Loading [MathJax]/jax/output/NativeMML/config.js
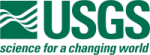 |
Isis 3 Programmer Reference
|
9 #include "BundleSettings.h"
11 #include <QDataStream>
17 #include <QXmlStreamWriter>
18 #include <QXmlInputSource>
20 #include "BundleObservationSolveSettings.h"
21 #include "IException.h"
24 #include "PvlKeyword.h"
25 #include "PvlObject.h"
26 #include "SpecialPixel.h"
27 #include "XmlStackedHandlerReader.h"
42 m_observationSolveSettings.append(defaultSolveSettings);
113 xmlReader->setErrorHandler(
new XmlHandler(
this, project));
125 : m_validateNetwork(other.m_validateNetwork),
126 m_cubeList(other.m_cubeList),
127 m_solveObservationMode(other.m_solveObservationMode),
128 m_solveRadius(other.m_solveRadius),
129 m_updateCubeLabel(other.m_updateCubeLabel),
130 m_errorPropagation(other.m_errorPropagation),
131 m_createInverseMatrix(other.m_createInverseMatrix),
132 m_outlierRejection(other.m_outlierRejection),
133 m_outlierRejectionMultiplier(other.m_outlierRejectionMultiplier),
134 m_globalPointCoord1AprioriSigma(other.m_globalPointCoord1AprioriSigma),
135 m_globalPointCoord2AprioriSigma(other.m_globalPointCoord2AprioriSigma),
136 m_globalPointCoord3AprioriSigma(other.m_globalPointCoord3AprioriSigma),
137 m_observationSolveSettings(other.m_observationSolveSettings),
138 m_convergenceCriteria(other.m_convergenceCriteria),
139 m_convergenceCriteriaThreshold(other.m_convergenceCriteriaThreshold),
140 m_convergenceCriteriaMaximumIterations(other.m_convergenceCriteriaMaximumIterations),
141 m_maximumLikelihood(other.m_maximumLikelihood),
142 m_solveTargetBody(other.m_solveTargetBody),
143 m_bundleTargetBody(other.m_bundleTargetBody),
144 m_cpCoordTypeReports(other.m_cpCoordTypeReports),
145 m_cpCoordTypeBundle(other.m_cpCoordTypeBundle),
146 m_outputFilePrefix(other.m_outputFilePrefix){
170 if (&other !=
this) {
172 m_cubeList = other.m_cubeList;
183 m_observationSolveSettings = other.m_observationSolveSettings;
270 bool updateCubeLabel,
271 bool errorPropagation,
275 double globalPointCoord1AprioriSigma,
276 double globalPointCoord2AprioriSigma,
277 double globalPointCoord3AprioriSigma) {
349 m_observationSolveSettings = obsSolveSettingsList;
518 return m_observationSolveSettings.size();
539 if (m_observationSolveSettings[i].observationNumbers().contains(observationNumber)) {
540 return m_observationSolveSettings[i];
543 return defaultSolveSettings;
562 return m_observationSolveSettings[n];
564 QString msg =
"Unable to find BundleObservationSolveSettings with index = ["
577 return m_observationSolveSettings;
602 if (criteria.compare(
"SIGMA0", Qt::CaseInsensitive) == 0) {
605 else if (criteria.compare(
"PARAMETERCORRECTIONS", Qt::CaseInsensitive) == 0) {
609 "Unknown bundle convergence criteria [" + criteria +
"].",
627 if (criteria ==
Sigma0)
return "Sigma0";
630 "Unknown convergence criteria enum [" +
toString(criteria) +
"].",
647 int maximumIterations) {
728 double maxModelCQuantile) {
731 QString msg =
"For bundle adjustments with multiple maximum likelihood estimators, the first "
732 "model must be of type HUBER or HUBER_MODIFIED.";
1051 stream.writeStartElement(
"bundleSettings");
1053 stream.writeStartElement(
"globalSettings");
1057 stream.writeStartElement(
"solveOptions");
1065 stream.writeEndElement();
1067 stream.writeStartElement(
"aprioriSigmas");
1069 stream.writeAttribute(
"pointCoord1",
"N/A");
1075 stream.writeAttribute(
"pointCoord2",
"N/A");
1081 stream.writeAttribute(
"pointCoord3",
"N/A");
1086 stream.writeEndElement();
1088 stream.writeStartElement(
"outlierRejectionOptions");
1094 stream.writeAttribute(
"multiplier",
"N/A");
1096 stream.writeEndElement();
1098 stream.writeStartElement(
"convergenceCriteriaOptions");
1099 stream.writeAttribute(
"convergenceCriteria",
1101 stream.writeAttribute(
"threshold",
1103 stream.writeAttribute(
"maximumIterations",
1105 stream.writeEndElement();
1107 stream.writeStartElement(
"maximumLikelihoodEstimation");
1109 stream.writeStartElement(
"model");
1110 stream.writeAttribute(
"type",
1113 stream.writeEndElement();
1115 stream.writeEndElement();
1117 stream.writeStartElement(
"outputFileOptions");
1119 stream.writeEndElement();
1121 stream.writeEndElement();
1123 if (!m_observationSolveSettings.isEmpty()) {
1124 stream.writeStartElement(
"observationSolveSettingsList");
1125 for (
int i = 0; i < m_observationSolveSettings.size(); i++) {
1126 m_observationSolveSettings[i].save(stream, project);
1128 stream.writeEndElement();
1133 stream.writeEndElement();
1146 m_xmlHandlerBundleSettings = bundleSettings;
1147 m_xmlHandlerProject = project;
1148 m_xmlHandlerCharacters =
"";
1149 m_xmlHandlerObservationSettings.clear();
1176 const QString &localName,
1177 const QString &qName,
1178 const QXmlAttributes &attributes) {
1179 m_xmlHandlerCharacters =
"";
1181 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, attributes)) {
1183 if (localName ==
"solveOptions") {
1185 QString solveObservationModeStr = attributes.value(
"solveObservationMode");
1186 if (!solveObservationModeStr.isEmpty()) {
1187 m_xmlHandlerBundleSettings->m_solveObservationMode =
toBool(solveObservationModeStr);
1190 QString solveRadiusStr = attributes.value(
"solveRadius");
1191 if (!solveRadiusStr.isEmpty()) {
1192 m_xmlHandlerBundleSettings->m_solveRadius =
toBool(solveRadiusStr);
1195 QString coordTypeReportsStr = attributes.value(
"controlPointCoordinateTypeReports");
1196 if (!coordTypeReportsStr.isEmpty()) {
1197 m_xmlHandlerBundleSettings->m_cpCoordTypeReports =
1201 QString coordTypeBundleStr = attributes.value(
"controlPointCoordinateTypeBundle");
1202 if (!coordTypeBundleStr.isEmpty()) {
1203 m_xmlHandlerBundleSettings->m_cpCoordTypeBundle =
1207 QString updateCubeLabelStr = attributes.value(
"updateCubeLabel");
1208 if (!updateCubeLabelStr.isEmpty()) {
1209 m_xmlHandlerBundleSettings->m_updateCubeLabel =
toBool(updateCubeLabelStr);
1212 QString errorPropagationStr = attributes.value(
"errorPropagation");
1213 if (!errorPropagationStr.isEmpty()) {
1214 m_xmlHandlerBundleSettings->m_errorPropagation =
toBool(errorPropagationStr);
1217 QString createInverseMatrixStr = attributes.value(
"createInverseMatrix");
1218 if (!createInverseMatrixStr.isEmpty()) {
1219 m_xmlHandlerBundleSettings->m_createInverseMatrix =
toBool(createInverseMatrixStr);
1222 else if (localName ==
"aprioriSigmas") {
1224 QString globalPointCoord1AprioriSigmaStr = attributes.value(
"pointCoord1");
1225 m_xmlHandlerBundleSettings->m_globalPointCoord1AprioriSigma =
Isis::Null;
1227 if (!globalPointCoord1AprioriSigmaStr.isEmpty()) {
1228 if (globalPointCoord1AprioriSigmaStr ==
"N/A") {
1229 m_xmlHandlerBundleSettings->m_globalPointCoord1AprioriSigma =
Isis::Null;
1232 m_xmlHandlerBundleSettings->m_globalPointCoord1AprioriSigma
1233 =
toDouble(globalPointCoord1AprioriSigmaStr);
1237 QString globalPointCoord2AprioriSigmaStr = attributes.value(
"pointCoord2");
1238 if (!globalPointCoord2AprioriSigmaStr.isEmpty()) {
1239 if (globalPointCoord2AprioriSigmaStr ==
"N/A") {
1240 m_xmlHandlerBundleSettings->m_globalPointCoord2AprioriSigma =
Isis::Null;
1243 m_xmlHandlerBundleSettings->m_globalPointCoord2AprioriSigma
1244 =
toDouble(globalPointCoord2AprioriSigmaStr);
1248 QString globalPointCoord3AprioriSigmaStr = attributes.value(
"radius");
1249 if (!globalPointCoord3AprioriSigmaStr.isEmpty()) {
1250 if (globalPointCoord3AprioriSigmaStr ==
"N/A") {
1251 m_xmlHandlerBundleSettings->m_globalPointCoord3AprioriSigma =
Isis::Null;
1254 m_xmlHandlerBundleSettings->m_globalPointCoord3AprioriSigma
1255 =
toDouble(globalPointCoord3AprioriSigmaStr);
1259 else if (localName ==
"outlierRejectionOptions") {
1260 QString outlierRejectionStr = attributes.value(
"rejection");
1261 if (!outlierRejectionStr.isEmpty()) {
1262 m_xmlHandlerBundleSettings->m_outlierRejection =
toBool(outlierRejectionStr);
1265 QString outlierRejectionMultiplierStr = attributes.value(
"multiplier");
1266 if (!outlierRejectionMultiplierStr.isEmpty()) {
1267 if (outlierRejectionMultiplierStr !=
"N/A") {
1268 m_xmlHandlerBundleSettings->m_outlierRejectionMultiplier
1269 =
toDouble(outlierRejectionMultiplierStr);
1272 m_xmlHandlerBundleSettings->m_outlierRejectionMultiplier = 3.0;
1276 else if (localName ==
"convergenceCriteriaOptions") {
1278 QString convergenceCriteriaStr = attributes.value(
"convergenceCriteria");
1279 if (!convergenceCriteriaStr.isEmpty()) {
1280 m_xmlHandlerBundleSettings->m_convergenceCriteria
1284 QString convergenceCriteriaThresholdStr = attributes.value(
"threshold");
1285 if (!convergenceCriteriaThresholdStr.isEmpty()) {
1286 m_xmlHandlerBundleSettings->m_convergenceCriteriaThreshold
1287 =
toDouble(convergenceCriteriaThresholdStr);
1290 QString convergenceCriteriaMaximumIterationsStr = attributes.value(
"maximumIterations");
1291 if (!convergenceCriteriaMaximumIterationsStr.isEmpty()) {
1292 m_xmlHandlerBundleSettings->m_convergenceCriteriaMaximumIterations
1293 =
toInt(convergenceCriteriaMaximumIterationsStr);
1296 else if (localName ==
"model") {
1297 QString type = attributes.value(
"type");
1298 QString quantile = attributes.value(
"quantile");
1299 if (!type.isEmpty() && !quantile.isEmpty()) {
1300 m_xmlHandlerBundleSettings->m_maximumLikelihood.append(
1301 qMakePair(MaximumLikelihoodWFunctions::stringToModel(type),
1305 else if (localName ==
"outputFileOptions") {
1306 QString outputFilePrefixStr = attributes.value(
"fileNamePrefix");
1307 if (!outputFilePrefixStr.isEmpty()) {
1308 m_xmlHandlerBundleSettings->m_outputFilePrefix = outputFilePrefixStr;
1311 else if (localName ==
"bundleObservationSolveSettings") {
1312 m_xmlHandlerObservationSettings.append(
1327 m_xmlHandlerCharacters += ch;
1328 return XmlStackedHandler::characters(ch);
1342 const QString &qName) {
1343 if (!m_xmlHandlerCharacters.isEmpty()) {
1344 if (localName ==
"validateNetwork") {
1345 m_xmlHandlerBundleSettings->m_validateNetwork =
toBool(m_xmlHandlerCharacters);
1347 else if (localName ==
"observationSolveSettingsList") {
1348 for (
int i = 0; i < m_xmlHandlerObservationSettings.size(); i++) {
1349 m_xmlHandlerBundleSettings->m_observationSolveSettings.append(
1350 *m_xmlHandlerObservationSettings[i]);
1352 m_xmlHandlerObservationSettings.clear();
1355 m_xmlHandlerCharacters =
"";
1357 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
1368 qDebug() <<
"Parse error with BundleSettings at line " << exception.lineNumber()
1369 <<
", " <<
"column " << exception.columnNumber() <<
": "
1370 << qPrintable(exception.message());
double globalPointCoord1AprioriSigma() const
Retrieves global a priori sigma for 1st coordinate of points for this bundle.
void save(QXmlStreamWriter &stream, const Project *project) const
This method is used to write a BundleSettings object in an XML format.
void setObservationSolveOptions(QList< BundleObservationSolveSettings > obsSolveSettingsList)
Add the list of solve options for each observation.
@ ParameterCorrections
All parameter corrections will be used to determine that the bundle adjustment has converged.
bool solveTargetBody() const
This method is used to determine whether the bundle adjustment will solve for target body.
QList< QPair< MaximumLikelihoodWFunctions::Model, double > > maximumLikelihoodEstimatorModels() const
Retrieves the list of maximum likelihood estimator (MLE) models with their corresponding C-Quantiles.
bool errorPropagation() const
This method is used to determine whether this bundle adjustment will perform error propagation.
This is free and unencumbered software released into the public domain.
bool solveMeanRadius() const
This method is used to determine whether the bundle adjustment will solve for target body mean radius...
bool m_createInverseMatrix
Indicates whether to create the inverse matrix file.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle end tags for the BundleSettings serialized XML.
BundleTargetBodyQsp m_bundleTargetBody
A pointer to the target body settings and information.
double globalPointCoord3AprioriSigma() const
Retrieves the global a priori sigma 3rd coordinate of points for this bundle.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
double m_outlierRejectionMultiplier
The multiplier value for outlier rejection.
bool solveRadius() const
This method is used to determine whether this bundle adjustment will solve for radius.
void setBundleTargetBody(BundleTargetBodyQsp bundleTargetBody)
Sets the target body for the bundle adjustment.
@ Unknown
A type of error that cannot be classified as any of the other error types.
Model
The supported maximum likelihood estimation models.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
XmlHandler(BundleSettings *bundleSettings, Project *project)
Create an XML Handler (reader) that can populate the BundleSettings class data.
double convergenceCriteriaThreshold() const
Retrieves the convergence threshold to be used to solve the bundle adjustment.
void setCubeList(QString fileName)
BundleSettings::setCubeList.
ConvergenceCriteria convergenceCriteria() const
Retrieves the convergence criteria to be used to solve the bundle adjustment.
QString m_outputFilePrefix
The prefix for all output files.
bool m_solveObservationMode
Indicates whether to solve for observation mode.
BundleTargetBodyQsp bundleTargetBody() const
Retrieves a pointer to target body information for the bundle adjustment.
bool solvePoleRA() const
This method is used to determine whether the bundle adjustment will solve for target body pole right ...
double m_globalPointCoord1AprioriSigma
The global a priori sigma for latitude or X.
BundleSettings & operator=(const BundleSettings &other)
Assignment operator to allow proper copying of the 'other' BundleSettings object to this one.
void setCreateInverseMatrix(bool createMatrix)
Turn the creation of the inverse correlation matrix file on or off.
int numberTargetBodyParameters() const
This method is used to determine whether the bundle adjustment will solve for target body pole positi...
void setConvergenceCriteria(ConvergenceCriteria criteria, double threshold, int maximumIterations)
Set the convergence criteria options for the bundle adjustment.
void addMaximumLikelihoodEstimatorModel(MaximumLikelihoodWFunctions::Model model, double cQuantile)
Add a maximum likelihood estimator (MLE) model to the bundle adjustment.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Manage a stack of content handlers for reading XML files.
bool validateNetwork() const
This method is used to determine whether to validate the network before the bundle adjustment.
bool IsSpecial(const double d)
Returns if the input pixel is special.
bool solvePoleDec() const
This method is used to determine whether the bundle adjustment will solve for target body pole declin...
The main project for ipce.
@ Sigma0
The value of sigma0 will be used to determine that the bundle adjustment has converged.
QList< QPair< MaximumLikelihoodWFunctions::Model, double > > m_maximumLikelihood
Model and C-Quantile for each of the three maximum likelihood estimations.
bool m_validateNetwork
Indicates whether the network should be validated.
virtual bool characters(const QString &ch)
Add a character from an XML element to the content handler.
void setSolveOptions(bool solveObservationMode=false, bool updateCubeLabel=false, bool errorPropagation=false, bool solveRadius=false, SurfacePoint::CoordinateType coordTypeBundle=SurfacePoint::Latitudinal, SurfacePoint::CoordinateType coordTypeReports=SurfacePoint::Latitudinal, double globalPointCoord1AprioriSigma=Isis::Null, double globalPointCoord2AprioriSigma=Isis::Null, double globalPointCoord3AprioriSigma=Isis::Null)
Set the solve options for the bundle adjustment.
bool m_solveRadius
Indicates whether to solve for point radii.
static ConvergenceCriteria stringToConvergenceCriteria(QString criteria)
Converts the given string value to a BundleSettings::ConvergenceCriteria enumeration.
double m_globalPointCoord3AprioriSigma
The global a priori sigma for radius or Z.
@ Rectangular
Body-fixed rectangular x/y/z coordinates.
~XmlHandler()
Destroys BundleSettings::XmlHandler object.
int m_convergenceCriteriaMaximumIterations
Maximum number of iterations before quitting the bundle adjustment if it has not yet converged to the...
static QString modelToString(Model model)
Static method to return a string represtentation for a given MaximumLikelihoodWFunctions::Model enum.
CoordinateType
Defines the coordinate typ, units, and coordinate index for some of the output methods.
ConvergenceCriteria
This enum defines the options for the bundle adjustment's convergence.
ConvergenceCriteria m_convergenceCriteria
Enumeration used to indicate what criteria to use to determine bundle adjustment convergence.
~BundleSettings()
Destroys the BundleSettings object.
bool solvePMAcceleration() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
int toInt(const QString &string)
Global function to convert from a string to an integer.
@ HuberModified
A modification to Huber's method propsed by William J.J.
QString outputFilePrefix() const
Retrieve the output file prefix.
bool m_updateCubeLabel
Indicates whether to update cubes.
double outlierRejectionMultiplier() const
Retrieves the outlier rejection multiplier for the bundle adjustment.
SurfacePoint::CoordinateType controlPointCoordTypeReports() const
Indicates the control point coordinate type for reports.
bool solvePoleDecVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body pole declin...
BundleSettings()
Constructs a BundleSettings object.
double m_globalPointCoord2AprioriSigma
The global a priori sigma for longitude or Y.
bool m_errorPropagation
Indicates whether to perform error propagation.
int numberSolveSettings() const
Retrieves the number of observation solve settings.
SurfacePoint::CoordinateType m_cpCoordTypeBundle
Indicates the coordinate type used for control points in the bundle adjustment.
void setOutlierRejection(bool outlierRejection, double multiplier=1.0)
Set the outlier rejection options for the bundle adjustment.
Container class for BundleAdjustment settings.
bool fatalError(const QXmlParseException &exception)
Format an error message indicating a problem with BundleSettings.
int convergenceCriteriaMaximumIterations() const
Retrieves the maximum number of iterations allowed to solve the bundle adjustment.
static CoordinateType stringToCoordinateType(QString type)
This method converts the given string value to a SurfacePoint::CoordinateType enumeration.
double m_convergenceCriteriaThreshold
Tolerance value corresponding to the selected convergence criteria.
double globalPointCoord2AprioriSigma() const
Retrieves the global a priori sigma for 2nd coordinate of points for this bundle.
void setValidateNetwork(bool validate)
Sets the internal flag to indicate whether to validate the network before the bundle adjustment.
const double Null
Value for an Isis Null pixel.
This class is needed to read/write BundleSettings from/to an XML formateed file.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool createInverseMatrix() const
Indicates if the settings will allow the inverse correlation matrix to be created.
bool updateCubeLabel() const
This method is used to determine whether this bundle adjustment will update the cube labels.
void init()
Set Default vales for a BundleSettings object.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
bool solvePMVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
SurfacePoint::CoordinateType m_cpCoordTypeReports
Indicates the coordinate type for outputting control points in reports.
bool m_outlierRejection
Indicates whether to perform automatic outlier detection/rejection.
bool solveTriaxialRadii() const
This method is used to determine whether the bundle adjustment will solve for target body triaxial ra...
This class is used to modify and manage solve settings for 1 to many BundleObservations.
@ Latitudinal
Planetocentric latitudinal (lat/lon/rad) coordinates.
bool solveObservationMode() const
This method is used to determine whether this bundle adjustment will solve for observation mode.
QList< BundleObservationSolveSettings > observationSolveSettings() const
Retrieves solve settings for the observation corresponding to the given index.
QString cubeList() const
BundleSettings::cubeList.
static QString convergenceCriteriaToString(ConvergenceCriteria criteria)
Converts the given BundleSettings::ConvergenceCriteria enumeration to a string.
bool validate(const NaifVertex &v)
Verifies that the given NaifVector or NaifVertex is 3 dimensional.
bool outlierRejection() const
This method is used to determine whether outlier rejection will be performed on this bundle adjustmen...
bool solvePoleRAVelocity() const
This method is used to determine whether the bundle adjustment will solve for target body pole right ...
bool solvePM() const
This method is used to determine whether the bundle adjustment will solve for target body prime merid...
bool m_solveTargetBody
Indicates whether to solve for target body.
void setOutputFilePrefix(QString outputFilePrefix)
Set the output file prefix for the bundle adjustment.
This is free and unencumbered software released into the public domain.
SurfacePoint::CoordinateType controlPointCoordTypeBundle() const
Indicates the control point coordinate type for the actual bundle adjust.