Loading [MathJax]/jax/output/NativeMML/config.js
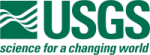 |
Isis 3 Programmer Reference
|
9 #include <QCryptographicHash>
12 #include "ProcessExport.h"
13 #include "Preference.h"
14 #include "IException.h"
15 #include "LineManager.h"
16 #include "BandManager.h"
17 #include "SpecialPixel.h"
18 #include "Histogram.h"
20 #include "Application.h"
21 #include "EndianSwapper.h"
22 #include "Projection.h"
62 for (
unsigned int i = 0; i <
p_str.size(); i++) {
94 double middle = (minimum + maximum) / 2.0;
123 double middle = (minimum + maximum) / 2.0;
154 const double maximum) {
155 if (minimum >= middle) {
157 "minimum must be less than the middle [ProcessExport::SetInputRange]";
160 if (middle >= maximum) {
162 "middle must be less than the maximum [ProcessExport::SetInputRange]";
202 const double maximum,
const int index) {
203 if (minimum >= middle) {
205 "minimum must be less than the middle [ProcessExport::SetInputRange]";
208 if (middle >= maximum) {
210 "middle must be less than the maximum [ProcessExport::SetInputRange]";
213 if (index >= (
int)
InputCubes.size() || index < 0) {
215 "index out of bounds";
368 for (
unsigned int i = 0; i <
InputCubes.size(); i++) {
370 QString strType = ui.
GetString(
"STRETCH");
371 if (strType ==
"MANUAL") {
379 else if (strType !=
"NONE") {
391 if (strType ==
"PIECEWISE") {
412 bool ProcessExport::HasInputRange()
const {
421 "There is no input minimum for channel " +
IString((
int) n),
432 "There is no input maximum for channel " +
IString((
int) n),
455 if (minimum >= maximum) {
457 "minimum must be less than the maximum [ProcessExport::SetOutputRange]";
611 if (p_format < 0 || p_format > 3) {
613 "Format of the output file must be set prior to calling this method [ProcessExport::SetOutputType]";
616 if (pixelIn == Isis::UnsignedByte)
618 else if (pixelIn == Isis::UnsignedWord)
620 else if (pixelIn == Isis::SignedWord)
622 else if (pixelIn == Isis::Real)
625 "Unsupported bit type for JP2 formatted files [ProcessExport::SetOutputType]";
633 "Unsupported bit type [ProcessExport::SetOutputType]";
654 if (byteOrderIn == Isis::NoByteOrder) {
657 else if (byteOrderIn == Isis::Lsb) {
660 else if (byteOrderIn == Isis::Msb) {
699 QString msg =
"Cannot generate a checksum. Call setGenerateChecksum(true) before startProcess";
704 QString msg =
"Unable to generate a checksum. Did you call startProcess?";
728 string m =
"You have not specified any input cubes";
748 for (
unsigned int i = 0; i <
InputCubes.size(); i++) {
804 string m =
"Invalid storage order.";
813 for (
int i = 0; i < buff->
size(); i++) {
814 (*buff)[i] =
p_str[0]->Map((*buff)[i]);
846 vector<BufferManager *> imgrs = GetBuffers();
847 for (
int k = 1; k <= length; k++) {
848 vector<Buffer *> ibufs;
850 for (
unsigned int j = 0; j <
InputCubes.size(); j++) {
855 for (
int i = 0; i <
InputCubes[0]->sampleCount(); i++)
856 (*imgrs[j])[i] =
p_str[j]->Map((*imgrs[j])[i]);
858 ibufs.push_back(imgrs[j]);
864 for (
unsigned int i = 0; i < imgrs.size(); i++) imgrs[i]->next();
870 vector<BufferManager *> ProcessExport::GetBuffers() {
872 vector<BufferManager *> imgrs;
883 string m =
"Invalid storage order.";
909 vector<BufferManager *> imgrs;
910 for (
unsigned int i = 0; i <
InputCubes.size(); i++) {
911 if ((
InputCubes[i]->sampleCount() == samples) &&
916 imgrs.push_back(iline);
919 string m =
"All input cubes must have the same dimensions";
948 vector<BufferManager *> imgrs;
949 for (
unsigned int i = 0; i <
InputCubes.size(); i++) {
950 if ((
InputCubes[i]->sampleCount() == samples) &&
955 imgrs.push_back(iline);
958 string m =
"All input cubes must have the same dimensions";
986 vector<BufferManager *> imgrs;
987 for (
unsigned int i = 0; i <
InputCubes.size(); i++) {
991 imgrs.push_back(iband);
994 string m =
"All input cubes must have the same dimensions";
1031 string m =
"Output stream cannot be generated for requested storage order type.";
1041 QByteArray byteArray;
1043 for (
int i = 0; i < buff->
size(); i++) {
1044 (*buff)[i] =
p_str[0]->Map((*buff)[i]);
1045 byteArray.append((*buff)[i]);
1064 for (
int i = 0; i < buff->
size(); i++) {
1065 (*buff)[i] =
p_str[0]->Map((*buff)[i]);
1100 char *out8 =
new char[in.
size()];
1101 for (
int samp = 0; samp < in.
size(); samp++) {
1102 double pixel = in[samp];
1106 else if (pixel >= 255.0) {
1107 out8[samp] = (char)255;
1110 out8[samp] = (char)(in[samp] + 0.5);
1113 fout.write(out8, in.
size());
1137 short *out16s =
new short[in.
size()];
1138 for (
int samp = 0; samp < in.
size(); samp++) {
1139 double pixel = in[samp];
1141 if (pixel <= -32768.0) {
1142 tempShort = (short)32768;
1143 tempShort = -1 * tempShort;
1145 else if (pixel >= 32767.0) {
1146 tempShort = (short)32767;
1150 if (in[samp] < 0.0) {
1151 tempShort = (short)(in[samp] - 0.5);
1154 tempShort = (short)(in[samp] + 0.5);
1157 void *p_swap = &tempShort;
1160 fout.write((
char *)out16s, in.
size() * 2);
1184 unsigned short *out16u =
new unsigned short[in.
size()];
1185 for (
int samp = 0; samp < in.
size(); samp++) {
1186 double pixel = in[samp];
1187 unsigned short tempShort;
1191 else if (pixel >= 65535.0) {
1195 tempShort = (
unsigned short)(in[samp] + 0.5);
1197 unsigned short *p_swap = &tempShort;
1201 fout.write((
char *)out16u, in.
size() * 2);
1224 int *out32 =
new int[in.
size()];
1225 for (
int samp = 0; samp < in.
size(); samp++) {
1226 double pixel = in[samp];
1228 if (pixel <= -((
double)FLT_MAX)) {
1229 tempFloat = -((double)FLT_MAX);
1231 else if (pixel >= (
double)FLT_MAX) {
1232 tempFloat = (double)FLT_MAX;
1235 tempFloat = (double)in[samp];
1237 void *p_swap = &tempFloat;
1240 fout.write((
char *)out32, in.
size() * 4);
1260 os.open(worldFile.toLatin1().data(), ios::out);
1263 os << std::fixed << setprecision(15)
1270 os << std::fixed << setprecision(15)
1274 os << std::fixed << setprecision(15)
1275 << proj->
XCoord() << endl;
1278 os << std::fixed << setprecision(15)
1279 << proj->
YCoord() << endl;
unsigned short int UnsignedShortInt(void *buf)
Swaps an unsigned short integer value.
std::vector< Isis::Cube * > InputCubes
A vector of pointers to opened Cube objects.
double p_Lis
The output value for pixels whose input DNs are Low Instrument Saturation values.
void SetOutputLis(const double value)
Set output special pixel value for LIS.
Buffer manager, for moving through a cube in lines.
double Percent(const double percent) const
Computes and returns the value at X percent of the histogram.
void CheckStatus()
Checks and updates the status.
double Resolution() const
This method returns the resolution for mapping world coordinates into projection coordinates.
bool next()
Moves the shape buffer to the next position.
bool m_canGenerateChecksum
Flag to determine if a file checksum will be generated.
bool IsLsb()
Return true if this host is an LSB first machine and false if it is not.
void SetMaximumSteps(const int steps)
This sets the maximum number of steps in the process.
ByteOrder p_endianType
The byte order of the output file.
void SetInputRange()
Set input pixel range from user.
void setCanGenerateChecksum(bool flag)
@description Set m_canGenerateChecksum which determines if we can generate a MD5 checksum on the imag...
ExportFormat p_format
Current storage order.
double p_Hrs
The output value for pixels whose input DNs are High Representation Saturation values.
QCryptographicHash * m_cryptographicHash
A cryptographic hash that will generate an MD5 checksum of the image data.
double GetDouble(const QString ¶mName) const
Allows the retrieval of a value for a parameter of type "double".
Base class for all cube processing derivatives.
void isisOut8(Buffer &in, std::ofstream &fout)
Method for writing 8-bit unsigned pixel data to a file stream.
void CreateWorldFile(const QString &worldFile)
Create a standard world file for the input cube.
double OutputHrs()
Return the output special pixel value for HRS.
void SetOutputHrs(const double value)
Set output special pixel value for HRS.
std::vector< BufferManager * > GetBuffersBIL()
A single line of input data from each input cube will be passed to the line processing function.
void setFormat(ExportFormat format)
Sets the storage order of the output file.
Buffer manager, for moving through a cube in bands.
double OutputLrs()
Return the output special pixel value for LRS.
double p_His
The output value for pixels whose input DNs are High Instrument Saturation values.
std::vector< BufferManager * > GetBuffersBSQ()
A single line of input data from each input cube will be passed to the line processing function.
double OutputHis()
Return the output special pixel value for HIS.
double p_outputMaximum
Desired maximum pixel value in the Buffer.
int ExportFloat(void *buf)
Swaps a floating point value for Exporting.
short int ShortInt(void *buf)
Swaps a short integer value.
double OutputNull()
Return the output special pixel value for NULL.
void SetOutputRange(const double minimum, const double maximum)
Set output pixel range in Buffer.
double p_Lrs
The output value for pixels whose input DNs are Low Representation Saturation values.
bool begin()
Moves the shape buffer to the first position.
double p_outputMiddle
Middle pixel value (minimum+maximun)/2.0 in the Buffer.
void isisOut16s(Buffer &in, std::ofstream &fout)
Method for writing 16-bit signed pixel data to a file stream.
void SetOutputHis(const double value)
Set output special pixel value for HIS.
double p_Null
The output value for pixels whose input DNs are Null values.
Manages a Buffer over a cube.
ByteOrder
Tests the current architecture for byte order.
Buffer for reading and writing cube data.
bool end() const
Returns true if the shape buffer has accessed the end of the cube.
bool p_Lis_Set
Indicates whether p_Lis has been set (i.e.
void SetOutputType(Isis::PixelType pixelIn)
Set output pixel bit type in Buffer.
std::vector< double > p_inputMinimum
Minimum pixel value in the input cube to be mapped to the minimum value in the Buffer.
static UserInterface & GetUserInterface()
Returns the UserInterface object.
double GetInputMaximum(unsigned int n=0) const
Get the valid maximum pixel value for the Nth input cube.
void isisOut32(Buffer &in, std::ofstream &fout)
Method for writing 32-bit signed floating point pixels data to a file stream.
virtual void StartProcess(void funct(Isis::Buffer &in))
This method invokes the process operation over a single input cube.
bool p_Hrs_Set
Indicates whether p_Hrs has been set (i.e.
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
EndianSwapper * p_endianSwap
Object to swap the endianness of the raw output to either MSB or LSB.
void SetOutputLrs(const double value)
Set output special pixel value for LRS.
void SetOutputEndian(enum ByteOrder endianness)
Set byte endianness of the output cube.
@ BIL
Band interleaved by line.
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
std::vector< Stretch * > p_str
Stretch object to ensure a reasonable range of pixel values in the output data.
void InitProcess()
Convenience method that checks to make sure the user is only using valid input to the StartProcess me...
@ JP2
Compressed JPEG2000.
bool p_Lrs_Set
Indicates whether p_Lrs has been set (i.e.
void Clear(const QString ¶mName)
Clears the value(s) in the named parameter.
QString checksum()
@description Generates a file checksum.
PixelType
Enumerations for Isis Pixel Types.
std::vector< BufferManager * > GetBuffersBIP()
A single band of input data from each input cube will be passed to the band processing function.
bool canGenerateChecksum()
@description Return if we can generate a checksum
@ Programmer
This error is for when a programmer made an API call that was illegal.
double Median() const
Returns the median.
Namespace for the standard library.
Container of a cube histogram.
bool IsValidPixel(const double d)
Returns if the input pixel is valid.
Command Line and Xml loader, validation, and access.
QString GetString(const QString ¶mName) const
Allows the retrieval of a value for a parameter of type "string".
double GetInputMinimum(unsigned int n=0) const
Get the valid minimum pixel value for the Nth input cube.
std::vector< double > p_inputMaximum
Maximum pixel value in the input cube to be mapped to the maximum value in the Buffer.
std::vector< double > p_inputMiddle
Middle pixel value in the input cube to be mapped to the (minimum+maximum)/2.0 value in the Buffer.
bool p_His_Set
Indicates whether p_His has been set (i.e.
Isis::Progress * p_progress
Pointer to a Progress object.
void PutDouble(const QString ¶mName, const double &value)
Allows the insertion of a value for a parameter of type "double".
int size() const
Returns the total number of pixels in the shape buffer.
void isisOut16u(Buffer &in, std::ofstream &fout)
Method for writing 16-bit unsigned pixel data to a file stream.
Adds specific functionality to C++ strings.
@ BIP
Band interleaved by pixel.
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
Base class for Map Projections.
void SetOutputNull(const double value)
Set output special pixel value for NULL.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
double p_outputMinimum
Desired minimum pixel value in the Buffer.
bool p_Null_Set
Indicates whether p_Null has been set (i.e.
PixelType p_pixelType
The bits per pixel of the output image.
double OutputLis()
Return the output special pixel value for LIS.
This is free and unencumbered software released into the public domain.
virtual ~ProcessExport()
Destructor.