File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
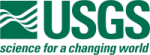 |
Isis 3 Programmer Reference
|
1 #ifndef ProcessExport_h
2 #define ProcessExport_h
15 #include <QCryptographicHash>
19 #include "BufferManager.h"
21 #include "EndianSwapper.h"
22 #include "SpecialPixel.h"
24 #include "UserInterface.h"
127 virtual void StartProcess(
void funct(std::vector<Isis::Buffer *> &in));
140 bool HasInputRange()
const;
143 void SetInputRange(
const double minimum,
const double maximum);
144 void SetInputRange(
const double minimum,
const double maximum,
const int index);
145 void SetInputRange(
const double minimum,
const double middle,
146 const double maximum);
147 void SetInputRange(
const double minimum,
const double middle,
148 const double maximum,
const int index);
175 template <
typename Functor>
void ProcessCubes(
const Functor & functor) {
187 std::vector<BufferManager *> imgrs = GetBuffers();
188 for (
int k = 1; k <= length; k++) {
189 std::vector<Buffer *> ibufs;
191 for (
unsigned int cubeIndex = 0; cubeIndex <
InputCubes.size(); cubeIndex++) {
193 InputCubes[cubeIndex]->read(*imgrs[cubeIndex]);
196 for (
int sampleIndex = 0; sampleIndex < samples; sampleIndex++) {
197 (*imgrs[cubeIndex])[sampleIndex] =
198 p_str[cubeIndex]->Map((*imgrs[cubeIndex])[sampleIndex]);
201 ibufs.push_back(imgrs[cubeIndex]);
207 for (
unsigned int i = 0; i < imgrs.size(); i++) imgrs[i]->next();
217 std::vector<BufferManager *> GetBuffers();
std::vector< Isis::Cube * > InputCubes
A vector of pointers to opened Cube objects.
double p_Lis
The output value for pixels whose input DNs are Low Instrument Saturation values.
void SetOutputLis(const double value)
Set output special pixel value for LIS.
void CheckStatus()
Checks and updates the status.
bool m_canGenerateChecksum
Flag to determine if a file checksum will be generated.
ExportFormat
Storage order enumeration.
ByteOrder p_endianType
The byte order of the output file.
void SetInputRange()
Set input pixel range from user.
void setCanGenerateChecksum(bool flag)
@description Set m_canGenerateChecksum which determines if we can generate a MD5 checksum on the imag...
ExportFormat p_format
Current storage order.
double p_Hrs
The output value for pixels whose input DNs are High Representation Saturation values.
QCryptographicHash * m_cryptographicHash
A cryptographic hash that will generate an MD5 checksum of the image data.
Base class for all cube processing derivatives.
void isisOut8(Buffer &in, std::ofstream &fout)
Method for writing 8-bit unsigned pixel data to a file stream.
void CreateWorldFile(const QString &worldFile)
Create a standard world file for the input cube.
double OutputHrs()
Return the output special pixel value for HRS.
void SetOutputHrs(const double value)
Set output special pixel value for HRS.
std::vector< BufferManager * > GetBuffersBIL()
A single line of input data from each input cube will be passed to the line processing function.
void setFormat(ExportFormat format)
Sets the storage order of the output file.
double OutputLrs()
Return the output special pixel value for LRS.
double p_His
The output value for pixels whose input DNs are High Instrument Saturation values.
std::vector< BufferManager * > GetBuffersBSQ()
A single line of input data from each input cube will be passed to the line processing function.
double OutputHis()
Return the output special pixel value for HIS.
double p_outputMaximum
Desired maximum pixel value in the Buffer.
double OutputNull()
Return the output special pixel value for NULL.
virtual void StartProcess(void funct())
In the base class, this method will invoked a user-specified function exactly one time.
void SetOutputRange(const double minimum, const double maximum)
Set output pixel range in Buffer.
double p_Lrs
The output value for pixels whose input DNs are Low Representation Saturation values.
double p_outputMiddle
Middle pixel value (minimum+maximun)/2.0 in the Buffer.
void isisOut16s(Buffer &in, std::ofstream &fout)
Method for writing 16-bit signed pixel data to a file stream.
void SetOutputHis(const double value)
Set output special pixel value for HIS.
double p_Null
The output value for pixels whose input DNs are Null values.
ByteOrder
Tests the current architecture for byte order.
Buffer for reading and writing cube data.
bool p_Lis_Set
Indicates whether p_Lis has been set (i.e.
void SetOutputType(Isis::PixelType pixelIn)
Set output pixel bit type in Buffer.
std::vector< double > p_inputMinimum
Minimum pixel value in the input cube to be mapped to the minimum value in the Buffer.
double GetInputMaximum(unsigned int n=0) const
Get the valid maximum pixel value for the Nth input cube.
void isisOut32(Buffer &in, std::ofstream &fout)
Method for writing 32-bit signed floating point pixels data to a file stream.
virtual void StartProcess(void funct(Isis::Buffer &in))
This method invokes the process operation over a single input cube.
bool p_Hrs_Set
Indicates whether p_Hrs has been set (i.e.
void isisOut64(Buffer &in, std::ofstream &fout)
Method for writing 64-bit signed double precision floating point pixels data to a file stream.
EndianSwapper * p_endianSwap
Object to swap the endianness of the raw output to either MSB or LSB.
void SetOutputLrs(const double value)
Set output special pixel value for LRS.
double GetOutputMaximum()
Get the valid maximum pixel value to be written to the output file.
void SetOutputEndian(enum ByteOrder endianness)
Set byte endianness of the output cube.
@ BIL
Band interleaved by line.
std::vector< Stretch * > p_str
Stretch object to ensure a reasonable range of pixel values in the output data.
void InitProcess()
Convenience method that checks to make sure the user is only using valid input to the StartProcess me...
@ JP2
Compressed JPEG2000.
bool p_Lrs_Set
Indicates whether p_Lrs has been set (i.e.
QString checksum()
@description Generates a file checksum.
PixelType
Enumerations for Isis Pixel Types.
std::vector< BufferManager * > GetBuffersBIP()
A single band of input data from each input cube will be passed to the band processing function.
bool canGenerateChecksum()
@description Return if we can generate a checksum
Command Line and Xml loader, validation, and access.
double GetOutputMinimum()
Get the valid minimum pixel value to be written to the output file.
double GetInputMinimum(unsigned int n=0) const
Get the valid minimum pixel value for the Nth input cube.
std::vector< double > p_inputMaximum
Maximum pixel value in the input cube to be mapped to the maximum value in the Buffer.
std::vector< double > p_inputMiddle
Middle pixel value in the input cube to be mapped to the (minimum+maximum)/2.0 value in the Buffer.
bool p_His_Set
Indicates whether p_His has been set (i.e.
Isis::Progress * p_progress
Pointer to a Progress object.
void isisOut16u(Buffer &in, std::ofstream &fout)
Method for writing 16-bit unsigned pixel data to a file stream.
@ BIP
Band interleaved by pixel.
void SetOutputNull(const double value)
Set output special pixel value for NULL.
double p_outputMinimum
Desired minimum pixel value in the Buffer.
Process class for exporting cubes.
bool p_Null_Set
Indicates whether p_Null has been set (i.e.
PixelType p_pixelType
The bits per pixel of the output image.
ProcessExport()
Constructs an Export object.
double OutputLis()
Return the output special pixel value for LIS.
This is free and unencumbered software released into the public domain.
virtual ~ProcessExport()
Destructor.