addChannel(i);
279 m_exportDescription->outputPixelValidMax());
285 setOutputPixelRange(m_exportDescription->outputPixelAbsoluteMin(),
286 m_exportDescription->outputPixelAbsoluteMax());
308 if (m_exportDescription) {
309 return m_exportDescription->pixelType();
326 if (dn < m_outputPixelMinimum) {
327 return m_outputPixelMinimum;
329 else if (dn > m_outputPixelMaximum) {
330 return m_outputPixelMaximum;
393 format = format.toLower();
405 "Cannot export image as format [" + format +
"]",
ProcessExport & process() const
Get a reference to the process object, useful for subclasses to access and manipulate the process.
void setOutputPixelRange(double outputPixelMinimum, double outputPixelMaximum)
Set the DN floor and ceiling for the exported image.
double inputMinimum(int channel) const
Returns the input minimum for the given channel.
Exports cubes into JPEG 2000 images.
Exports cubes into one of several formats with Qt facilities.
File name manipulation and expansion.
Cube * addChannel(int i)
Add a channel of input data to the process from the export description at the given index.
void SetInputRange()
Set input pixel range from user.
Describes how a cube as a single color channel to be exported.
Describes how a series of cubes should be exported.
ExportDescription & exportDescription() const
Gets the description for the output image.
void CreateWorldFile(const QString &worldFile)
Create a standard world file for the input cube.
void setFormat(ExportFormat format)
Sets the storage order of the output file.
virtual void writeGrayscale(vector< Buffer * > &in) const =0
Pure virtual method for writing a line of grayscale data to the output image.
FileName filename() const
Returns a copy of the filename associated with this channel.
CubeAttributeInput attributes() const
Returns a copy of the input attributes associated with this channel.
void SetOutputRange(const double minimum, const double maximum)
Set output pixel range in Buffer.
virtual void EndProcess()
End the processing sequence and cleans up by closing cubes, freeing memory, etc.
void createWorldFile(FileName outputName)
Creates a world file is the input has a map projection.
void SetOutputType(Isis::PixelType pixelIn)
Set output pixel bit type in Buffer.
virtual void writeRgba(vector< Buffer * > &in) const =0
Pure virtual method for writing a line of RGBA data to the output image.
Cube * initializeProcess()
Sets up the export process with the parameters described within the given description.
virtual void write(FileName outputName, int quality=100, QString compression="none")
Export the Isis cube channels to the given standard image.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
FileName addExtension(const QString &extension) const
Adds a new extension to the file name.
virtual ~ImageExporter()
Destruct the exporter.
static ImageExporter * fromFormat(QString format)
A static (factory) method for constructing an ImageExporter instance from an output format.
double inputMaximum(int channel) const
Returns the input maximum for the given channel.
double inputMinimum() const
Returns the input minimum for this channel.
bool hasCustomRange() const
Returns true if the user of this instance has set a custom input range for this channel.
virtual Isis::Cube * SetInputCube(const QString ¶meter, const int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
void operator()(vector< Buffer * > &in) const
The method for writing a line of input image data (with potentially several bands representing color ...
virtual void writeRgb(vector< Buffer * > &in) const =0
Pure virtual method for writing a line of RGB data to the output image.
@ BIL
Band interleaved by line.
IO Handler for Isis Cubes.
virtual int outputPixelValue(double dn) const
Return the output clamped integer pixel value from the input double-precision DN.
QString extension() const
Gets the extension for the output image.
Exports cubes into TIFF images.
ImageExporter()
Construct the exporter.
Export Isis cubes into standard formats.
FileName removeExtension() const
Removes all extensions in the file name.
PixelType
Enumerations for Isis Pixel Types.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
int lines() const
Number of lines (rows) in the output image.
double inputMaximum() const
Returns the input maximum for this channel.
int bands() const
Number of bands (channels) in the output image.
static bool canWriteFormat(QString format)
Returns true if the format is "jp2".
PixelType pixelType() const
Returns the pixel type.
void setExportDescription(ExportDescription &desc)
Sets the description for the output image.
int samples() const
Number of samples (columns) in the output image.
static bool canWriteFormat(QString format)
Returns true if the format is supported by QImageWriter.
static bool canWriteFormat(QString format)
Returns true if the format is "tiff".
void setExtension(QString extension)
Sets the extension for the output image and generates the extension for the world file from it.
void SetOutputNull(const double value)
Set output special pixel value for NULL.
virtual void initialize(ExportDescription &desc)=0
Generic initialization with the export description.
Process class for exporting cubes.
This is free and unencumbered software released into the public domain.