File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
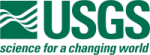 |
Isis 3 Programmer Reference
|
1 #ifndef BundleSettings_h
2 #define BundleSettings_h
15 #include <QSharedPointer>
21 #include "BundleTargetBody.h"
22 #include "BundleObservationSolveSettings.h"
23 #include "MaximumLikelihoodWFunctions.h"
24 #include "SpecialPixel.h"
25 #include "SurfacePoint.h"
26 #include "XmlStackedHandler.h"
30 class QXmlStreamWriter;
165 double multiplier = 1.0);
209 int maximumIterations);
284 void setSCPVLFilename(QString SCParamFilename);
288 QString SCPVLFilename()
const;
290 void save(QXmlStreamWriter &stream,
const Project *project)
const;
316 const QString &localName,
317 const QString &qName,
318 const QXmlAttributes &atts);
320 virtual bool endElement(
const QString &namespaceURI,
321 const QString &localName,
322 const QString &qName);
323 bool fatalError(
const QXmlParseException &exception);
330 QString m_xmlHandlerCharacters;
double globalPointCoord1AprioriSigma() const
Retrieves global a priori sigma for 1st coordinate of points for this bundle.
void save(QXmlStreamWriter &stream, const Project *project) const
This method is used to write a BundleSettings object in an XML format.
void setObservationSolveOptions(QList< BundleObservationSolveSettings > obsSolveSettingsList)
Add the list of solve options for each observation.
@ ParameterCorrections
All parameter corrections will be used to determine that the bundle adjustment has converged.
bool solveTargetBody() const
This method is used to determine whether the bundle adjustment will solve for target body.
@ Welsch
Use a Welsch maximum likelihood model.
Contains Pvl Groups and Pvl Objects.
QList< QPair< MaximumLikelihoodWFunctions::Model, double > > maximumLikelihoodEstimatorModels() const
Retrieves the list of maximum likelihood estimator (MLE) models with their corresponding C-Quantiles.
bool errorPropagation() const
This method is used to determine whether this bundle adjustment will perform error propagation.
This is free and unencumbered software released into the public domain.
bool solveMeanRadius() const
This method is used to determine whether the bundle adjustment will solve for target body mean radius...
bool m_createInverseMatrix
Indicates whether to create the inverse matrix file.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle end tags for the BundleSettings serialized XML.
BundleTargetBodyQsp m_bundleTargetBody
A pointer to the target body settings and information.
double globalPointCoord3AprioriSigma() const
Retrieves the global a priori sigma 3rd coordinate of points for this bundle.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
double m_outlierRejectionMultiplier
The multiplier value for outlier rejection.
bool solveRadius() const
This method is used to determine whether this bundle adjustment will solve for radius.
File name manipulation and expansion.
void setBundleTargetBody(BundleTargetBodyQsp bundleTargetBody)
Sets the target body for the bundle adjustment.
Model
The supported maximum likelihood estimation models.
XmlHandler(BundleSettings *bundleSettings, Project *project)
Create an XML Handler (reader) that can populate the BundleSettings class data.
double convergenceCriteriaThreshold() const
Retrieves the convergence threshold to be used to solve the bundle adjustment.
void setCubeList(QString fileName)
BundleSettings::setCubeList.
ConvergenceCriteria convergenceCriteria() const
Retrieves the convergence criteria to be used to solve the bundle adjustment.
QString m_outputFilePrefix
The prefix for all output files.
bool m_solveObservationMode
Indicates whether to solve for observation mode.
BundleTargetBodyQsp bundleTargetBody() const
Retrieves a pointer to target body information for the bundle adjustment.
bool solvePoleRA() const
This method is used to determine whether the bundle adjustment will solve for target body pole right ...
double m_globalPointCoord1AprioriSigma
The global a priori sigma for latitude or X.
BundleSettings & operator=(const BundleSettings &other)
Assignment operator to allow proper copying of the 'other' BundleSettings object to this one.
void setCreateInverseMatrix(bool createMatrix)
Turn the creation of the inverse correlation matrix file on or off.
int numberTargetBodyParameters() const
This method is used to determine whether the bundle adjustment will solve for target body pole positi...
void setConvergenceCriteria(ConvergenceCriteria criteria, double threshold, int maximumIterations)
Set the convergence criteria options for the bundle adjustment.
void addMaximumLikelihoodEstimatorModel(MaximumLikelihoodWFunctions::Model model, double cQuantile)
Add a maximum likelihood estimator (MLE) model to the bundle adjustment.
Manage a stack of content handlers for reading XML files.
bool validateNetwork() const
This method is used to determine whether to validate the network before the bundle adjustment.
This struct is needed to write the m_maximumLikelihood variable as an HDF5 table.
@ Huber
Use a Huber maximum likelihood model.
bool solvePoleDec() const
This method is used to determine whether the bundle adjustment will solve for target body pole declin...
The main project for ipce.
@ Sigma0
The value of sigma0 will be used to determine that the bundle adjustment has converged.
QList< QPair< MaximumLikelihoodWFunctions::Model, double > > m_maximumLikelihood
Model and C-Quantile for each of the three maximum likelihood estimations.
bool m_validateNetwork
Indicates whether the network should be validated.
unsigned int indexFieldValue
The index of the TableRecord.???
virtual bool characters(const QString &ch)
Add a character from an XML element to the content handler.
MaximumLikelihoodModel
This enum defines the options for maximum likelihood estimation.
void setSolveOptions(bool solveObservationMode=false, bool updateCubeLabel=false, bool errorPropagation=false, bool solveRadius=false, SurfacePoint::CoordinateType coordTypeBundle=SurfacePoint::Latitudinal, SurfacePoint::CoordinateType coordTypeReports=SurfacePoint::Latitudinal, double globalPointCoord1AprioriSigma=Isis::Null, double globalPointCoord2AprioriSigma=Isis::Null, double globalPointCoord3AprioriSigma=Isis::Null)
Set the solve options for the bundle adjustment.
bool m_solveRadius
Indicates whether to solve for point radii.
static ConvergenceCriteria stringToConvergenceCriteria(QString criteria)
Converts the given string value to a BundleSettings::ConvergenceCriteria enumeration.
double m_globalPointCoord3AprioriSigma
The global a priori sigma for radius or Z.
double quantileFieldValue
The quantile of the TableRecord.???
~XmlHandler()
Destroys BundleSettings::XmlHandler object.
int m_convergenceCriteriaMaximumIterations
Maximum number of iterations before quitting the bundle adjustment if it has not yet converged to the...
CoordinateType
Defines the coordinate typ, units, and coordinate index for some of the output methods.
ConvergenceCriteria
This enum defines the options for the bundle adjustment's convergence.
ConvergenceCriteria m_convergenceCriteria
Enumeration used to indicate what criteria to use to determine bundle adjustment convergence.
~BundleSettings()
Destroys the BundleSettings object.
bool solvePMAcceleration() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
QString outputFilePrefix() const
Retrieve the output file prefix.
bool m_updateCubeLabel
Indicates whether to update cubes.
double outlierRejectionMultiplier() const
Retrieves the outlier rejection multiplier for the bundle adjustment.
QSharedPointer< BundleSettings > BundleSettingsQsp
Definition for a BundleSettingsQsp, a shared pointer to a BundleSettings object.
SurfacePoint::CoordinateType controlPointCoordTypeReports() const
Indicates the control point coordinate type for reports.
bool solvePoleDecVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body pole declin...
BundleSettings()
Constructs a BundleSettings object.
double m_globalPointCoord2AprioriSigma
The global a priori sigma for longitude or Y.
bool m_errorPropagation
Indicates whether to perform error propagation.
int numberSolveSettings() const
Retrieves the number of observation solve settings.
SurfacePoint::CoordinateType m_cpCoordTypeBundle
Indicates the coordinate type used for control points in the bundle adjustment.
void setOutlierRejection(bool outlierRejection, double multiplier=1.0)
Set the outlier rejection options for the bundle adjustment.
Container class for BundleAdjustment settings.
bool fatalError(const QXmlParseException &exception)
Format an error message indicating a problem with BundleSettings.
int convergenceCriteriaMaximumIterations() const
Retrieves the maximum number of iterations allowed to solve the bundle adjustment.
double m_convergenceCriteriaThreshold
Tolerance value corresponding to the selected convergence criteria.
double globalPointCoord2AprioriSigma() const
Retrieves the global a priori sigma for 2nd coordinate of points for this bundle.
void setValidateNetwork(bool validate)
Sets the internal flag to indicate whether to validate the network before the bundle adjustment.
const double Null
Value for an Isis Null pixel.
This class is needed to read/write BundleSettings from/to an XML formateed file.
@ ModifiedHuber
Use a modified Huber maximum likelihood model.
bool createInverseMatrix() const
Indicates if the settings will allow the inverse correlation matrix to be created.
bool updateCubeLabel() const
This method is used to determine whether this bundle adjustment will update the cube labels.
void init()
Set Default vales for a BundleSettings object.
@ NoMaximumLikelihoodEstimator
Do not use a maximum likelihood model.
XML Handler that parses XMLs in a stack-oriented way.
bool solvePMVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
SurfacePoint::CoordinateType m_cpCoordTypeReports
Indicates the coordinate type for outputting control points in reports.
bool m_outlierRejection
Indicates whether to perform automatic outlier detection/rejection.
bool solveTriaxialRadii() const
This method is used to determine whether the bundle adjustment will solve for target body triaxial ra...
This class is used to modify and manage solve settings for 1 to many BundleObservations.
@ Latitudinal
Planetocentric latitudinal (lat/lon/rad) coordinates.
bool solveObservationMode() const
This method is used to determine whether this bundle adjustment will solve for observation mode.
QList< BundleObservationSolveSettings > observationSolveSettings() const
Retrieves solve settings for the observation corresponding to the given index.
QString cubeList() const
BundleSettings::cubeList.
static QString convergenceCriteriaToString(ConvergenceCriteria criteria)
Converts the given BundleSettings::ConvergenceCriteria enumeration to a string.
bool validate(const NaifVertex &v)
Verifies that the given NaifVector or NaifVertex is 3 dimensional.
QString nameFieldValue
The model name of the TableRecord.???
bool outlierRejection() const
This method is used to determine whether outlier rejection will be performed on this bundle adjustmen...
bool solvePoleRAVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body pole right ...
@ Chen
Use a Chen maximum likelihood model.
bool solvePM() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
bool m_solveTargetBody
Indicates whether to solve for target body.
void setOutputFilePrefix(QString outputFilePrefix)
Set the output file prefix for the bundle adjustment.
This is free and unencumbered software released into the public domain.
Q_DECLARE_METATYPE(Isis::PlotWindow *)
We have plot windows as QVariant data types, so here it's enabled.
SurfacePoint::CoordinateType controlPointCoordTypeBundle() const
Indicates the control point coordinate type for the actual bundle adjust.