File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
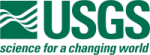 |
Isis 3 Programmer Reference
|
9 #include "BundleObservationSolveSettings.h"
11 #include <QDataStream>
18 #include <QXmlInputSource>
19 #include <QXmlStreamWriter>
21 #include "BundleImage.h"
24 #include "IException.h"
27 #include "PvlKeyword.h"
28 #include "PvlObject.h"
29 #include "XmlStackedHandlerReader.h"
54 xmlReader->setErrorHandler(
new XmlHandler(
this, project));
70 if (scParameterGroup.
hasKeyword(
"CKSOLVEDEGREE")) {
76 QString csolve =
"NONE";
77 csolve = (QString)scParameterGroup.
findKeyword(
"CAMSOLVE");
78 csolve = csolve.toUpper();
79 if (csolve ==
"NONE") {
83 else if (csolve ==
"ANGLES") {
87 else if (csolve ==
"VELOCITIES") {
91 else if (csolve ==
"ACCELERATIONS") {
95 else if (csolve ==
"ALL"){
101 if (scParameterGroup.
hasKeyword(
"OVEREXISTING")) {
102 QString parval = (QString)scParameterGroup.
findKeyword(
"OVEREXISTING");
103 parval = parval.toUpper();
104 if (parval ==
"TRUE" || parval ==
"YES") {
108 else if (parval ==
"FALSE" || parval ==
"NO") {
113 QString msg =
"The OVEREXISTING parameter must be set to TRUE or FALSE; YES or NO";
123 if (scParameterGroup.
hasKeyword(
"SPKSOLVEDEGREE"))
128 QString ssolve =
"NONE";
129 ssolve = (QString)scParameterGroup.
findKeyword(
"SPSOLVE");
130 ssolve = ssolve.toUpper();
131 if (ssolve ==
"NONE") {
135 else if (ssolve ==
"POSITION") {
139 else if (ssolve ==
"VELOCITIES") {
143 else if (ssolve ==
"ACCELERATIONS") {
147 else if (csolve ==
"ALL"){
154 QString parval = (QString)scParameterGroup.
findKeyword(
"TWIST");
155 parval = parval.toUpper();
156 if (parval ==
"TRUE" || parval ==
"YES") {
159 else if (parval ==
"FALSE" || parval ==
"NO") {
163 QString msg =
"The TWIST parameter must be set to TRUE or FALSE; YES or NO";
168 if (scParameterGroup.
hasKeyword(
"OVERHERMITE")) {
169 QString parval = (QString)scParameterGroup.
findKeyword(
"OVERHERMITE");
170 parval = parval.toUpper();
171 if (parval ==
"TRUE" || parval ==
"YES") {
175 else if (parval ==
"FALSE" || parval ==
"NO") {
180 QString msg =
"The OVERHERMITE parameter must be set to TRUE or FALSE; YES or NO";
186 if (scParameterGroup.
hasKeyword(
"CAMERA_ANGLES_SIGMA")) {
192 if (scParameterGroup.
hasKeyword(
"CAMERA_ANGULAR_VELOCITY_SIGMA")){
194 (
double)(scParameterGroup.
findKeyword(
"CAMERA_ANGULAR_VELOCITY_SIGMA")));
199 if (scParameterGroup.
hasKeyword(
"CAMERA_ANGULAR_ACCELERATION_SIGMA")){
201 (
double)(scParameterGroup.
findKeyword(
"CAMERA_ANGULAR_ACCELERATION_SIGMA")));
206 if (scParameterGroup.
hasKeyword(
"ADDITIONAL_CAMERA_POINTING_SIGMAS")) {
207 PvlKeyword additionalSigmas = scParameterGroup.
findKeyword(
"ADDITIONAL_CAMERA_POINTING_SIGMAS");
208 for (
int i = 0; i < additionalSigmas.size(); i++ ) {
215 if (scParameterGroup.
hasKeyword(
"SPACECRAFT_POSITION_SIGMA")) {
221 if (scParameterGroup.
hasKeyword(
"SPACECRAFT_VELOCITY_SIGMA")){
227 if (scParameterGroup.
hasKeyword(
"SPACECRAFT_ACCELERATION_SIGMA")) {
233 if (scParameterGroup.
hasKeyword(
"ADDITIONAL_SPACECRAFT_POSITION_SIGMAS")) {
234 PvlKeyword additionalSigmas = scParameterGroup.
findKeyword(
"ADDITIONAL_SPACECRAFT_POSITION_SIGMAS");
235 for (
int i = 0; i < additionalSigmas.size(); i++ ) {
242 if (scParameterGroup.
hasKeyword(
"CSMSOLVESET")) {
245 else if (scParameterGroup.
hasKeyword(
"CSMSOLVETYPE")) {
248 else if (scParameterGroup.
hasKeyword(
"CSMSOLVELIST")) {
249 PvlKeyword csmSolveListKey = scParameterGroup.
findKeyword(
"CSMSOLVELIST");
251 for (
int i = 0; i < csmSolveListKey.size(); i++) {
252 csmSolveList.append(csmSolveListKey[i]);
267 m_id =
new QUuid(other.
m_id->toString());
316 if (&other !=
this) {
319 m_id =
new QUuid(other.
m_id->toString());
361 m_id =
new QUuid(QUuid::createUuid());
471 if (option.compare(
"NoCSMParameters", Qt::CaseInsensitive) == 0) {
474 else if (option.compare(
"Set", Qt::CaseInsensitive) == 0) {
477 else if (option.compare(
"Type", Qt::CaseInsensitive) == 0) {
480 else if (option.compare(
"List", Qt::CaseInsensitive) == 0) {
485 "Unknown bundle CSM solve option " + option +
".",
500 return "NoCSMParameters";
513 "Unknown CSM solve option enum [" +
toString(option) +
"].",
527 if (set.compare(
"VALID", Qt::CaseInsensitive) == 0) {
528 return csm::param::VALID;
530 else if (set.compare(
"ADJUSTABLE", Qt::CaseInsensitive) == 0) {
531 return csm::param::ADJUSTABLE;
533 else if (set.compare(
"NON_ADJUSTABLE", Qt::CaseInsensitive) == 0) {
534 return csm::param::NON_ADJUSTABLE;
538 "Unknown bundle CSM parameter set " + set +
".",
552 if (set == csm::param::VALID) {
555 else if (set == csm::param::ADJUSTABLE) {
558 else if (set == csm::param::NON_ADJUSTABLE) {
559 return "NON_ADJUSTABLE";
563 "Unknown CSM parameter set enum [" +
toString(set) +
"].",
577 if (type.compare(
"NONE", Qt::CaseInsensitive) == 0) {
578 return csm::param::NONE;
580 else if (type.compare(
"FICTITIOUS", Qt::CaseInsensitive) == 0) {
581 return csm::param::FICTITIOUS;
583 else if (type.compare(
"REAL", Qt::CaseInsensitive) == 0) {
584 return csm::param::REAL;
586 else if (type.compare(
"FIXED", Qt::CaseInsensitive) == 0) {
587 return csm::param::FIXED;
591 "Unknown bundle CSM parameter type " + type +
".",
605 if (type == csm::param::NONE) {
608 else if (type == csm::param::FICTITIOUS) {
611 else if (type == csm::param::REAL) {
614 else if (type == csm::param::FIXED) {
619 "Unknown CSM parameter type enum [" +
toString(type) +
"].",
717 if (option.compare(
"NONE", Qt::CaseInsensitive) == 0) {
720 else if (option.compare(
"NoPointingFactors", Qt::CaseInsensitive) == 0) {
723 else if (option.compare(
"ANGLES", Qt::CaseInsensitive) == 0) {
726 else if (option.compare(
"AnglesOnly", Qt::CaseInsensitive) == 0) {
729 else if (option.compare(
"VELOCITIES", Qt::CaseInsensitive) == 0) {
732 else if (option.compare(
"AnglesAndVelocity", Qt::CaseInsensitive) == 0) {
735 else if (option.compare(
"ACCELERATIONS", Qt::CaseInsensitive) == 0) {
738 else if (option.compare(
"AnglesVelocityAndAcceleration", Qt::CaseInsensitive) == 0) {
741 else if (option.compare(
"ALL", Qt::CaseInsensitive) == 0) {
744 else if (option.compare(
"AllPolynomialCoefficients", Qt::CaseInsensitive) == 0) {
749 "Unknown bundle instrument pointing solve option " + option +
".",
768 else if (option ==
AnglesOnly)
return "AnglesOnly";
773 "Unknown pointing solve option enum [" +
toString(option) +
"].",
796 bool solvePolynomialOverExisting,
797 double anglesAprioriSigma,
798 double angularVelocityAprioriSigma,
799 double angularAccelerationAprioriSigma,
QList<double> * additionalPointingSigmas) {
826 if (anglesAprioriSigma > 0.0) {
834 if (angularVelocityAprioriSigma > 0.0) {
842 if (angularAccelerationAprioriSigma > 0.0) {
852 if (additionalPointingSigmas) {
853 for (
int i=0;i < additionalPointingSigmas->count();i++) {
977 if (option.compare(
"NONE", Qt::CaseInsensitive) == 0) {
980 else if (option.compare(
"NoPositionFactors", Qt::CaseInsensitive) == 0) {
983 else if (option.compare(
"POSITIONS", Qt::CaseInsensitive) == 0) {
986 else if (option.compare(
"PositionOnly", Qt::CaseInsensitive) == 0) {
989 else if (option.compare(
"VELOCITIES", Qt::CaseInsensitive) == 0) {
992 else if (option.compare(
"PositionAndVelocity", Qt::CaseInsensitive) == 0) {
995 else if (option.compare(
"ACCELERATIONS", Qt::CaseInsensitive) == 0) {
998 else if (option.compare(
"PositionVelocityAndAcceleration", Qt::CaseInsensitive) == 0) {
1001 else if (option.compare(
"ALL", Qt::CaseInsensitive) == 0) {
1004 else if (option.compare(
"AllPolynomialCoefficients", Qt::CaseInsensitive) == 0) {
1009 "Unknown bundle instrument position solve option " + option +
".",
1028 else if (option ==
PositionOnly)
return "PositionOnly";
1033 "Unknown position solve option enum [" +
toString(option) +
"].",
1053 bool positionOverHermite,
1054 double positionAprioriSigma,
1055 double velocityAprioriSigma,
1056 double accelerationAprioriSigma,
1082 if (positionAprioriSigma > 0.0) {
1090 if (velocityAprioriSigma > 0.0) {
1098 if (accelerationAprioriSigma > 0.0) {
1108 if (additionalPositionSigmas) {
1109 for (
int i=0;i < additionalPositionSigmas->count();i++) {
1215 const Project *project)
const {
1217 stream.writeStartElement(
"bundleObservationSolveSettings");
1218 stream.writeTextElement(
"id",
m_id->toString());
1219 stream.writeTextElement(
"instrumentId",
instrumentId());
1222 stream.writeStartElement(
"instrumentPointingOptions");
1223 stream.writeAttribute(
"solveOption",
1232 stream.writeStartElement(
"aprioriPointingSigmas");
1235 stream.writeTextElement(
"sigma",
"N/A");
1241 stream.writeEndElement();
1242 stream.writeEndElement();
1245 stream.writeStartElement(
"instrumentPositionOptions");
1246 stream.writeAttribute(
"solveOption",
1254 stream.writeStartElement(
"aprioriPositionSigmas");
1257 stream.writeTextElement(
"sigma",
"N/A");
1263 stream.writeEndElement();
1264 stream.writeEndElement();
1266 stream.writeEndElement();
1282 m_xmlHandlerObservationSettings = settings;
1283 m_xmlHandlerProject = project;
1284 m_xmlHandlerCharacters =
"";
1295 m_xmlHandlerProject = NULL;
1311 const QString &localName,
1312 const QString &qName,
1313 const QXmlAttributes &atts) {
1314 m_xmlHandlerCharacters =
"";
1315 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
1316 if (localName ==
"instrumentPointingOptions") {
1318 QString pointingSolveOption = atts.value(
"solveOption");
1319 if (!pointingSolveOption.isEmpty()) {
1320 m_xmlHandlerObservationSettings->m_instrumentPointingSolveOption
1324 QString numberCoefSolved = atts.value(
"numberCoefSolved");
1325 if (!numberCoefSolved.isEmpty()) {
1326 m_xmlHandlerObservationSettings->m_numberCamAngleCoefSolved =
toInt(numberCoefSolved);
1329 QString
ckDegree = atts.value(
"degree");
1331 m_xmlHandlerObservationSettings->m_ckDegree =
toInt(
ckDegree);
1339 QString
solveTwist = atts.value(
"solveTwist");
1344 QString solveOverExisting = atts.value(
"solveOverExisting");
1345 if (!solveOverExisting.isEmpty()) {
1346 m_xmlHandlerObservationSettings->m_solvePointingPolynomialOverExisting =
1347 toBool(solveOverExisting);
1350 QString interpolationType = atts.value(
"interpolationType");
1351 if (!interpolationType.isEmpty()) {
1352 m_xmlHandlerObservationSettings->m_pointingInterpolationType =
1357 else if (localName ==
"aprioriPointingSigmas") {
1358 m_xmlHandlerAprioriSigmas.clear();
1360 else if (localName ==
"instrumentPositionOptions") {
1362 QString positionSolveOption = atts.value(
"solveOption");
1363 if (!positionSolveOption.isEmpty()) {
1364 m_xmlHandlerObservationSettings->m_instrumentPositionSolveOption
1368 QString numberCoefSolved = atts.value(
"numberCoefSolved");
1369 if (!numberCoefSolved.isEmpty()) {
1370 m_xmlHandlerObservationSettings->m_numberCamPosCoefSolved =
toInt(numberCoefSolved);
1373 QString
spkDegree = atts.value(
"degree");
1383 QString solveOverHermiteSpline = atts.value(
"solveOverHermiteSpline");
1384 if (!solveOverHermiteSpline.isEmpty()) {
1385 m_xmlHandlerObservationSettings->m_solvePositionOverHermiteSpline =
1386 toBool(solveOverHermiteSpline);
1389 QString interpolationType = atts.value(
"interpolationType");
1390 if (!interpolationType.isEmpty()) {
1391 m_xmlHandlerObservationSettings->m_positionInterpolationType =
1395 else if (localName ==
"aprioriPositionSigmas") {
1396 m_xmlHandlerAprioriSigmas.clear();
1412 m_xmlHandlerCharacters += ch;
1413 return XmlStackedHandler::characters(ch);
1428 const QString &localName,
1429 const QString &qName) {
1430 if (!m_xmlHandlerCharacters.isEmpty()) {
1431 if (localName ==
"id") {
1432 m_xmlHandlerObservationSettings->m_id = NULL;
1433 m_xmlHandlerObservationSettings->m_id =
new QUuid(m_xmlHandlerCharacters);
1435 else if (localName ==
"instrumentId") {
1436 m_xmlHandlerObservationSettings->setInstrumentId(m_xmlHandlerCharacters);
1442 else if (localName ==
"sigma") {
1443 m_xmlHandlerAprioriSigmas.append(m_xmlHandlerCharacters);
1445 else if (localName ==
"aprioriPointingSigmas") {
1446 m_xmlHandlerObservationSettings->m_anglesAprioriSigma.clear();
1447 for (
int i = 0; i < m_xmlHandlerAprioriSigmas.size(); i++) {
1448 if (m_xmlHandlerAprioriSigmas[i] ==
"N/A") {
1449 m_xmlHandlerObservationSettings->m_anglesAprioriSigma.append(
Isis::Null);
1452 m_xmlHandlerObservationSettings->m_anglesAprioriSigma.append(
1453 toDouble(m_xmlHandlerAprioriSigmas[i]));
1457 else if (localName ==
"aprioriPositionSigmas") {
1458 m_xmlHandlerObservationSettings->m_positionAprioriSigma.clear();
1459 for (
int i = 0; i < m_xmlHandlerAprioriSigmas.size(); i++) {
1460 if (m_xmlHandlerAprioriSigmas[i] ==
"N/A") {
1461 m_xmlHandlerObservationSettings->m_positionAprioriSigma.append(
Isis::Null);
1464 m_xmlHandlerObservationSettings->m_positionAprioriSigma.append(
1465 toDouble(m_xmlHandlerAprioriSigmas[i]));
1469 m_xmlHandlerCharacters =
"";
1471 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
csm::param::Set m_csmSolveSet
The CSM parameter set to solve for.
static QString csmSolveSetToString(csm::param::Set set)
Convert a CSM parameter set enumeration value to a string.
QStringList m_csmSolveList
The names of the CSM parameters to solve for.
int m_ckDegree
Degree of the polynomial fit to the original camera angles.
@ Type
Solve for all CSM parameters of a specific type.
static QString instrumentPositionSolveOptionToString(InstrumentPositionSolveOption option)
Translates an enumerated InstrumentPositionSolveOption to its string representation.
@ PositionVelocityAcceleration
Solve for instrument positions, velocities, and accelerations.
int m_numberCamAngleCoefSolved
The number of camera angle coefficients in solution.
InstrumentPositionSolveOption instrumentPositionSolveOption() const
Accesses the instrument position solve option.
bool removeObservationNumber(QString observationNumber)
Removes an observation number from this solve settings.
int spkDegree() const
Accesses the degree of the polynomial fit to the original camera position (spkDegree).
@ NoPositionFactors
Solve for none of the position factors.
QList< double > aprioriPointingSigmas() const
Accesses the a priori pointing sigmas.
bool m_solvePositionOverHermiteSpline
The polynomial will be fit over an existing Hermite spline.
@ Set
Solve for all CSM parameters belonging to a specific set.
void setCSMSolveSet(csm::param::Set set)
Set the set of CSM parameters to solve for.
int m_ckSolveDegree
Degree of the camera angles polynomial being fit to in the bundle adjustment.
static csm::param::Set stringToCSMSolveSet(QString set)
Convert a string to its CSM parameter set enumeration value.
Source
The rotation can come from one of 3 places for an Isis cube.
@ Unknown
A type of error that cannot be classified as any of the other error types.
@ AllPointingCoefficients
Solve for all coefficients in the polynomials fit to the pointing angles.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
QStringList csmParameterList() const
Get the list of CSM parameters to solve for.
static CSMSolveOption stringToCSMSolveOption(QString option)
Convert a string to a CSM solve option enumeration value.
XmlHandler(BundleObservationSolveSettings *settings, Project *project)
Constructs an XmlHandler for serialization.
void setCSMSolveParameterList(QStringList list)
Set an explicit list of CSM parameters to solve for.
virtual bool characters(const QString &ch)
csm::param::Type csmParameterType() const
Get the type of CSM parameters to solve for.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
QString m_instrumentId
The spacecraft instrument id for this observation.
int ckDegree() const
Accesses the degree of polynomial fit to original camera angles (ckDegree).
SpicePosition::Source m_positionInterpolationType
SpicePosition interpolation types.
void setInstrumentId(QString instrumentId)
Sets the instrument id for this observation.
@ AnglesOnly
Solve for pointing angles: right ascension, declination and, optionally, twist.
csm::param::Set csmParameterSet() const
Get the set of CSM parameters to solve for.
SpicePosition::Source positionInterpolationType() const
Accesses the SpicePosition interpolation type for the spacecraft position.
void addObservationNumber(QString observationNumber)
Associates an observation number with these solve settings.
@ AnglesVelocity
Solve for pointing angles and their angular velocities.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Manage a stack of content handlers for reading XML files.
bool IsSpecial(const double d)
Returns if the input pixel is special.
The main project for ipce.
@ PolyFunctionOverHermiteConstant
Object is reading from splined.
bool m_solveTwist
Solve for "twist" angle.
static QString instrumentPointingSolveOptionToString(InstrumentPointingSolveOption option)
Tranlsates an enumerated InstrumentPointingSolveOption value to its string representation.
void initialize()
Initializes the default state of this BundleObservationSolveSettings.
bool m_solvePointingPolynomialOverExisting
The polynomial will be fit over the existing pointing polynomial.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
QSet< QString > observationNumbers() const
Returns a list of observation numbers associated with these solve settings.
InstrumentPointingSolveOption instrumentPointingSolveOption() const
Accesses the instrument pointing solve option.
@ PolyFunction
Object is calculated from nth degree polynomial.
int m_spkSolveDegree
Degree of the camera position polynomial being fit to in the bundle adjustment.
Contains multiple PvlContainers.
InstrumentPositionSolveOption m_instrumentPositionSolveOption
Option for how to solve for instrument position.
QList< double > aprioriPositionSigmas() const
Accesses the a priori position sigmas.
InstrumentPointingSolveOption
Options for how to solve for instrument pointing.
@ PositionOnly
Solve for instrument positions only.
QSet< QString > m_observationNumbers
Associated observation numbers for these settings.
int toInt(const QString &string)
Global function to convert from a string to an integer.
BundleObservationSolveSettings()
Constructor with default parameter initializations.
@ AnglesVelocityAcceleration
Solve for pointing angles, their velocities and their accelerations.
int m_spkDegree
Degree of the polynomial fit to the original camera position.
@ NoPointingFactors
Solve for none of the pointing factors.
bool solvePositionOverHermite() const
Whether or not the polynomial for solving will be fit over an existing Hermite spline.
@ PolyFunction
From nth degree polynomial.
~BundleObservationSolveSettings()
Destructor.
int spkSolveDegree() const
Accesses the degree of thecamera position polynomial being fit to in the bundle adjustment (spkSolveD...
Source
This enum indicates the status of the object.
int numberCameraAngleCoefficientsSolved() const
Accesses the number of camera angle coefficients in the solution.
CSMSolveOption csmSolveOption() const
Get how the CSM parameters to solve for are specified for this observation.
static InstrumentPositionSolveOption stringToInstrumentPositionSolveOption(QString option)
Translates a QString InstrumentPositionSolveOption to its enumerated value.
BundleObservationSolveSettings & operator=(const BundleObservationSolveSettings &src)
Assigns the state of another BundleObservationSolveSettings to this one.
void setInstrumentPositionSettings(InstrumentPositionSolveOption option, int spkDegree=2, int spkSolveDegree=2, bool positionOverHermite=false, double positionAprioriSigma=-1.0, double velocityAprioriSigma=-1.0, double accelerationAprioriSigma=-1.0, QList< double > *additionalPositionSigmas=nullptr)
Sets the instrument pointing settings.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
@ AllPositionCoefficients
Solve for all coefficients in the polynomials fit to the instrument positions.
const double Null
Value for an Isis Null pixel.
static InstrumentPointingSolveOption stringToInstrumentPointingSolveOption(QString option)
Translates a QString InstrumentPointingSolveOption to its enumerated value.
void save(QXmlStreamWriter &stream, const Project *project) const
Saves this BundleObservationSolveSettings to an xml stream.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
static QString csmSolveOptionToString(CSMSolveOption option)
Convert a CSM solve option enumeration value to a string.
static csm::param::Type stringToCSMSolveType(QString type)
Convert a string to its CSM parameter type enumeration value.
QList< double > m_positionAprioriSigma
The instrument pointing a priori sigmas.
@ PositionVelocity
Solve for instrument positions and velocities.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
SpiceRotation::Source m_pointingInterpolationType
SpiceRotation interpolation type.
int ckSolveDegree() const
Accesses the degree of the camera angles polynomial being fit to in the bundle adjustment (ckSolveDeg...
CSMSolveOption m_csmSolveOption
How the CSM solution is specified.
void setCSMSolveType(csm::param::Type type)
Set the type of CSM parameters to solve for.
@ List
Solve for an explicit list of CSM parameters.
@ NoCSMParameters
Do not solve for CSM parameters.
QUuid * m_id
A unique ID for this object (useful for others to reference this object when saving to disk).
static QString csmSolveTypeToString(csm::param::Type type)
Convert a CSM parameter type enumeration value to a string.
QList< double > m_anglesAprioriSigma
The image position a priori sigmas.The size of the list is equal to the number of coefficients in the...
This class is used to modify and manage solve settings for 1 to many BundleObservations.
@ PolyFunctionOverSpice
Kernels plus nth degree polynomial.
SpiceRotation::Source pointingInterpolationType() const
Accesses the SpiceRotation interpolation type for the instrument pointing.
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
~XmlHandler()
XmlHandler destructor.
csm::param::Type m_csmSolveType
The CSM parameter type to solve for.
void setInstrumentPointingSettings(InstrumentPointingSolveOption option, bool solveTwist, int ckDegree=2, int ckSolveDegree=2, bool solvePolynomialOverExisting=false, double anglesAprioriSigma=-1.0, double angularVelocityAprioriSigma=-1.0, double angularAccelerationAprioriSigma=-1.0, QList< double > *additionalPointingSigmas=nullptr)
Sets the instrument pointing settings.
InstrumentPointingSolveOption m_instrumentPointingSolveOption
Option for how to solve for instrument pointing.
bool solveTwist() const
Accesses the flag for solving for twist.
QString instrumentId() const
Accesses the instrument id for this observation.
bool solvePolyOverPointing() const
Whether or not the solve polynomial will be fit over the existing pointing polynomial.
int m_numberCamPosCoefSolved
The number of camera position coefficients in the solution.
InstrumentPositionSolveOption
Options for how to solve for instrument position.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
CSMSolveOption
Options for how to solve for CSM parameters.
int numberCameraPositionCoefficientsSolved() const
Accesses the number of camera position coefficients in the solution.