File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
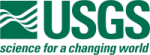 |
Isis 3 Programmer Reference
|
9 #include "MaximumLikelihoodWFunctions.h"
11 #include <QDataStream>
17 #include "IException.h"
57 double tweakingConstant) {
64 : m_model(other.m_model),
65 m_tweakingConstant(other.m_tweakingConstant) {
69 MaximumLikelihoodWFunctions::~MaximumLikelihoodWFunctions() {
73 MaximumLikelihoodWFunctions &MaximumLikelihoodWFunctions::operator=(
74 const MaximumLikelihoodWFunctions &other) {
159 IString msg =
"Maximum likelihood estimation tweaking constants must be > 0.0";
192 return this->
huber(residualZScore);
196 return this->
welsch(residualZScore);
198 return this->
chen(residualZScore);
287 return exp(-(weightFactor)*(weightFactor));
306 - residualZScore * residualZScore;
307 return 6 * weightFactor * weightFactor;
368 if (modelName.compare(
"HUBER", Qt::CaseInsensitive) == 0) {
371 else if (modelName.compare(
"HUBER_MODIFIED", Qt::CaseInsensitive) == 0 ||
372 modelName.compare(
"HUBERMODIFIED", Qt::CaseInsensitive) == 0 ||
373 modelName.compare(
"HUBER MODIFIED", Qt::CaseInsensitive) == 0) {
376 else if (modelName.compare(
"WELSCH", Qt::CaseInsensitive) == 0) {
379 else if (modelName.compare(
"CHEN", Qt::CaseInsensitive) == 0) {
384 "Unknown maximum likelihood model name " + modelName +
".",
419 QDataStream &MaximumLikelihoodWFunctions::write(QDataStream &stream)
const {
427 QDataStream &MaximumLikelihoodWFunctions::read(QDataStream &stream) {
436 QDataStream &
operator<<(QDataStream &stream,
const MaximumLikelihoodWFunctions &mlwf) {
437 return mlwf.write(stream);
442 QDataStream &
operator>>(QDataStream &stream, MaximumLikelihoodWFunctions &mlwf) {
443 return mlwf.read(stream);
449 stream << (qint32)modelEnum;
457 stream >> modelInteger;
const double HALFPI
The mathematical constant PI/2.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
double weightScaler(double residualZScore)
This provides the scalar for the weight (not the scaler for the square root of the weight,...
Model
The supported maximum likelihood estimation models.
void setModel(Model modelSelection)
Allows the maximum likelihood model to be changed together and the default tweaking constant to be se...
double tweakingConstantQuantile()
Suggest a quantile of the probility distribution of the residuals to use as the tweaking constants ba...
void setTweakingConstantDefault()
Sets default tweaking constants based on the maximum likelihood estimation model being used.
double huberModified(double residualZScore)
Modified Huber maximum likelihood estimation function evaluation.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
double chen(double residualZScore)
Modified Huber maximum likelihood estimation function evaluation.
QString weightedResidualCutoff()
Method to return a string represtentation of the weighted residual cutoff (if it exists) for the Maxi...
static QString modelToString(Model model)
Static method to return a string represtentation for a given MaximumLikelihoodWFunctions::Model enum.
@ HuberModified
A modification to Huber's method propsed by William J.J.
MaximumLikelihoodWFunctions()
Sets up a maximumlikelihood estimation function with Huber model and default tweaking constant.
double tweakingConstant() const
Returns the current tweaking constant.
Model model() const
Accessor method to return the MaximumLikelihoodWFunctions::Model enumeration.
double m_tweakingConstant
The tweaking constant for the maximum likelihood models.
@ Programmer
This error is for when a programmer made an API call that was illegal.
@ Welsch
The Welsch method aggresively discounts measures with large resiudals.
double huber(double residualZScore)
Huber maximum likelihood estimation function evaluation.
@ Huber
According to Zhang (Parameter Estimation: A Tutorial with application to conic fitting) "[Huber's] es...
Class provides maximum likelihood estimation functions for robust parameter estimation,...
Adds specific functionality to C++ strings.
double sqrtWeightScaler(double residualZScore)
This provides the scaler to the sqrt of the weight, which is very useful for building normal equation...
Model m_model
The enumerated value for the maximum likelihood estimation model to be used.
std::istream & operator>>(std::istream &is, CSVReader &csv)
Input read operator for input stream sources.
void setTweakingConstant(double tweakingConstant)
Allows the tweaking constant to be changed without changing the maximum likelihood function.
double welsch(double residualZScore)
Modified Huber maximum likelihood estimation function evaluation.
This is free and unencumbered software released into the public domain.
@ Chen
The Chen method was found in "Robust Regression with Projection Based M-estimators" Chen,...