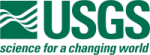 |
Isis 3 Programmer Reference
|
1 #ifndef BundleObservationSolveSettings_h
2 #define BundleObservationSolveSettings_h
15 #include <QStringList>
19 #include "SpicePosition.h"
20 #include "SpiceRotation.h"
21 #include "XmlStackedHandler.h"
25 class QXmlStreamWriter;
31 class XmlStackedHandlerReader;
143 bool solvePolynomialOverExisting =
false,
144 double anglesAprioriSigma = -1.0,
145 double angularVelocityAprioriSigma = -1.0,
146 double angularAccelerationAprioriSigma = -1.0,
175 bool positionOverHermite =
false,
176 double positionAprioriSigma = -1.0,
177 double velocityAprioriSigma = -1.0,
178 double accelerationAprioriSigma = -1.0,
189 void save(QXmlStreamWriter &stream,
const Project *project)
const;
206 virtual bool startElement(
const QString &namespaceURI,
const QString &localName,
207 const QString &qName,
const QXmlAttributes &atts);
209 virtual bool endElement(
const QString &namespaceURI,
const QString &localName,
210 const QString &qName);
217 QString m_xmlHandlerCharacters;
296 #endif // BundleObservationSolveSettings_h
csm::param::Set m_csmSolveSet
The CSM parameter set to solve for.
static QString csmSolveSetToString(csm::param::Set set)
Convert a CSM parameter set enumeration value to a string.
QStringList m_csmSolveList
The names of the CSM parameters to solve for.
int m_ckDegree
Degree of the polynomial fit to the original camera angles.
@ Type
Solve for all CSM parameters of a specific type.
static QString instrumentPositionSolveOptionToString(InstrumentPositionSolveOption option)
Translates an enumerated InstrumentPositionSolveOption to its string representation.
@ PositionVelocityAcceleration
Solve for instrument positions, velocities, and accelerations.
int m_numberCamAngleCoefSolved
The number of camera angle coefficients in solution.
InstrumentPositionSolveOption instrumentPositionSolveOption() const
Accesses the instrument position solve option.
bool removeObservationNumber(QString observationNumber)
Removes an observation number from this solve settings.
int spkDegree() const
Accesses the degree of the polynomial fit to the original camera position (spkDegree).
@ NoPositionFactors
Solve for none of the position factors.
QList< double > aprioriPointingSigmas() const
Accesses the a priori pointing sigmas.
bool m_solvePositionOverHermiteSpline
The polynomial will be fit over an existing Hermite spline.
@ Set
Solve for all CSM parameters belonging to a specific set.
void setCSMSolveSet(csm::param::Set set)
Set the set of CSM parameters to solve for.
int m_ckSolveDegree
Degree of the camera angles polynomial being fit to in the bundle adjustment.
static csm::param::Set stringToCSMSolveSet(QString set)
Convert a string to its CSM parameter set enumeration value.
File name manipulation and expansion.
Source
The rotation can come from one of 3 places for an Isis cube.
@ AllPointingCoefficients
Solve for all coefficients in the polynomials fit to the pointing angles.
QStringList csmParameterList() const
Get the list of CSM parameters to solve for.
static CSMSolveOption stringToCSMSolveOption(QString option)
Convert a string to a CSM solve option enumeration value.
XmlHandler(BundleObservationSolveSettings *settings, Project *project)
Constructs an XmlHandler for serialization.
void setCSMSolveParameterList(QStringList list)
Set an explicit list of CSM parameters to solve for.
virtual bool characters(const QString &ch)
csm::param::Type csmParameterType() const
Get the type of CSM parameters to solve for.
QString m_instrumentId
The spacecraft instrument id for this observation.
int ckDegree() const
Accesses the degree of polynomial fit to original camera angles (ckDegree).
SpicePosition::Source m_positionInterpolationType
SpicePosition interpolation types.
void setInstrumentId(QString instrumentId)
Sets the instrument id for this observation.
@ AnglesOnly
Solve for pointing angles: right ascension, declination and, optionally, twist.
csm::param::Set csmParameterSet() const
Get the set of CSM parameters to solve for.
SpicePosition::Source positionInterpolationType() const
Accesses the SpicePosition interpolation type for the spacecraft position.
void addObservationNumber(QString observationNumber)
Associates an observation number with these solve settings.
@ AnglesVelocity
Solve for pointing angles and their angular velocities.
Manage a stack of content handlers for reading XML files.
The main project for ipce.
QSharedPointer< BundleObservationSolveSettings > BundleObservationSolveSettingsQsp
Definition for BundleObservationSolveSettingsQsp, a QSharedPointer to a BundleObservationSolveSetting...
bool m_solveTwist
Solve for "twist" angle.
static QString instrumentPointingSolveOptionToString(InstrumentPointingSolveOption option)
Tranlsates an enumerated InstrumentPointingSolveOption value to its string representation.
void initialize()
Initializes the default state of this BundleObservationSolveSettings.
bool m_solvePointingPolynomialOverExisting
The polynomial will be fit over the existing pointing polynomial.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
QSet< QString > observationNumbers() const
Returns a list of observation numbers associated with these solve settings.
InstrumentPointingSolveOption instrumentPointingSolveOption() const
Accesses the instrument pointing solve option.
int m_spkSolveDegree
Degree of the camera position polynomial being fit to in the bundle adjustment.
Contains multiple PvlContainers.
InstrumentPositionSolveOption m_instrumentPositionSolveOption
Option for how to solve for instrument position.
QList< double > aprioriPositionSigmas() const
Accesses the a priori position sigmas.
InstrumentPointingSolveOption
Options for how to solve for instrument pointing.
@ PositionOnly
Solve for instrument positions only.
QSet< QString > m_observationNumbers
Associated observation numbers for these settings.
BundleObservationSolveSettings()
Constructor with default parameter initializations.
@ AnglesVelocityAcceleration
Solve for pointing angles, their velocities and their accelerations.
int m_spkDegree
Degree of the polynomial fit to the original camera position.
@ NoPointingFactors
Solve for none of the pointing factors.
bool solvePositionOverHermite() const
Whether or not the polynomial for solving will be fit over an existing Hermite spline.
~BundleObservationSolveSettings()
Destructor.
int spkSolveDegree() const
Accesses the degree of thecamera position polynomial being fit to in the bundle adjustment (spkSolveD...
Source
This enum indicates the status of the object.
int numberCameraAngleCoefficientsSolved() const
Accesses the number of camera angle coefficients in the solution.
CSMSolveOption csmSolveOption() const
Get how the CSM parameters to solve for are specified for this observation.
static InstrumentPositionSolveOption stringToInstrumentPositionSolveOption(QString option)
Translates a QString InstrumentPositionSolveOption to its enumerated value.
BundleObservationSolveSettings & operator=(const BundleObservationSolveSettings &src)
Assigns the state of another BundleObservationSolveSettings to this one.
void setInstrumentPositionSettings(InstrumentPositionSolveOption option, int spkDegree=2, int spkSolveDegree=2, bool positionOverHermite=false, double positionAprioriSigma=-1.0, double velocityAprioriSigma=-1.0, double accelerationAprioriSigma=-1.0, QList< double > *additionalPositionSigmas=nullptr)
Sets the instrument pointing settings.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
@ AllPositionCoefficients
Solve for all coefficients in the polynomials fit to the instrument positions.
static InstrumentPointingSolveOption stringToInstrumentPointingSolveOption(QString option)
Translates a QString InstrumentPointingSolveOption to its enumerated value.
void save(QXmlStreamWriter &stream, const Project *project) const
Saves this BundleObservationSolveSettings to an xml stream.
static QString csmSolveOptionToString(CSMSolveOption option)
Convert a CSM solve option enumeration value to a string.
static csm::param::Type stringToCSMSolveType(QString type)
Convert a string to its CSM parameter type enumeration value.
QList< double > m_positionAprioriSigma
The instrument pointing a priori sigmas.
@ PositionVelocity
Solve for instrument positions and velocities.
SpiceRotation::Source m_pointingInterpolationType
SpiceRotation interpolation type.
int ckSolveDegree() const
Accesses the degree of the camera angles polynomial being fit to in the bundle adjustment (ckSolveDeg...
CSMSolveOption m_csmSolveOption
How the CSM solution is specified.
void setCSMSolveType(csm::param::Type type)
Set the type of CSM parameters to solve for.
XML Handler that parses XMLs in a stack-oriented way.
@ List
Solve for an explicit list of CSM parameters.
@ NoCSMParameters
Do not solve for CSM parameters.
QUuid * m_id
A unique ID for this object (useful for others to reference this object when saving to disk).
static QString csmSolveTypeToString(csm::param::Type type)
Convert a CSM parameter type enumeration value to a string.
QList< double > m_anglesAprioriSigma
The image position a priori sigmas.The size of the list is equal to the number of coefficients in the...
This class is used to modify and manage solve settings for 1 to many BundleObservations.
SpiceRotation::Source pointingInterpolationType() const
Accesses the SpiceRotation interpolation type for the instrument pointing.
~XmlHandler()
XmlHandler destructor.
csm::param::Type m_csmSolveType
The CSM parameter type to solve for.
void setInstrumentPointingSettings(InstrumentPointingSolveOption option, bool solveTwist, int ckDegree=2, int ckSolveDegree=2, bool solvePolynomialOverExisting=false, double anglesAprioriSigma=-1.0, double angularVelocityAprioriSigma=-1.0, double angularAccelerationAprioriSigma=-1.0, QList< double > *additionalPointingSigmas=nullptr)
Sets the instrument pointing settings.
InstrumentPointingSolveOption m_instrumentPointingSolveOption
Option for how to solve for instrument pointing.
bool solveTwist() const
Accesses the flag for solving for twist.
QString instrumentId() const
Accesses the instrument id for this observation.
bool solvePolyOverPointing() const
Whether or not the solve polynomial will be fit over the existing pointing polynomial.
int m_numberCamPosCoefSolved
The number of camera position coefficients in the solution.
InstrumentPositionSolveOption
Options for how to solve for instrument position.
This is free and unencumbered software released into the public domain.
CSMSolveOption
Options for how to solve for CSM parameters.
int numberCameraPositionCoefficientsSolved() const
Accesses the number of camera position coefficients in the solution.